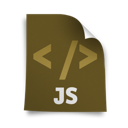
After discussing the absolute basics, it’s time to talk about arrays and strings in JavaScript. These are really important elements, and having mastered them we are ready to go to the next level.
Arrays and strings in JavaScript
At first we’ll take a look at text strings and basic methods of processing them. Afterwards we’ll discuss the arrays.
Personally I really like coding something related with strings and/or arrays.
The text is supported by the String class (type). This class allows us to create objects that contain strings; chars and subtitles. Also provides a lot of methods to operate on these strings:
anchor | replace |
big | search |
blink | slice |
bold | small |
charAt | split |
charCodeAt | strike |
concat | sub |
fixed | substr |
fontcolor | substring |
fontsize | sup |
indexOf | toLowerCase |
italics | toSource |
lastIndexOf | toUpperCase |
link | toString |
match | valueOf |
Example — working with strings:
var str = new String("Hi JavaScript!"); document.writeln("Our string: " + str.italics() + " has " + str.length + "chars length");
Using the dot operator we call the method of the String class, associated with our object (string).
The + operator is used to connect the strings. Of course in the context of String type. For the numbers it’s just operation of summation.
Example — split the string by a separator:
var tab = "ab-cd-ef-gh".split("-"); for (index in tab) { document.write(tab[index] + "<br />"); }
For example, such effect in PHP is reachable by the explode() function.
Example — operations on a string (then anchor method):
var str = new String("abc"); // puts str to the <a> markup: // <a name="myname">abc</a> var str = "abc".anchor("myname");
Methods for processing strings
Below we described frequently used methods of the String class:
– charAt — returns the character located within the index specified by the argument:
var c = "abc".charAt(1);
– charCodeAt — returns the character code:
var code = "abc".charCodeAt(1);
– concat — string concatenation:
var str1 = "A"; var str2 = "B"; var str3 = "123".concat(str1, str2); // result: 123AB
– fromCharCode — returns a string made of the characters whose numeric codes were passed as arguments:
var str = String.fromCharCode(65, 66, 67);
– indexOf — returns the index (position) of a character or substring; the second argument determines the starting point (index), if not specified then the default value (0) is used:
var index = "abcabcabc".indexOf("bc");
– lastIndexOf — the same as indexOf, but string is searched from the end:
var index = "abcabcabc".lastIndexOf("bc"); // result: 7
– replace — replacing part of the string:
var str = "John has a cat. John also knows JavaScript."; var str2 = str.replace(/John/gi, "Ben"); // regular expression
In this case we swapped John with Ben.
– search — checks whether a string described by the regular expression (regExp variable) occurs within a string:
myString.search(regExp);
– slice — returns a slice of the string, first parameter is the point of start, the second (optional) — the stopping point:
var str1 = "abcdefg".slice(3); var str2 = "abcdefg".slice(3, 5);
– valueOf — returns a basic value stored by an object. In case of text string it’s the same as string.toString(), and returns the string contained in the object.
More about regular expressions we will write in next, more detailed article.
Arrays in JavaScript
To create and operate on arrays there is a “class” named Array.
Methods of the Array class:
concat | slice |
join | sort |
pop | splice |
push | toString |
reverse | unshift |
shift | valueOf |
Example — increase the length of an array (indirect):
var tab = new Array(); tab[100] = 1;
Example — using the join() method:
var str = tab.join(',');
The join() method will return a string built from the array elements separated by separator (here: the comma).
The same as for strings, we can use the length property, which informs us about the number of elements in our array:
var tab = new Array(); tab.push(1,2); alert(tab.length);
Example — array sorting:
var tab = new Array('PHP', 'Ada', 'JavaScript', 'Ruby'); tab.sort(); alert(tab.join()); // Ada,JavaScript,PHP,Ruby
This is obviously a very simple example of sorting.
The definition of arrays — may be done in different ways:
var t = new Array("a","b","c");
can be written in a concise form:
var t = ["a","b","c"];
We can easily add and remove items to the array (an object of the Array class). For this we have push() and pop() methods.
// add element(s) at the end of an array: tab.push(element1[, element2, …, elementN]); // return the last element tab.pop();
– splice — removes elements from an array (new ones can be inserted in their place):
// removing elements splice(index, howMuch, [element1][, …, elementN]);
Example:
var tab1 = new Array("a", "b", 0, 0 , 0, "f", "g"); // will remove 3 elements, starting from index of 2 // and will put new elements (c, d, e) instead: tab1.splice(2, 3, "c", "d", "e");
The Array class also contains the shift() and unshift() methods, which are similar to push() and pop(). The main difference is that they operate at the beginning of array, not at the end.
Generally elements of an array can be removed through:
– splice method — the result will be a new array, without unnecessary elements:
// removes 5 elements var newTab = tab.splice(1, 5);
– delete operator:
delete tab[index];
Additional examples of work with the Array class you can find here: w3schools.
2-D arrays in JavaScript
In JavaScript, we can also use multidimensional arrays. We show how this can be done.
Example — 2D array — matrix:
str = "Matrix "; var a = new Array(4); // crating new array with N elements for (i = 0; i < 4; i++) { a[i] = new Array(4); for (j = 0; j < 4; j++) { a[i][j] = "[" + i + ", " + j + "]"; } } // processing and legibly printing of 2D array for (i = 0; i < 4; i++) { s = "Row " + i + ":"; for (j = 0; j < 4; j++) { s += a[i][j]; } str += s + " | "; } alert(str);
Another way to define a multidimensional array in JavaScript:
var matrix = [ [0, 1, 2], [3, 4, 5], [6, 7, 8] ]; alert(matrix[2][1]); // 7
And with this example we finish a brief statement of arrays and strings in JavaScript, thus discussing basics of the language.
Summary
We already have a solid base to explore more serious and interesting issues of the JS language.
Constructive comments are welcome.
In the next article we will work with DOM (Document Object Model) in JavaScript. We won’t forget also about BOM (Browser Object Model).
Thank you.