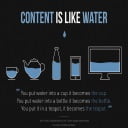
Today a quick tutorial about Bootstrap and creating responsive websites. Actually a template of good start point for further projects. We will also talk about the techniques, which allow us to reach specific results. We also collected links to resources with valuable information.
Bootstrap Tutorial — responsive template by hand
As we know, Twitter Bootstrap (TB) is an open-source CSS framework. Incredibly simplifies and accelerates the creation of solid, nice looking web projects (UI). Mobile-first approach included.
So let’s get straight to the point and create a basic template. We want to get it ready to run in desktop, as well mobile browsers. The first detail will be to define the viewport (area visible for the user at one screen). More about HTML5 basics can be found here.
HTML5 document and including necessary files
Let’s create HTML5 document, and include jQuery, as well Bootstrap 3.3.7 (e.g. from https://www.bootstrapcdn.com).
Simply a skeleton of the page. Example A:
<!DOCTYPE html> <html> <head> <title>Bootstrap</title> <meta charset="utf-8"> <meta name="viewport" content="width=device-width, initial-scale=1"> <meta http-equiv="X-UA-Compatible" content="IE=edge,chrome=1"> <link href="http://fonts.googleapis.com/css?family=Raleway:400,300,600" rel="stylesheet" type="text/css"> <link rel="stylesheet" href="https://maxcdn.bootstrapcdn.com/bootstrap/3.3.7/css/bootstrap.min.css" integrity="sha384-BVYiiSIFeK1dGmJRAkycuHAHRg32OmUcww7on3RYdg4Va+PmSTsz/K68vbdEjh4u" crossorigin="anonymous"> <link rel="stylesheet" href="https://maxcdn.bootstrapcdn.com/bootstrap/3.3.7/css/bootstrap-theme.min.css" integrity="sha384-rHyoN1iRsVXV4nD0JutlnGaslCJuC7uwjduW9SVrLvRYooPp2bWYgmgJQIXwl/Sp" crossorigin="anonymous"> </head> <body> <div class="container"> <h1>Bootstrap starter</h1> <div class="row"> <div class="col-xs-12 col-sm-6 col-md-4 col-lg-3"> A </div> </div> <div class="row"> <div class="col-xs-12 col-sm-6 col-md-4 col-lg-3"> B </div> </div> </div> <script src="https://ajax.googleapis.com/ajax/libs/jquery/1.12.4/jquery.min.js"></script> <script src="https://maxcdn.bootstrapcdn.com/bootstrap/3.3.7/js/bootstrap.min.js" integrity="sha384-Tc5IQib027qvyjSMfHjOMaLkfuWVxZxUPnCJA7l2mCWNIpG9mGCD8wGNIcPD7Txa" crossorigin="anonymous"></script> </body> </html>
A word about the container — rows must be placed inside the element with .container (fixed-width) or .container-fluid (full-width) class, for correct alignment of elements.
Some elements have CSS classes, such as:
class=”col-xs-12 col-sm-6 col-md-4 col-lg-3″
through which (in a short) we define CSS rules for any devices (desktop, tablet, mobile, …). Certainly if we handle only selected cases, e.g.:
class=”col-xs-12 col-sm-6″
then for the other cases there will be the defaults used.
OK, but .col-xs-3, -md, -wtf?
It is in fact very simple, but so useful. Let’s decode what those prefixes mean. In simple words, they are used to define on what sizes of device’s screen those classes should be applied. The Grid system in Bootstrap 3+ defines such four sizes:
xs = extra small screens (mobile phones, max 767px)
sm = small screens (tablets,>= 768px)
md = medium screens (some desktops,>= 992px)
lg = large screens (remaining desktops,>= 1200px)
Usually we should define the classes according to the cases of devices we want to handle.
For example the div element with classes like col-xs-6 and col-sm-4 covers half the screen on the phone (xs), and 1/3 of the screen on the tablet (sm).
Similarly:
<div class="col-xs-6 col-sm-8">Column 2</div>
will cover half the screen on the phone, and 2/3 on the tablet. And so on.
