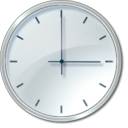
Today we take a closer look at ready-made tools to assist us in working with date and time. So libraries with functions to operate on date and time in JavaScript; formating, parsing, comparing, etc.
We already talked about basics of date and time functinos in JavaScript here. Now we turn to the more advanced tools.
The time functions in JavaScript
One of these types of tasks can be processing of date given as http://en.wikipedia.org/wiki/Unix_time to text and make it human readable.
We can write own function quickly:
function simpleTimeConverter(unix_timestamp) { var inputDate = new Date(unix_timestamp * 1000); var months = ['Jan','Feb','Mar','Apr','May','Jun', 'Jul','Aug','Sep','Oct','Nov','Dec']; var year = inputDate.getFullYear(); var month = months[inputDate.getMonth()]; var date = inputDate.getDate(); var hour = inputDate.getHours(); var min = inputDate.getMinutes(); var sec = inputDate.getSeconds(); var time = date + '. ' + month + ' ' + year + ' ' + hour; time += ':' + min + ':' + sec; return time; } var uxt = 1069497161; alert(simpleTimeConverter(uxt)); // 22. Nov 2003 11:32:41
Maybe we don’t work with date and time in JavaScript everyday, but it’s good to have quick and tested solutions. So we need good libraries with time functions.
Below we placed solutions in our opinion worthy of attention.
Date and time libraries for JavaScript
The date() function from php.js
A simple solution as first. In already discussed library php.js there is an implementation of the date() function known from PHP. It is excellent for formatting dates, including their variants for different nationalities (with a little effort from the programmer).
The possibilities offered by Dojo Toolkit
Some time ago we talked about Dojo Toolkit. This great library also offers date and time support, including calculations on dates or date formatting for multiple languages, and more.
http://dojotoolkit.org/documentation/tutorials/1.9/dojo_date/
moment.js
This is a powerful JavaScript library for parsing, validation, formatting, and other operations on dates.
URL: http://momentjs.com/
XDate
Another interesting library, which is a wrapper around the native Date object. It provides many functions for manipulating dates, formatting, and parsing.
Countdown.js
This is a library for calculating differences between dates and their clear presentation.
Later.js
This is another interesting tool for calculations on dates, but more importantly, these calculations are used for planning. This is because the library is used for scheduling tasks to be performed. It’s like a simple cron on JavaScript.
URL: http://bunkat.github.io/later/
Summary
When we look at the presented solutions closer, we see that even JavaScript offers great opportunities when it comes to working with date and time. Everyone can find something suitable for your needs.