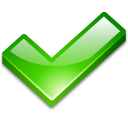
Update 09-2014 : note — this article has been originally created several years ago, so maybe a little different from today’s standards of good and recommended solutions.
Welcome to the next part about practical examples and solutions in JavaScript. We’ll focus mainly on issues of validation (validator elements implementation). It’s just continuation of the previous article.
Best solutions in JavaScript
And let’s start with a simple validator of file types. This is useful for quickly testing the file type, that user wants to send in the form.
Example — file extension validation in JavaScript:
function checkForValidFileExtension(elemVal) { var fp = elemVal; if (fp.indexOf('.') == -1) return false; var allowedExts = new Array("jpg", "jpeg", "pjpeg", "png"); var ext = fp.substring(fp.lastIndexOf('.') + 1). toLowerCase(); for (var i = 0; i < allowedExts.length; i++) { if (ext == allowedExts[i]) return true; } // forbidden return false; }
Example of use (with the Prototype JS library):
Event.observe('user_avatar','change',function() { // value of the "file" field (our file to test) var avv = $("user_avatar").value; var errmsg = "Wrong file type!"; if (checkForValidFileExtension(avv) == true) { $("filemsg").innerHTML = ""; // unlock the ability to send form $('registerSubmit').disabled = false; } else { $("filemsg").innerHTML = errmsg; // block the ability to send form $('registerSubmit').disabled = true; } });
E-mail address validation in JavaScript
The presented version is able to verify one or more e-mail addresses.
Multiple e-mail addresses separated by comma should be given e.g. in the text box. Thus, you can check the whole list of e-mail addresses.
Example — validation of one or more e-mail addresses:
// buffer for incorrect addresses var wrong_buff = ""; function checkEmail() { var input = new Array(); var regx = /^[a-zA-Z0-9_-]+(\.[a-zA-Z0-9_-]+)*@ ([a-zA-Z0-9_-]+)(\.[a-zA-Z0-9_-]+)*(\.[a-zA-Z]{2,4})$/i; var emails = $('email_field').value; // get address (or more if they are comma-separated) input = emails.split(","); var dt = input.length; for(idx = 0; idx < dt; idx++) { var tmp = trim(input[idx]); if (tmp == "") continue; if (tmp.match(regx) == null) { wrong_buff += tmp + ","; } } if (wrong_buff.length < 0) { var lc = wrong_buff.lastIndexOf(","); wrong_buff = wrong_buff.slice(0, lc); return false; } return true; }
Date validation
By arranging a regular expression, we can check correctness of the date.
Example — checking date format in JavaScript:
<p>Date: <input type="text" id="txt1" /><br /> E.g. 10/02/2007 <br /> <input type="button" value="Check" onclick="validate()" /></p>
function isValidDate(sText) { var reDate = /(?:0[1-9]|[12][0-9]|3[01])\/ (?:0[1-9]|1[0-2])\/(?:19|20\d{2})/; return reDate.test(sText); } function validate() { var oInput1 = document.getElementById("txt1"); if (isValidDate(oInput1.value)) { alert("Date is correct"); } else { alert("Wrong format!"); } }
Removing HTML tags from text
Here is a simple, but complete example for removing HTML tags from text data.
<p>Enter the text containing HTML</p> <textarea rows="10" cols="50" id="txt1"></textarea> <input type="button" value="Remove HTML" onclick="showText()" />
String.prototype.stripHTML = function () { var reTag = /<(?:.|\s)*?>/g; return this.replace(reTag, ""); }; function showText() { var oInput1 = document.getElementById("txt1"); var oInput2 = document.getElementById("txt2"); oInput2.value = oInput1.value.stripHTML(); }
Anyone heard of XSS knows how important task it is (particularly dangerous are embedded malicious JavaScripts). And of course it can be done not only on the server side.
Summary
Thus we finished the next part of the series: Best solutions in JavaScript.
The first part was little bit longer, and maybe more interesting. But programming is unfortunately not just doing cool stuff. In any case, we can always meet something, what we do not like too much.
For example I don’t like validation too much, but I know how important is the accuracy of the data and a safety.
In the meantime, we encourage you to follow the subsequent entries.
Thank you.