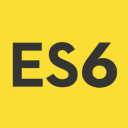
Today… a small article about the approach to writing code. Do you write your JS code declaratively, or rather imperatively? It’s good to take a look closer. Similarly to approaches of writing and organizing CSS styles.
Declarative JavaScript vs imperative in JavaScript/ES6
Declarative programming is a programming paradigm — an approach to building the structure and elements of computer programs, which expresses the logic of calculations without describing its control flow. In contrast to programs written imperatively, the programmer describes the conditions, that the final solution must meet (what we want to achieve), and not the detailed sequence of steps that lead to it (how to do it).
Many languages, that use a declarative approach, tries to minimize or eliminate side effects, describing what the program must do in their field of domains, instead of describing how to achieve this as a sequence of programming language primitives. In turn, in imperative approach we implement algorithms in clear steps.
Boredom. Let’s just look at it.
Imperative code defines what and how has to be done, eg:
– go to the kitchen
– cut the bread
– take the butter out of the fridge
– …
– …
– put a sandwich on the table
In case of declarative we just describe what we need:
– I want a sandwich
Where’s the catch?
Simply, by building our program declaratively, we describe what we need. We compose the program from individual elements. These elements can exist in a framework or library; open-source or in our own libraries. If they are not available, we write them. Imperatively? Yes, in the end, we will come to such a level of abstraction.
Simply, when there is no lower level of abstraction, we need to write a code (functions, libraries, components, etc.) that implements what we need. At a higher level of abstraction, we will use this by building a program declaratively and modifying it more easily.
Boring again, so let’s take a look at JS code samples.
Examples
I = imperative
D = declarative
Example:
// I function makeWidget(options) { const element = document.createElement('div'); element.style.backgroundColor = options.bgColor; element.style.width = options.width; element.style.height = options.height; element.textContent = options.txt; return element; } // D function makeWidget(type, txt) { return new Element(type, txt); }
Instead of writing step by step, we use the “Element” solution, which implements the things we need.
Example — array elements operations:
// I const numbers = [1, 2, 3, 4, 5]; const doubled = []; for (let i = 0; i < numbers.length; i += 1) { const newNumber = numbers[i] * 2; doubled.push(newNumber); } console.log(doubled); // => [2,4,6,8,10] // D const numbersD = [1, 2, 3, 4, 5]; const doubledD = numbersD.map((n) => { return n * 2; }); console.log(doubledD); // => [2,4,6,8,10]
BTW we used here the map method.
And an example below uses the reduce method:
// I const numbers = [1, 2, 3, 4, 5]; let total = 0; for (let i = 0; i < numbers.length; i += 1) { total += numbers[i]; } console.log(total); // => 15 // D const numbers = [1, 2, 3, 4, 5]; const total = numbers.reduce((sum, n) => { return sum + n; }, 0); console.log(total); // => 15
Etc.
In many situations the imperative code is OK; certainly when we write business logic, it will usually be an imperative code, as there is no more generic abstraction for a given case. This code will describe HOW the operation should be performed. And then, at a higher level of abstraction (declarative JavaScript), we save time by defining mainly what to do, using the developed solutions.
Links
https://en.wikipedia.org/wiki/Declarative_programming
https://codeburst.io/declarative-vs-imperative-programming-a8a7c93d9ad2
https://www.netguru.co/blog/imperative-vs-declarative
https://medium.freecodecamp.com/imperative-vs-declarative-programming-283e96bf8aea#.9fmxv73fc
Summary
And summary different than usually.
Attention! Changes are coming. For the better. The blog will appear in a new version in the near future. That means more modern and prettier when it comes to looks, and more “compact” when it comes to posts.
This article is the last of its kind. Although the amount of topics is so huge, however, due to the amount of activities around, there is no time to publish larger articles.
BUT there will be something else — the Tips!
We will change the blog theme to a nicer, responsive and modern one. As it is nowadays. Also the posts — will be short, but published more often.
The old content still will be there, we’ll just focus on short tips; specific solutions of “how to do X”.
Short tips / samples / solutions. And there is a lot of tips and solutions on the list, as we collect them when working with the projects. There are always some problems to solve, and later the solutions may be useful also for the others.
And it’s not only about JS, html5 and CSS, but generally about programming & co (Rails, databases, etc). Having some small solutions for the problems, it’s worth to share with the others 🙂
See you again, later. Thank you && happy coding!!!