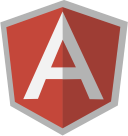
Today we will continue learning the Angular JS framework, by getting to know better the most important parts of AngularJS application, as well by analyzing small practical examples.
Learning AngularJS — further steps
The previous part describes basic aspects of this great framework.
We already know that AngularJS is a JavaScript language framework, and it extends HTML through ng-directives. The previous part of this course shows also a simple example with data-binding. We are also aware of many useful directives, for example ng-init:
<div ng-app="" ng-init="firstName='Joe'"> <p>The name is <span ng-bind="firstName"></span></p> </div>
Let’s add AngularJS expressions to this. They exist in double brackets, e.g. {{ expression }} and on them AngularJS can execute its magic. The expressions can also be stored in the ng-bind=”expression” directive.
Example — Angular will handle adding two numbers in the expression:
<div ng-app=""> <p>Result: {{ 5 + 5 }}</p> </div>
Expressions in AngularJS work well with all types of data
– numbers:
<div ng-app="" ng-init="quantity=1;cost=9"> <p>Total price €: {{ quantity * cost }}</p> </div>
– strings:
<div ng-app="" ng-init="firstName='Donnie';lastName='Brasco'"> <p>The name: {{ firstName + " " + lastName }}</p> </div>
– objects and arrays also typically for JavaScript:
<div ng-app="" ng-init="person={first:'Joe',last:'Pistone'}"> <p>The name: {{ person.last }}</p> </div>
<div ng-app="" ng-init="points=[1,5,9,20,40]"> <p>Pick up array element: {{ points[3] }}</p> </div>
AngularJS expressions, same as JavaScript expressions, can contain literals, operators and variables. The main difference between them is that AngularJS expressions can be stored inside of HTML.
Learning AngularJS — models and controllers
We use the ng-controller directive to define an element for a controller. Let’s analyze a simple example. We create HTML elements and a module, that implements a controller.
HTML
<div ng-app="myApp" ng-controller="MyCtrl"> First Name: <input type="text" ng-model="firstName"><br> Last Name: <input type="text" ng-model="lastName"><br> <br> Full Name: {{firstName + " " + lastName}} </div>
JavaScript
var app = angular.module('myApp', []); app.controller('MyCtrl', function($scope) { $scope.firstName = "Donnie"; $scope.lastName = "Brasco"; });
As we know, the $scope is a glue between a model and controller. In our example AngularJS will set values in an expression, and also will take care about updating them, when the user will change values of input fields.
AngularJS modules
As we have just seen, the module is created through the angular.module function:
<div ng-app="myApp">…</div>
var app = angular.module("myApp", []);
Models — ng-model directive
We have seen how it works in practice. The ng-model directive binds the value from HTML control (input, select, textarea) with application data.
A simple example:
<div ng-app="myApp" ng-controller="MyCtrl"> Name: <input ng-model="name"> <h3>You entered: {{name}}</h3> </div>
var app = angular.module('myApp', []); app.controller('MyCtrl', function($scope) { $scope.name = "Donnie Brasco"; });
Binding can be in two directions (in example input with ng-model directive and {{name}} expression).
More abut AngularJS models:
http://www.w3schools.com/angular/angular_model.asp
AngularJS — controllers
AngularJS application controllers, which controls its data and behavior, we define using the ng-controller directive.
Directive ng-model binds inputs with properties of the controller. There is also $scope — an application object, that owns the variables and application functions, which are grouped in a given scope.
Inside the controllers we can perform operations on data.
Example #1 — angular.uppercase():
<div ng-app="myApp" ng-controller="MyCtrl"> <p>{{ x1 }}</p> <p>{{ x2 }}</p> </div>
var app = angular.module('myApp', []); app.controller('MyCtrl', function($scope) { $scope.x1 = "John"; $scope.x2 = angular.uppercase($scope.x1); });
Example #2 — using controllers in AngularJS:
<div ng-app="myApp" ng-controller="MyCtrl"> First Name: <input type="text" ng-model="firstName"><br> Last Name: <input type="text" ng-model="lastName"><br> <br> Full Name: {{fullName()}} </div>
The fullName() expression is a call of the function.
var app = angular.module('myApp', []); app.controller('MyCtrl', function($scope) { $scope.firstName = "John"; $scope.lastName = "Doe"; $scope.fullName = function() { return $scope.firstName + " " + $scope.lastName; }; });
To define a controller, we can also use the following syntax:
var myApp = angular.module('myApp', []); myApp.controller('MyCtrl', ['$scope', function ($scope) { $scope.lastName = 'Brasco'; … }]);
Tip #1
The controllers we can also keep in external files, and include them to an application (e.g. myController.js).
