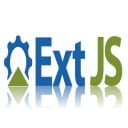
Today’s course is little bit different. This is a one-piece mini-course of Ext JS library. It aims to introduce this powerful JavaScript framework.
The Ext JS
It’s a framework for JavaScript language, which supports building interactive web applications, user interfaces and more, using techniques such as DOM or AJAX.
Ext JS has among others many built-in controls of GUI, such as date-picker, HTML editor, controls for trees and grids, toolbars and menus in the style of desktop applications, sliders or charts based on vector graphics. And furthermore other advanced functionality.
A wealth of possibilities was presented briefly in demo examples.
You should, however, accept the license, because the library depending on purpose, is available on the Open Source or commercial license.
Starting with Ext JS
Get Ext JS from the official website.
It is also possible to take advantage of the CDN:
<script type="text/javascript" charset="utf-8" src="http://cdn.sencha.io/ext-4.2.0-gpl/ext-all.js"></script>
We test whether everything is as it should be:
Ext.onReady(function() { alert("Congratulations - Ext JS is loaded"); // here we add the code based on Ext JS });
In jQuery we have .ready event:
$(document).ready(function() {
// …
});
And we can see some similarity here. Ext JS has the Ext.onReady event, which determines that the library has been successfully loaded.
Accessing elements
Getting an element, what in “pure” JavaScript is done through:
var myDiv = document.getElementById('myDiv');
and in many frameworks (like Prototype JS or jQuery) we have “$” functions:
$(‘myDiv’);
https://www.instagram.com/distinctivetravelscom
However, in Ext JS it’s simply Ext.get():
Ext.onReady(function() { var myDiv = Ext.get('myDiv'); });
Performing operations on elements
Ext JS provides a number of methods known probably from other frameworks, for example adding CSS classes to the element dynamically, and also more specific for Ext JS, like Element.center().
Let’s look at examples:
// flash - a background item is highlighted myDiv.highlight(); // adding CSS class myDiv.addClass('red'); // centered position of the element myDiv.center(); // setting a certain transparency to the element myDiv.setOpacity(.25);
More about Ext.element we can find in right place of the documentation.
A step ahead: DOM.
Selecting DOM nodes
Sometimes, for various reasons, we need to look for and retrieve elements by the parameters other than their IDs. Then we have to search the document by CSS classes used for elements, or by the names of HTML tags.
We discussed this already in the case of Prototype JS and jQuery. Ext JS works on a similar principle. There is the Ext.select() applied.
Example — each paragraph will be highlighted:
Ext.select('p').highlight();
DomQuery in Ext JS supports a wide range of options for getting items, including most of DOM selectors (W3C CSS3), basic XPath, HTML attributes, and others.
Example:
Ext.query("span"); // span elements of the document Ext.query("span", "foo"); // span with ID = foo
We recommend a fairly detailed article about the basics of DomQuery.
Events
To control and event handling, Ext JS provides powerful methods with a friendly syntax.
A simple example of an event handler in Ext JS:
HTML
<input type="button" id="myBtn" value="Click" />
JavaScript
Ext.onReady(function() { Ext.get('myBtn').on('click', function() { alert("You clicked the button"); }); });
Button is only an example, we obviously can associate an event with any other element of the document, e.g.:
Ext.onReady(function() { Ext.select('p').on('click', function() { // do something … }); });
Passing arguments to the event handler
A simple example of how to do this, by creating a delegate through createDelegate:
var myHandler = function(evt, t, o, myArg1, myArg2, myArg3) { alert('Test'); } Ext.select('.element-type1').addListener( 'click', myHandler.createDelegate(this, [1, 'Abc', 123], true)); Ext.select('.element-type2').addListener( 'click', myHandler.createDelegate(this, [2, 'Def', 555], true));
The arguments used for the event handler is invoke, are:
evt, t, o these are standard arguments passed to the event handler in Ext JS, above all, the target element of the event and the arguments (collection of arguments passed to the function).
Whereas, arguments myArg1, myArg2, myArg3 are our own, optional parameters. Sometimes a programmer might need them, so there is easy possibility to pass them.
Summary
That’s all in our mini-course of the Ext JS. It’s very powerful framework — just look at the examples of applications developed on the basis of Ext JS.
For the curious, a link to interesting tutorials, and of course to the official documentation (for various Ext JS versions).
E(xt)njoy JS!