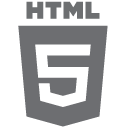
In the second, summary part of the basic course HTML5, we look closely at the next great elements of the language.
HTML5 short course: the Modernizr library
At the beginning we add yourself to facilitate work in the form of the Modernizr library.
This is a JavaScript library that detects the availability of HTML5 and CSS3 features in our browser.
We can download the Modernizr from official website, where is also a tool allowing us to choose the test only for those features, that interests us. Ad by the way we can see a full list of features, that Modernizr can detect.
We may also use the CDN, such as cdnjs.com.
So let’s add the library to our project:
<script src="modernizr.js" type="text/javascript"></script>
And add the no-js class to our main markup (html):
<html class="no-js"> …
Modernizr runs automatically, so you can immediately use it, for example, to check whether the browser supports the Canvas element:
if (Modernizr.canvas) { // let's draw! } else { // no native canvas support available }
As we can see, the tests performed by Modernizr allows not only for instant detect the availability of the required functionality, but also the implementation of an alternative for browsers without support for desired feature.
Actions that the above code executes, we can also write manually in JavaScript:
function supports_canvas() { return !!document.createElement('canvas').getContext; }
But I prefer the Modernizr’s way.
Please note that Modernizr is not a library that adds missing functionality to the browser.
There is some kind of such solution — the html5shiv library, which may add support for some HTML5 elements in IE9+.
In newer versions of Modernizr we will find many useful things, such as:
– conditional loading resources using load() method,
– Media Queries ( e.g.: Modernizr.mq(‘only all and (max-width: 500px)’) ),
– detection of rendering engines prefixes used by the browser ( e.g.: Modernizr.prefixed(‘transform’) ).
Let’s look at some other examples
Checking if WebGL is available:
if (Modernizr.webgl) { // game_start(); } else { alert("Sorry, you need better browser to play … "); }
HTML5 great supports playback of audio and video files. Details will be discussed in next articles. Not every browser and can support these features, so we should add a test:
// way #1 if (Modernizr.video) { // play movie } // way #2 - pure JavaScript: function supports_video() { return !!document.createElement('video').canPlayType; }
And in this way we can detect the availability of Local Storage feature:
if (Modernizr.localstorage) { // store some data } // or classic: function supports_local_storage() { try { return 'localStorage' in window && window['localStorage'] !== null; } catch(e) { return false; } }
Modernizr library is simply pleasant to use.
As the premise is the HTML5 short course, let’s discuss Input types — great form fields offered by HTML5.
Input fields in HTML5
So what do we have here:
<input type="search"> <!-- for search boxes --> <input type="number"> <!-- for spinboxes --> <input type="range"> <!-- for sliders --> <input type="color"> <!-- for color pickers --> <input type="tel"> <!-- for telephone numbers --> <input type="url"> <!-- for web addresses --> <input type="email"> <!-- for email addresses --> <input type="date"> <!-- for calendar date pickers --> <input type="month"> <!-- for months --> <input type="week"> <!-- for weeks --> <input type="time"> <!-- for timestamps --> <input type="datetime"> <!-- absolute date/time stamps --> <input type="datetime-local"> <!-- for local dates and times -->
As you can see the developer receives a lot of convenience, since he doesn’t have to implement own solutions for receiving from the data of a specific type / format from the user. The corresponding fields are already built in HTML5.
Of course, why not take a test of availability — Modernizr allows even to test for field types.
Example:
if (!Modernizr.inputtypes.date) { // no native support for <input type="date"> // try to use some JavaScript Framework perhaps … }
The next subsection contains concise examples about the use of the forms in HTML5.
HTML5 short course: examples of forms
As first — the Placeholder:
<form> <input name="q" placeholder="Go to a Website"> <input type="submit" value="Search"> </form>
The Autofocus attribute:
<form> <input name="q" autofocus> <input type="submit" value="Search"> </form>
Email field type:
<form> <input type="email"> <input type="submit" value="Go"> </form>
The number field with additional parameters, such as values range:
<input type="number" min="0" max="12" step="2" value="5">
Of course in JavaScript we find a dedicated methods for working for such fields:
– input.stepUp(n); // increases the value by n
– input.stepDown(n) // decreases by n
– input.valueAsNumber // returns the current value as a floating point number (the input.value property always returns string)
And check the availability of appropriately using Modernizr:
if (!Modernizr.inputtypes.number) { // … }
Slider and the range field type:
<input type="range" min="0" max="10" step="2" value="6">
Color picker — color type of field:
<input type="color">
So it generally looks for fields.