Tip #2
The recommended naming convention for AngularJS controllers is “PascalCase”, for example DemoController:
angular.module('myApp', []). controller('DemoController', function($scope) { … });
More about AngularJS controllers:
http://www.w3schools.com/angular/angular_controllers.asp
$scope
The Scope is the glue between controllers and views. Scope binds the data between the view (HTML) and the controller (JavaScript). The programmer don’t have to worry about this.
Example — using Scope:
<div ng-app="myApp" ng-controller="MyCtrl"> <h2>{{car}}</h2> </div>
var app = angular.module('myApp', []); app.controller('MyCtrl', function($scope) { $scope.car = "Volvo S80, 4.4 V8"; });
$scope is an object with properties and methods, which are provided both for the view that the controller.
Root Scope
All AngularJS applications have the $rootScope, which is a “master” scope, created on HTML with ng-app directive.
rootScope is available for the whole application. If a variable has the same and in the rootScope and “lower” scopes (current), it will be overwritten (only in those scopes).
Example — rootScope:
<div ng-app="myApp"> <p>The rootScope's color:</p> <h1>{{color}}</h1> <div ng-controller="MyCtrl"> <p>The scope of the controller's color:</p> <h1>{{color}}</h1> </div> <p>The rootScope's color is still:</p> <h1>{{color}}</h1>
var app = angular.module('myApp', []); app.run(function($rootScope) { $rootScope.color = 'green'; }); app.controller('MyCtrl', function($scope) { $scope.color = "red"; }); </div>
More information here:
http://www.w3schools.com/angular/angular_scopes.asp
Learning AngularJS — sample application
It’s time for a little bit more practice with AngularJS. Our simple application will process the list of messages. AngularJS will take care about painless rendering of the data in DOM.
Let’s assume we have a list of messages from some data source (e.g. obtained via AJAX, API, etc) as an array of objects.
First of all, let’s assign this array to the property in our scope:
var myApp = angular.module('myApp', []); myApp.controller('EmailsCtrl', ['$scope', function ($scope) { // an object for the messages $scope.emails = {}; // sample data, obtained from the the server // as an array of objects $scope.emails.messages = [ { "from": "Joe Pistone", "subject": "Something happened…", "sent": "2014-10-02T08:05:54Z" },{ "from": "Donnie Brasco", "subject": "Forget about it", "sent": "2014-09-22T22:22:00Z" },{ "from": "Sonny Black", "subject": "What the ****?", "sent": "2014-09-21T14:14:14Z" } ]; }]);
Now let’s create a view, which implements simple processing of data from $scope:
<div ng-app="myApp"> <div ng-controller="EmailsCtrl"> <ul> <li ng-repeat="message in emails.messages"> <p>From: {{ message.from }}</p> <p>Subject: {{ message.subject }}</p> <p>{{ message.sent | date:'MMM d, y h:mm:ss a' }}</p> </li> </ul> </div> </div>
Ready! We can see here the usage of ng-repeat directive, which implements a simple logic of procssing in loop, as well using AngularJS filters (for data formatting).
More about fiters we’ll write in the next part.
Scope functions
We can add few lines in our JS code, to extend the $scope by a method for removing messages:
myApp.controller('MainCtrl', ['$scope', function ($scope) { $scope.deleteEmail = function (index) { $scope.emails.messages.splice(index, 1) }; }]);
Our view needs only the following line of code:
<a ng-click="deleteEmail($index)">Delete email</a>
with the ng-click directive. AngularJS will handle the rest.
Please pay attention for $index parameter, which represents the number of current element.
Global static data
The data can be shared between Angular’s $scope and the rest of web page. For example:
window.globalData = {}; globalData.emails = ** here an array with list of messages **
Such data can be easy assigned to a variable inside of scope:
myApp.controller('EmailsCtrl', ['$scope', function ($scope) { $scope.emails = {}; $scope.emails.messages = globalData.emails; }]);
AngularJS is really helpful in programming modern web applications.
Resources
A rich set of ready AngularJS recipes
Learning AngularJS — summary
Here we finish the part two of basic AngularJS course. Models, controllers, views and scope. When we know them, we can already do really a lot.
In the next part we will take a look on forms and validation, filters, services and other topics of this great JS framework.
Thank you.