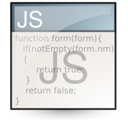
In this article we will talk about working with cookies in JavaScript. And no, it’s not about eating.
Working with cookies in JavaScript
Cookies — small information sent by a web server and stored on the user side (typically on the hard disk).
Cookies are most commonly used for counters, probes, online stores, sites that require login, advertising and to monitor the activity of visitors. They are just a way of maintaining “communication” between the user and the server.
The syntax of the HTTP header for cookie:
Set-Cookie: name=x; expires=date; path=mypath; domain=mydomain; secure
Description of parameters:
name=value — in fact cookie name is the only required parameter. It consists of any characters except colons, commas, whitespace and slashes (/). If you need to use them, they are usually encoded in a format suitable for URL (%XX), where XX is the ASCII code (e.g. %2F is encoded slash, %20 — encoded space char).
expires=date — this attribute tells the browser the expiration date of the cookie. It will be deleted from the disk when its expiration date is exceeded. If no expiration date specified, the cookie will be deleted when the session ends. The date must be entered in the correct format — for example: “Tuesday, 11-Nov-2007 08:39:09 GMT”.
domain=mydomain — this parameter specifies the visibility of cookie. When checking the cookie file on the client’s computer, the browser compares the stored domain with the domain server, which sends the headers.
path=mypath — the path attribute is applied to reduce the visibility of cookies. All pages included in this directory and its subdirectories will be able to use them.
secure — a parameter without a value, if specified, the cookie will be visible (sent) only when the connection is encrypted (currently possible using HTTPS).
A basic example — setting the cookie:
document.cookie = "myLang=DE";
Reading the cookie:
var cookies = document.cookie.split(/; /g);
When accessing, JavaScript gets a list of all the cookies, but as a string, not as an array. So we need to split a string, using a semicolon as the separator.
A toolbox
The code below is a comprehensive set of functions for handling cookies in pure JavaScript.
Create a cookie:
function writeCookie(cookieName, cookieValue, expires, domain, path, secureFlag) { if (cookieName) { var cookieDetails = cookieName + "=" + escape(cookieValue); cookieDetails += (expires ? "; expires=" + expires.toGMTString(): ''); cookieDetails += (domain ? "; domain=" + domain: ''); cookieDetails += (path ? "; path=" + path: ''); cookieDetails += (secureFlag ? "; secure": ''); document.cookie = cookieDetails; } }
Get unencrypted value of the cookie:
function readUnescapedCookie(cookieName) { var cookieValue = document.cookie; var cookieRegExp = new RegExp("\b" + cookieName + "=([^;]*)"); cookieValue = cookieRegExp.exec(cookieValue); if (cookieValue != null) { cookieValue = cookieValue[1]; } return cookieValue; }
Get a value from the cookie:
function readCookie(cookieName) { cookieValue = readUnescapedCookie(cookieName) if (cookieValue != null) { cookieValue = unescape(cookieValue); } return cookieValue; }
Delete the existing cookie(s):
function deleteCookie(cookieName) { var expiredDate = new Date(); expiredDate.setMonth(-1); writeCookie(cookieName, "", expiredDate); }
Create a cookie and set a single value:
function writeMultiValueCookie(cookieName, multiValueName, value, expires, domain, path, secureFlag) { var cookieValue = readUnescapedCookie(cookieName); if (cookieValue) { var stripAttributeRegExp = new RegExp("(^|&)" + multiValueName + "=[^&]*&?"); cookieValue = cookieValue.replace( stripAttributeRegExp, "$1"); if (cookieValue.length != 0) { cookieValue += "&"; } } else { cookieValue = ""; } cookieValue += multiValueName + "=" + escape(value); var cookieDetails = cookieName + "=" + cookieValue; cookieDetails += (expires ? "; expires=" + expires. toGMTString(): ''); cookieDetails += (domain ? "; domain=" + domain: ''); cookieDetails += (path ? "; path=" + path: ''); cookieDetails += (secureFlag ? "; secure": ''); document.cookie = cookieDetails; }
Get a single value stored within the cookie:
function readMultiValueCookie(cookieName, multiValueName) { var cookieValue = readUnescapedCookie(cookieName); var extractMultiValueCookieRegExp = new RegExp("\b" + multiValueName + "=([^;&]*)"); cookieValue = extractMultiValueCookieRegExp. exec(cookieValue); if (cookieValue != null) { cookieValue = unescape(cookieValue[1]); } return cookieValue; }
Delete a single value stored within the cookie:
function deleteMultiValueCookie(cookieName, multiValueName, expires, domain, path, secureFlag) { var cookieValue = readUnescapedCookie(cookieName); if (cookieValue) { var stripAttributeRegExp = new RegExp("(^|&)" + multiValueName + "=[^&]*&?"); cookieValue = cookieValue.replace( stripAttributeRegExp, "$1"); if (cookieValue.length != 0) { var cookieDetails = cookieName + "=" + cookieValue; cookieDetails += (expires ? "; expires=" + expires.toGMTString(): ''); cookieDetails += (domain ? "; domain=" + domain: ''); cookieDetails += (path ? "; path=" + path: ''); cookieDetails += (secureFlag ? "; secure": ''); document.cookie = cookieDetails; } else { deleteCookie(cookieName); } } }
Check if cookies are enabled in the browser:
function cookiesEnabled() { var cookiesEnabled = window.navigator.cookieEnabled; if (!cookiesEnabled) { document.cookie = "cookiesEnabled=True"; cookiesEnabled = new Boolean(document.cookie). valueOf(); } return cookiesEnabled; }
Summary
It’s basically everything you need to easily work with cookies in JavaScript.
And for the curious, the cookies under a magnifying glass at MDN.
Have fun with cookies!