Let’s look at a small example of form validation. The “novalidate” attribute specifies which fields will not be validated. For required field we use “required” markup:
<form novalidate> <input type="email" id="e-addr"> <input type="submit" value="Subscribe"> </form> <form> <input id="q" required> <input type="submit" value="Search"> </form>
Another example:
<label>Email:</label> <input name="email" type="email" required>
Microdata
The microdata specification in HTML5 provides a way for marking the content by labels. Their aim is to describe the specific types of information — for example, an opinion or events. Using microdata allows, for example, to declare that the photograph is available on a specific license.
A simple example:
<div item> <p>My name is <span itemprop="name">Hans</span>.</p> <p>I am <span itemprop="nationality">German</span>.</p> </div>
Checking availability in JavaScript:
function supports_microdata_api() { return !!document.getItems; }
Other examples — taken from http://diveintohtml5.info/:
<p> <img src="http://www.example.com/photo.jpg" alt="[me smiling]"> </p> <section itemscope itemtype="http://data-vocabulary.org/Person"> <img itemprop="photo" width="204" height="250" src="http://diveintohtml5.info/examples/2000_05_mark.jpg" alt="[Mark Pilgrim, circa 2000]"> <dd itemprop="address" itemscope itemtype="http://data-vocabulary.org/Address"> <span itemprop="street-address">100 Main Street</span><br> <span itemprop="locality">Anytown</span>, <span itemprop="region">PA</span> <span itemprop="postal-code">19999</span> <span itemprop="country-name">USA</span> </dd>
We can say that’s some way of extending HTML5.
Additional articles:
New semantic elements
HTML5 have the new semantic elements. Among them we can mention:
<section>
Element represents a generic section of the document / application, usually used for thematic grouping of content and includes a header.
<nav>
Basically — an element that represents the section in which we place links to other sites (navigation).
<article>
This element is used for elements such as forum posts, blog entries, comments, or just an article.
<aside>
In such elements usually placed side components, such as sidebars, advertising space or whatever what we would like to separate from the main content.
<hgroup>
This element will group headers (h1-h6) of the section, especially multi-level.
<header>
In this element usually put the header page; we can group headers or whole group (hgroup), logo or search form, etc.
<footer>
Footer of the page.
There are other elements, such as <time> for date / time, or the <mark> element.
Example:
<article> <header> <h1>A syndicated post</h1> </header> <p>Lorem ipsum blah blah…</p> </article> <time datetime="2009-05-23" pubdate="pubdate">May 23, 2009</time>
Example #2 — nav:
<div id="nav"> <ul> <li><a href="#">home</a></li> <li><a href="#">blog</a></li> <li><a href="#">gallery</a></li> <li><a href="#">about</a></li> </ul> </div>
Example #3 — footer:
<footer> <p>(c) 2012 <a href="#">Author</a></p> </footer>
The use of elements is not rigidly defined. We can, for example, combine and nest elements aims to obtain extensive footer (“fat footer”).
Example:
<footer> <nav> <h1>Navigation</h1> <ul> <li><a href="/">Home</a></li> <li><a href="/folio.html">Portfolio</a></li> <li><a href="/offer.html">Offer</a></li> </ul> </nav> <nav> <h1>Contact</h1> <ul> <li><a href="/contact.html">Contact</a></li> <li><a href="/help.html">Help</a></li> <li><a href="/sitemap.html">Site Map</a></li> </ul> </nav> <section> <h1>Social</h1> <ul> <li><a href="http://twitter.com/xyz">Twitter</a></li> <li><a href="http://facebook.com/xyz">Facebook</a></li> </ul> </section> <p class="copyright">Copyright © 2012 Xyz</p> </footer>
I recommend also examples with description on W3Schools,
Summary
And thus concludes the second part of the HTML5 short course. But in any case we not finish exploring the great possibilities of the HTML5 language.
http://davidwalsh.name/html5-input-types-alternative