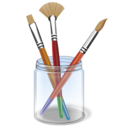
Some time ago we got a small job. The subject: small research and creation of simple on-line editor for images, which should be editable from HTML5 Canvas and JavaScript level. Then the user should be able to save / send the final image. Today we present the basic elements and some examples related to this subject.
HTML5 Canvas, JavaScript and images
Basically we need (all the necessary libraries are included in our ready example):
– advanced JavaScript libraries: base64.js, canvas2image.js, jsmanipulate.js,
– PHP files, where we will process the data,
– images (jpg, png) to process.
Steps to do:
1. Load image from file JPG or PNG file to the HTML5 Canvas element (or draw a simple picture directly, using Canvas methods)
2. Perform some graphics operations on this picture
3. Get the modified contents of the Canvas, so that you can save it in a file
The 3rd point was most important in our case.
A whole magic was performed through one simple method — toDataURL() of the HTML5 Canvas object:
var strDataURI = oCanvas.toDataURL(); // returns "data:image/png;base64,iVBORw0KGgoAAAANSUhEUgAAAMgA…"
This method returns the Canvas content as data: URI. This allows for further operations, such as converting and saving the data in the form of the final file.
The data: URI string can be used directly as src attribute of image tag, and displayed in the browser:
<img src="data:image/png;base64,iVBORw0KGgoAAAANSUhEUgA… " />
Let’s go to the practice and sample code.
Example — simple UI:
… <div id="wrapper"> <canvas id="cnv" width="200" height="200"></canvas> <br /><br /> <div id="tb"><button onclick="testing()">Test</button></div> </div> …
JavaScript:
var canvas = document.getElementById('cnv'); if (canvas.getContext) { var context = canvas.getContext('2d'); // simple drawing on canvas context.beginPath(); context.moveTo(75,25); context.quadraticCurveTo(25,25,25,62.5); context.quadraticCurveTo(25,100,50,100); context.quadraticCurveTo(50,120,30,125); context.quadraticCurveTo(60,120,65,100); context.quadraticCurveTo(125,100,125,62.5); context.quadraticCurveTo(125,25,75,25); context.stroke(); } else { alert('Error in canvas context loading'); } // the handler function testing() { // save data as PNG (default) var strDataPng = canvas.toDataURL(); // alert(strDataPng); var my_url = "processor_png.php"; $.ajax({ type: "POST", url: my_url, data: { filecode: strDataPng } }).done(function( msg ) { alert("PNG file saved!"); }); // … }
In this example, we draw a completely new picture, only with HTML5 Canvas methods. Then we get the data representing this image and we send it via AJAX to PHP script, which saves these data to the file.
A simple example of such PHP script:
… if (isset($_POST["filecode"])) { // $imageData = $GLOBALS['HTTP_RAW_POST_DATA']; $imageData = $_POST["filecode"]; // cleanups $filteredData = substr($imageData, strpos($imageData, ",") + 1); // decode right data $unencodedData = base64_decode($filteredData); // output $fp = fopen("tests/file_png.png", "wb"); fwrite($fp, $unencodedData); fclose($fp); } …
This code fetches and decodes the data and then saves it to a file on the server.
On-line examples:
– JPG file test
http://directcode.eu/samples/html5_canvas_mod_script_js_blog/test_jpg.php
– PNG file test
http://directcode.eu/samples/html5_canvas_mod_script_js_blog/test_png.php
Examples for download:
https://github.com/dominik-w/js_html5_com/tree/master/html5_canvas_mod_script_js_blog
The elements of this case study were inspired by, among others, the articles from the nihilogic.dk page.
Summary
That’s all for today. I hope the discussed topic was interesting and will help someone with issues related to processing, getting and saving the data from HTML5 Canvas element.
Thank you and have fun!