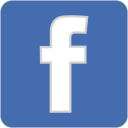
In today’s article I present a small set of issues, which I commonly used when working with the Facebook application development.
Programming Facebook applications
Of course, applications typically have components based on PHP SDK, but in general most of the functionality was created using a dedicated SDK for JavaScript Facebook API.
Programming Facebook Apps is a fairly broad topic in general, and also in the case of individual SDK (PHP, Mobile i of course JavaScript).
Not to mention all the complications and errors that can occur while developing real applications. We can add to that the hours spent at stackoverflow.com, and unfortunately also different requirements, restrictions and changes imposed by Facebook, that application developers simply have to respect.
Sometimes we need to change something in the older applications due to Breaking changes.
Yes it is, but at least the developer is not alone thanks to large communities centered around Facebook Apps Development.
OK, let’s do something practical.
JavaScript Facebook API
The basic step is to load and initialize the Facebook JavaScript SDK, and then — calling the FB.getLoginStatus() method.
Example:
// … FB.getLoginStatus(function(response) { alert('OK'); }); // …
This method is used to check whether the user is logged in to Facebook (and our application) at all.
The standard operation of loading and initializing the SDK is presented in the next code example.
Example — harnessing JavaScript Facebook API to work:
HTML — first of all, let’s ensure that the document has the fb-root element:
<div id="fb-root"></div>
JavaScript — let’s configure the parameters of our FB application (which we have to create at https://developers.facebook.com/:
window.fbAsyncInit = function() { // init the FB JS SDK FB.init({ appId : 'YOUR_APP_ID', // App ID from the App Dashboard status : true, // check the login status upon init? cookie : true, // set sessions cookies … xfbml : true // parse XFBML tags on this page? }); // Additional initialization code (e.g. adding Event Listeners) goes here … FB.getLoginStatus(function(response) { alert('OK'); }); }; // Load the SDK's source Asynchronously (function(d, debug){ var js, id = 'facebook-jssdk', ref = d.getElementsByTagName('script')[0]; if (d.getElementById(id)) {return;} js = d.createElement('script'); js.id = id; js.async = true; js.src = "//connect.facebook.net/en_US/all" + (debug ? "/debug" : "") + ".js"; ref.parentNode.insertBefore(js, ref); }(document, /*debug*/ false));
Thus, we can begin to use the JavaScript Facebook API.
To check in detail, whether the user is logged into our application, we can use the code as below:
FB.getLoginStatus(function(response) { if (response.status === 'connected') { // [1] // the user is logged in and has authenticated our app var uid = response.authResponse.userID; var accessToken = response.authResponse.accessToken; } else if (response.status === 'not_authorized') { // [2] // the user is logged in to Facebook, // but has not authenticated your app } else { // [3] // the user isn't logged in to Facebook. } });
Simply we have:
case [1] — the user is logged in to Facebook AND our application,
case [2] — the user is logged in to Facebook, BUT but didn’t pass the authorization process in our application,
case [3] — the user is NOT logged in to Facebook.
FB.login
The user can be not logged in. Then we can use the FB.login() method, which allows the user to authenticate in the application using OAuth dialog.
Example — using the FB.login method:
FB.login(function(response) { if (response.authResponse) { alert('Welcome! Fetching your information… '); FB.api('/me', function(response) { alert('Hello, ' + response.name + '!'); }); } else { alert('User canceled login or did not fully authorize.'); } });
With this method we can do another important operation: to ask the user for permission:
FB.login(function(response) { // … }, {scope: 'email,user_likes'}); // permissions
This may be necessary in case of many applications, as they are doing various things, so an access to various user’s data may be required.
Detailed information about permissions you can find in the documentation.
More code
Below I present a small JS library with frequently used functions. It is written in a simple way, as a set of separate functions, with the parameters available globally.
This means that we don’t pass them to the function; just define globally in the application templates, and then we simply call the function. Of course you may not like this approach, but here let’s focus on the JavaScript Facebook API features, to do certain things in our FB app. This can be adjusted to the architecture of our application. Anyway…
An example:
var window.psDialogName = 'Test'; // … var window.psDialogLink = 'http://url.com'; publishStory();
A set of functions below.
/** * @name fb.js * @description Toolbox - JavaScript Facebook API * @author Dominik Wlazlowski <office@directcode.eu> * @version 0.5 */ // publish a story to the user wall function publishStory() { FB.ui({ method: 'feed', name: window.psDialogName, caption: window.psDialogCaption, description: window.psDialogDesc, link: window.psDialogLink, picture: window.psDialogPicture }, function(response) { console.log('publishStory() response: ', response); }); } // publish a story to the wall of user friends function publishStoryForFriend() { randNum = Math.floor ( Math.random() * friendIDs.length ); var friendID = friendIDs[randNum]; // console.log('Opening a dialog for friendID: ', friendID); FB.ui({ method: 'feed', to: friendID, name: window.psDialogName, caption: window.psDialogCaption, description: window.psDialogDesc, link: window.psDialogLink, picture: window.psDialogPicture, user_message_prompt: window.psDialogPrompt }, function(response) { console.log('publishStoryForFriend() response: ', response); }); } // send invite friends request (simple) function inviteFriends() { FB.ui({ method: 'apprequests', message: window.ifDialogMessage }, requestCallback); } // invite specified friends function inviteFriendsByIDs() { FB.ui({ method: 'apprequests', suggestions: window.ifDialogFriendIDs, message: window.ifDialogMessage }, requestCallback); } // send a single (random) friend function inviteSingleFriend() { randNum = Math.floor(Math.random() * window.ifDialogFriendIDs.length); var friendID = window.ifDialogFriendIDs[randNum]; FB.ui({ method: 'apprequests', to: friendID, message: window.ifDialogMessage }, requestCallback); } function requestCallback(response) { // console.log('Invite friends request: ', response); return true; }
These are the typical things that we may have to do when developing Facebook applications.
The requestCallback function is a callback typical for AJAX, with response (answer from the API) as a parameter.
In example there’s no need to perform additional steps in this callback, so it’s common for couple functions (aimed for sending invitations to the application).
Some functions have a parameter like xxxFriendIDs, e.g. ifDialogFriendIDs. This is a list of user IDs separated by commas.
Such a list can be generated in different ways, but I would recommend here a proven solution. It’s a jQuery plugin with multiple possibilities of configuration.
This plugin is jquery-facebook-multi-friend-selector, available on GitHub:
https://github.com/mbrevoort/jquery-facebook-multi-friend-selector
In the parameters we can set the maximum number of friends, the the user can select, and define other options.
In this simple way we got a list of user IDs, that can be further processed.
More tools
The next great tool is fbrell. It allows to quickly launch and test solutions based on the JavaScript Facebook API.
Example:
http://www.fbrell.com/auth/account-info
Our code we can save under unique URL, get back later, and share with other developers.
More useful tools from Facebook:
https://developers.facebook.com/tools/
especially Graph API Explorer:
https://developers.facebook.com/tools/explorer
and Debug:
https://developers.facebook.com/tools/debug.
Bonus
At the end — a ready solution to implement AutoComplete feature: searching our Facebook friends.
Available here:
https://gist.github.com/aarongrando/1635158
great in its simplicity (less than 60 lines of code).
Summary
Facebook Application Programming today is a desirable skill. SDK for JavaScript and API are well developed and we can do a great job with them.
FB.getLoginStatus(function(response) {
alert(‘Thank you’);
});