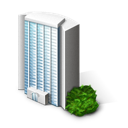
Today we present the tutorial about creating a simple web application, which stores data in localStorage. The app will be able to save and store the settings of its own appearance.
Web storage tutorial
1. Let’s start by adding jQuery and simple CSS styles for UI elements:
… <script src="http://code.jquery.com/jquery-1.9.1.min.js"> </script> <style> #main { border: solid 1px #00e; margin: 0 auto; padding: 5px; text-align: center; width: 33%; } </style> …
2. Create a simple form in the body section:
<body> <section id="main"> <form onsubmit="javascript:storeSettings()"> <label>Select font size: </label><br /> <input id="your_font" type="number" max="50" min="5" value="14"> <br /> <label>Select border size: </label><br /> <input id="your_border" type="number" max="10" min="1" value="1"> <br /> <label>Select color for background: </label><br /> <input id="your_color" type="color" value="#ffffff"> <br /> <p> <input type="submit" value="Save settings"> <input onclick="removeSettings()" type="reset" value="Remove settings"> </p> </form> </section> </body>
Now we can code handlers in JavaScript.
3. Let’s write a simple function to retrieve the data from UI and to save it in localStorage:
function storeSettings() { if ('localStorage' in window && window['localStorage'] !== null) { try { var your_color = $('#your_color').val(); var your_font = $('#your_font').val(); var your_border = $('#your_border').val(); localStorage.setItem('bgcolor', your_color); localStorage.setItem('fontsize', your_font); localStorage.setItem('border', your_border); } catch (e) { alert('An error occured'); } } else { alert('Sorry, your browser must have localStorage support'); } }
The function starts with checking if localStorage is available in the browser.
4. Let’s add an auxiliary function that sets the default values:
function setDefaults() { document.body.style.backgroundColor = '#ffffff'; document.body.style.fontSize = '14px'; $('#main').css('border', 'solid 1px #00e'); $('#your_color').val('#ffffff'); $('#your_font').val('14'); $('#your_border').val('1'); }
As we set the default values in more than one place in the code, it’s good to save this action as a separated function.
5. Code handler for the “Remove settings” button:
function removeSettings() { localStorage.removeItem('bgcolor'); localStorage.removeItem('fontsize'); localStorage.removeItem('border'); setDefaults(); }
This function removes our data saved in localStorage.
6. At the end let’s add the function, which retrieves settings from localStorage (or sets default, if there’s no data yet) and applies these settings for UI element of our application:
function useSettings() { if (localStorage.length != 0) { document.body.style.backgroundColor = localStorage.getItem('bgcolor'); var font_size = localStorage.getItem('fontsize'); document.body.style.fontSize = font_size + 'px'; var border_size = localStorage.getItem('border'); $('#main').css('border', 'solid ' + border_size + 'px #00e'); $('#your_color').val(localStorage.getItem('bgcolor')); $('#your_font').val(font_size); $('#your_border').val(border_size); } else { setDefaults(); } } $(document).ready(function() { useSettings() });
The function sets also styles for values for input elements. At the end we called this function automatically.
And that’s all! On-line demo here:
http://directcode.eu/samples/web-storage-demo-ui-app-full.html
and the whole example also on GitHub:
https://github.com/dominik-w/js_html5_com/blob/master/web-storage-demo-ui-app-full.html
Summary
Today’s example has been tested in solid browsers (FF, Chrome) and works very well. It is quite interesting approach to personalized web application development. Thank you, localStorage.
Have fun!