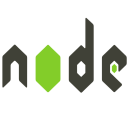
JavaScript’s popularity is obvious. And solutions such as node.js have a big influence on this. For a longer time, we can see and hear a lot about this technology.
We also use node from some time, and… we can do really cool things, so for sure it’s something worthy of attention. And finally I have some time to write about node.js.
JavaScript has a bright future, but also I think we can consider node.js as a mandatory skill.
node.js — introduction
Node.js is a platform that allows to run JavaScript code. It is built on V8 JS engine — the same is used e.g. in Google Chrome.
And so what? Well, this JavaScript code we can run outside the browser! And thanks to the API and node.js modules we have a lot possibilities, typical for server-side technologies, such as file I/O, database communication or socket.io.
Node provides us with a powerful set of tools!
To install extensions we use NPM:
Node.js is a programming environment designed to create highly scalable web applications, especially web servers written in JavaScript.
Node.js allows you to create applications using asynchronous event-driven I/O system. Obviously, this is an open source project.
OK, enough talking — let’s code!
How to use node.js
Working with node.js can start in the blink of an eye. We need node and npm tools — installed and available in command line.
For this, simply grab and install the version of node.js for our system:
Having node.js installed, you can run the test code.
The simplest example — run node in command line and write:
console.log("Hello node!"); // -> Hello node!
A better idea would be to save the code in the file, e.g. test1.js. Then we can call:
$node test1.js
// -> Hello node!
We can also run the script in debug mode:
$ node debug test.js
The console.log function is the main way to display messages for the user (developer).
Similar to alert function on client-side. Btw. we can send alert to our server written in node as a response, for example:
var str = "<html>…<script> alert here </script>…</html>"; res.end(str);
With the res.end method we answer for the request. Let’s write the simplest server to see node.js in action.
The server in node.js
This is actually the most basic thing.
Example:
// my_server.js var http = require('http'); http.createServer(function handler(req, res) { res.writeHead(200, {'Content-Type': 'text/html'}); res.end('Hello World\n <h1>Node.js!</h1>'); }).listen(1337, '127.0.0.1'); console.log('Server running at http://127.0.0.1:1337/');
Run:
$ node my_server.js
Now we can open URL on a specific port, and see the content sent from our server:
http://127.0.0.1:1337
Attention:
after making changes in the code, we need to restart the server to apply them.
To terminate the script, type Ctrl + C in console.
Request and response — processing data from the user
Another example is a simple, but complete code showing how to process the user request, and send an answer.
To get the data from user we use the ‘data’ event, which is triggered when the data stream is coming from the HTTP request.
Example:
// add necessary modules var http = require('http'); var qs = require('querystring'); // build a simple form var pageHTML = '<html>' + '<head>' + '<title>Add something</title>' + '<meta charset="utf-8">' + '</head>' + '<body>' + '<form method="post" action="">' + '<div>' + '<label for="nickname">Nickname:</label>' + '<input type="text" name="nickname">' + '</div>' + '<div>' + '<input type="submit" value="send it">' + '</div>' + '</form>' + '</body>' + '</html>'; // create server and process data var server = http.createServer(function (req, res) { var requestData = ''; // check HTTP method and show the right content if (req.method === "GET") { res.writeHead(200, {'Content-Type': 'text/html'}); res.end(pageHTML); // serve our HTML code } else if (req.method === "POST") { req.setEncoding('utf-8'); req.on('data', function(data) { requestData += data; }); req.on('end', function() { var postData = qs.parse(requestData); res.writeHead(200, {'Content-Type': 'text/html'}); res.end('<h1>Your nick: '+ postData.nickname + '</h1>'); }); } }); server.listen(1337, '127.0.0.1'); console.log('Server running at http://127.0.0.1:1337/');
The server checks the type of request. If GET — serves prepared HTML page with the form.
If POST — processes the data:
… req.on('data', function(data) { requestData += data; }); …
Then in the ‘end’ event, sends a response to the user. This is an event occurring when the data stream ends, and we can send a reply.
Of course we used a very simple approach — HTML code in a variable. But do not worry — in node.js we can use the templates and many other facilities.
Installing node.js modules
Modules bring us huge possibilities. Their installation is very simple:
$ npm install module_name
For example:
$ npm install mysql
Node also has application development frameworks. Very popular is Express.js:
$ npm install express
Communication on sockets:
$ npm install socket.io
About sockets and socket.io we will write in next articles.
More about node.js
The main point to refer is the node.js API documentation:
http://nodejs.org/api/synopsis.html
Summary
This concludes a quick introduction to the wonderful node.js technology. People can do amazing things by adding node.js to the projects based on other technologies, such as PHP, Raspberry PI, or mobile.
In subsequent articles about node.js we will work, among others, with DB communication (MySQL, Mongo), sockets and more.
Enjoy.js
[…] — node.js […]
“Why was node.js a big deal?”
=>
“It just happened to be in the right time, at the right place.”
https://medium.com/@slsoftworks/javascript-world-domination-af9ca2ee5070
[…] presenting basics of node.js and how to work with databases, it’s time to reach for even more interesting […]