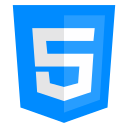
Hello! It’s time for further exploration of HTML5 Canvas, by implementing the next examples. Today, slightly more advanced things like animation and other graphical operations.
HTML5 Canvas in examples
So let’s start.
To run examples quickly, we use the same very simple page template, as in the previous article.
A simple animation using HTML5 Canvas
Simple, because we will be animating (moving) one graphic object.
To create animations we will use the JavaScript functions, such as setTimeout or setInterval. We also add graphics operations on the HTML5 Canvas element, such as “drawing” objects on Canvas or cleaning the area (ctx.clearRect(0, 0, x, y)) to refresh whole “picture”.
Consider the following code:
// … function draw() { var canvas = document.getElementById("canvas"); ctx = canvas.getContext("2d"); anim_img = new Image(size_x, size_y); // start animation after loading the image anim_img.onload = function() { setInterval('myAnimation()', 10); } anim_img.src = 'resources/ff_anim.png'; } function myAnimation() { // clean the area ctx.clearRect(0, 0, canvas_size_x, canvas_size_y); // after reaching the edge - change to the opposite // direction; so the effect of bounce from the edge if (x_icon < 0 || x_icon > canvas_size_x - size_x) { stepX = -stepX; } if (y_icon < 0 || y_icon > canvas_size_y - size_y) { stepY = -stepY; } // move the image … x_icon += stepX; y_icon += stepY; // and draw in new place ctx.drawImage(anim_img, x_icon, y_icon); } // …
We have two functions in this example:
– draw() — the main function called in document onload event, which performs the initial operations (a kind of assembly),
– myAnimation() — auxiliary function, which performs the main animation; is invoked every 10 [ms] in draw() function.
Full source code: available here.
And an example on-line: HTML5 Canvas Animation Demo.
3D text
In the previous article we had an example of writing text on HTML5 Canvas. Today one step further — 3D text.
// … function draw() { canvas = document.getElementById("canvas"); ctx = canvas.getContext("2d"); ctx.font = "60px Verdana"; ctx.fillStyle = "black"; ctx.textAlign = "center"; // center horizontally ctx.textBaseline = "middle"; // vertically render3dText(ctx, "Text 3D!", canvas.width / 2, 125, 6); } function render3dText(ctx, text, x, y, textDepth) { var i; for (i = 0; i < textDepth; i++) { ctx.fillText(text, x - i, y - i); } ctx.fillStyle = "#0094ed"; ctx.shadowColor = "#000000"; ctx.shadowBlur = 12; ctx.shadowOffsetX = textDepth + 2; ctx.shadowOffsetY = textDepth + 2; ctx.fillText(text, x - i, y - i); } // …
This case differs slightly from the plain text output; we have drawing lower layers of the text (it happens inside the render3dText() function) and adding a shadow.
A full example: here.
Now we’ll talk about operations on images.
Manipulating the image on the HTML5 Canvas element
In our example, we rotate the image with a given angle. This can be done quite simply by methods such as translate() and rotate() shared by the context of Canvas element.
Example:
// … function draw() { var canvas = document.getElementById("canvas"); var ctx = canvas.getContext("2d"); var img_obj = new Image(); img_obj.onload = function() { // setting the context on the middle of canvas … ctx.translate(canvas.width / 2, canvas.height / 2); // and rotating by 45 degrees ctx.rotate(-1 * Math.PI / 4); ctx.drawImage(this, -1 * img_obj.width / 2, -1 * img_obj.height / 2); }; img_obj.src = "res/html5_d.png"; } // …
After loading the image, we move to the middle of Canvas element, where we draw already rotated image:
Source code available: here.
More advanced operations are correspondingly more complicated. And here I would like to introduce a great library that simplifies the graphical operations on the Canvas element.
Bonus: the CamanJS library
The name of library can be explained as “Canvas manipulation in Javascript”.
The idea provides a simple in use interface to perform advanced and efficient graphical operations on HTML5 Canvas element, as well as on the pictures loaded.
Homepage of the project: http://camanjs.com/
Let’s look at a basic example:
Caman('#my-image', function () { this.brightness(10); this.contrast(30); this.sepia(60); this.saturation(-30); this.render(); });
Four graphical operations in four lines of code! It’s impressive when we realize how much work would be required to code this “by hand”.
More examples of CamanJS available here: http://camanjs.com/examples/ — as we can see this library is a great tool.
More advanced examples
Resources for the curious:
– a good example of HTML5 canvas animation implementation:
– the next part, with much more advanced example of implementation:
– a set of examples demonstrating the power and capabilities of HTML5, Canvas and JavaScript:
Summary
We discussed some (hopefully interesting) examples of graphical operations on the HTML5 Canvas element. There are also absolute basics useful for game programming.
Have fun with graphics generated by your code 🙂
HTML5 and javascript in Motion For Beginner Level course
Learning Course for HTML5 & JS in Motion for Jun Dev
https://www.udemy.com/html5-and-javascript-in-motion-for-beginner-level-course/?couponCode=easy+learning