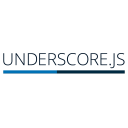
Functional programming is a programming methodology being a variation of declarative programming, in which functions belongs to the fundamental values, and the emphasis is on valuation of (often recursive) functions.
It’s used also by the underscore.js library, which supports functional programming in JavaScript.
Functional programming in JavaScript and underscore.js
This JavaScript library offers a variety of facilities available in Prototype.js (or e.g. in Ruby language!), BUT doesn’t overload the native JS objects.
On underscore.js another popular library is based: Backbone.js! The author of both libraries is also the creator of CoffeeScript.
Examples of use — underscore.js
_.map([1, 2, 3], function(num){ return num * 3; }); // => [3, 6, 9]
In such a simple way we can perform mapping of all the values in the list given as an argument.
_.times(4, function() { console.log("JS") });
This example will print “JS” in the console four times.
Underscore.js contains more than 100 useful functions, such as each, map, filter, find, invoke.
There are also utility functions.
Example — noConflict:
var underscore = _.noConflict(); underscore.times(4, function() { console.log("JS") });
In this library we will find a whole range of possibilities, including support for reflection.
Example:
_.functions(_); // => ["all", "any", "bind", "bindAll", "clone", "compact", …
The function functions_.functions(object) (also having alias “methods”), returns a sorted list of the names of all methods in the object.
In underscore.js we can expect functions such as escape:
_.escape('Curly, Larry & Moe'); // => "Curly, Larry & Moe" _.unescape('Curly, Larry & Moe'); // => "Curly, Larry & Moe"
Extract value from the object? Sure:
_([1, 2, 3]).value(); // => [1, 2, 3]
And many other possibilities. The underscore.js is a brilliant library. Not only because of *what* functions it offers, but also *how* they are done, and how we can use them.
Resources
– functional programming
http://eloquentjavascript.net/1st_edition/chapter6.html
– underscore.js homepage
We also recommend the book: “Functional JavaScript” (O’Reilly).
Download — a test page with some functions of underscore in action:
http://directcode.eu/samples/underscore.zip
Functional programming in JavaScript and the underscore.js library — summary
Functional Programming in JavaScript is a very interesting topic. As an example we can take the underscore.js library, which is a very neat tool.
_thankyou();