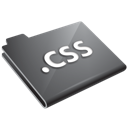
Today another article on the border. It’s about CSS media queries, which are very significant, especially in the context of modern web applications.
CSS media queries
In general, it’s a CSS3 module allowing content rendering to adapt to conditions such as screen resolution. This functionality is actually the core of Responsive Web Design (RWD).
Using media queries
We have to define the @media type and one or more expression concerning properties. If the device will fit into these conditions, then the defined CSS styles will be used.
Example:
@media screen and (min-width: 480px) { border: solid 1px; }
CSS media queries can also be used to attach the whole CSS files.
Examples:
<link rel="stylesheet" type="text/css" href="my_file.css" media="(max-width: 800px)" /> <link rel="stylesheet" type="text/css" href="small_device.css" media="only screen and (max-width: 480px)" />
As you can see they are heavily used:
– min-width and max-width parameters determining the minimum and maximum width of the screen, which satisfies the conditions for use of defined CSS rules.
Logical operators
It is possible to combine the different conditions using logical operators such as not, and, only.
Examples — logical operators in CSS media queries:
/* 1. CSS rules for width = 760px or less */ @media screen and (max-width: 760px) { body { font-weight: none; } div#leftcolumn { display: none; } … } /* 2. CSS rules for width between 600 and 1200px */ @media (min-width: 600px) and (max-width: 1200px) { /* CSS code … */ }
We can easily determine the orientation of the screen:
@media (min-width: 720px) and (orientation: landscape) { … }
and define (separated by commas) the different types of media, for example:
@media screen and (min-width: 1280px), print and (min-resolution: 300dpi) { /* CSS code … */ }
Behavior of comma in this case is similar to an OR operator. This way we can define what what will show up on a small smartphone, iPad or TV screen.
More examples of queries
/* all devices */ @media all { … } /* only to print */ @media print { … } /* surface display HD 1080 or higher */ @media (min-width: 1920px) { … } /* HDTV */ @media (aspect-ratio: 16/9) { … } /* vertical screen */ @media all and (orientation: portrait) { … } /* horizontal */ @media all and (orientation: landscape) { … } /* screens with a height of exactly 1080px */ @media (device-height: 1080px) { … }
So in CSS3 we have a lot of possibilities to determine the types and sizes of screens.
And how to do this in JavaScript? The same — to use media queries!
Matchmedia and using media queries in JavaScript
The related API is window.matchMedia, which allows to test the given criteria.
Example:
var mq = window.matchMedia( "(min-width: 600px)" );
We defined a condition, that subsequently we can check and perform the desired actions in JavaScript code:
if (mq.matches) { alert('The window at least 600px'); } else { alert('Too small …'); }
Please also check the ‘Testing media queries’ page at MDN:
https://developer.mozilla.org/en-US/docs/Web/Guide/CSS/Testing_media_queries
An alternative method is to use the Modernizr library. This library also has functions to perform media queries tests, for example:
Modernizr.mq('only all and (max-width: 600px)')
Details can be found in the documentation:
Resources
As usual, a handful of resources for the curious:
– CSS media queries @ Mozilla:
https://developer.mozilla.org/en-US/docs/Web/Guide/CSS/Media_queries
– use of media queries in practice:
http://css-tricks.com/css-media-queries/
– a website about CSS media queries, including testing tools, examples, and even issues such as Responsive Adsense:
Summary
CSS media queries gaining in importance. Learning how to use them shouldn’t take too much time. And for that, we will have yet another valuable skill.
Have fun with media queries!
Practical analysis — Incidentally , if your company has been searching for a a form , my boss came across a sample form here
http://goo.gl/4ZpOZC