Bootstrap grid is a great “scaffolding” for responsive projects. Certainly worth taking a moment for studying it and checking in practice:
http://getbootstrap.com/css/#grid
Further articles about Bootstrap Grid:
http://www.tutorialrepublic.com/twitter-bootstrap-tutorial/bootstrap-grid-system.php
http://www.wearesicc.com/quick-tips-5-column-layout-with-twitter-bootstrap
Bootstrap offers something extra — the way to define, whether an element has to be visible or hidden on a specific size of the screen. We can handle that simply, using following classes:
.visible-xs
.visible-sm
.visible-md
.visible-lg
and:
.hidden-xs
.hidden-sm
.hidden-md
.hidden-lg
This is the simplest way to control the visible / hidden behavior of elements on different devices.
Example:
<div class="visible-xs-block visible-sm-block"></div>
In this case, the div element will be displayed (block) only on smartphones and tablets, and hidden in case of desktop.
Documentation:
http://getbootstrap.com/css/#responsive-utilities
By the way, it’s good to check the documentation of the components:
http://getbootstrap.com/components
Be more responsive
Time for more complex example. This time something more like a real website, that adjusts the view according to size of the screen. For this we will also add the media queries.
Example B
HTML:
… <body> <div id="container"> <div id="header"> <h1>Page template</h1> </div> <div id="content"> <h2>Content</h2> <p>Lorem ipsum …</p> <p>Foo bar …</p> <p>Lorem ipsum …</p> <p>Foo bar …</p> <br> <p>Thank you!</p> </div> <div id="sidebar"> <h2>Options</h2> <p>Option A</p> <p>Option B</p> <p>Option C</p> <p>Option D</p> </div> <div id="footer"> <h3>Our awesome footer!</h3> </div> </div> </body>
Sample CSS:
html, body { height: 100%; margin: 5px; padding: 5px; font: 100% Arial, sans-serif; } /** Defaults **/ #header { height: 150px; } #content { background: #ffdb19; float: left; padding: 5px 15px; width: 75%; } #sidebar { margin-top: 20px; float: right; width: 20%; } #footer { clear: both; text-align: center; } #header, #sidebar, #footer { border: solid 1px #ccc; padding: 5px 15px; }
Let’s define also media queries, which will adjust the appearance of elements, depending on what screen (device) the page will be opened:
/** Media Queries to handle particular cases **/ /* 980px or less */ @media screen and (max-width: 980px) { #content { width: 60%; } #sidebar { width: 30%; } } /* 700px or less */ @media screen and (max-width: 700px) { #content { width: auto; float: none; } #sidebar { width: auto; float: none; } } /* 480px or less */ @media screen and (max-width: 480px) { #header { height: auto; } h1, h2 { font-size: 22px; } #sidebar { display: none; } }
According to this what we defined, the view will be adjusted automatically on various devices. Samples:
https://www.screencast.com/t/Cw7bS4TTbnl
https://www.screencast.com/t/O9UZCGVrM
https://www.screencast.com/t/j8IqJM88M
https://www.screencast.com/t/fxR4xajsvAxx
Source code (full HTML and CSS of our examples):
http://javascript-html5-tutorial.net/demos/tutorial-bootstrap-start.zip
Resources
– Bootstrap from scratch + examples:
Using Mustache JS by jQuery and Express application example. Part 1/2.
Mustache JS tutorial by jQuery and Express application example. Part 2/2.
– Tutorials:
http://inspirationalpixels.com/tutorials/creating-a-responsive-menu-with-html-css-jquery
https://scotch.io/tag/bootstrap
– Media queries:
http://www.w3schools.com/css/css_rwd_mediaqueries.asp
http://www.moreofless.co.uk/bootstrap-3-media-queries-default-sizes
http://www.vandelaydesign.com/turn-any-site-into-a-responsive-site
https://scotch.io/tutorials/default-sizes-for-twitter-bootstraps-media-queries
– Templates — ready examples of responsive websites, create with Bootstrap:
http://www.w3schools.com/bootstrap/bootstrap_templates.asp
For example:
http://www.w3schools.com/bootstrap/tryit.asp?filename=trybs_temp_blog&stacked=h
– More examples — a good start point for our projects:
http://getbootstrap.com/getting-started/#examples
For example:
http://getbootstrap.com/examples/carousel
http://getbootstrap.com/examples/dashboard
– Various solutions:
http://javascript-html5-tutorial.net/category/bootstrap
See also
Modernizr — supports detection of browser features, as well the work with media queries.
Summary
Mobile first, creating responsive websites, that are looking awesome on various devices… It can be a nice fun! Bootstrap supports this kind of work really well.
We plan next articles about Bootstrap and the mobile-first approach, and for now, we wish you a lot of fun with responsive projects development.
Enjoy!