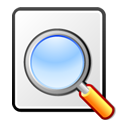
It’s time to take a step forward. We explore DOM and BOM (Browser Object Model, don’t confuse with Byte Order Mark).
JavaScript DOM
We will meet with DOM many times. The topic is extensive, but definitely it’s one of the most interesting things in JavaScript.
Moreover, thanks to the operations of the DOM we have big possibilities at the level of our code.
DOM (Document Object Model) — presents the document (current page) so that JavaScript sees document as an object. DOM is a set of methods and fields that allow you to add, delete, find and change the items.
The document object — collections:
anchors[] | forms[] |
images[] | links[] |
The document object — properties:
cookie | documentMode |
domain | lastModified |
readyState | referrer |
title | URL |
The document object — methods:
close() | getElementById() |
getElementsByName() | getElementsByTagName() |
open() | write() |
writeln() |
BOM (Browser Object Model) — it’s much less common term than DOM. In this sense we have objects related with the browser window.
So DOM refers to the document in browser window, and BOM refers to the browser (the window object in JavaScript).
JavaScript — window object properties:
closed | outerWidth |
defaultStatus | pageXOffset |
document | pageYOffset |
frames | parent |
history | screen |
innerHeight | screenLeft |
innerWidth | screenTop |
length | screenX |
location | screenY |
name | self |
navigator | status |
opener | top |
outerHeight |
JavaScript — window object methods:
alert() | open() |
blur() | print() |
clearInterval() | prompt() |
clearTimeout() | resizeBy() |
close() | resizeTo() |
confirm() | scroll() |
createPopup() | scrollBy() |
focus() | scrollTo() |
moveBy() | setInterval() |
moveTo() | setTimeout() |
DOM — methods
Main methods for working with DOM:
– getElementById(‘foo’) — returns a reference to the element by its ID
– getElementsByName(‘foo’) — returns a list of elements with a given name in the document
– getElementsByTagName(‘foo’) — returns a HTMLCollection of elements with the given tag name
– getElementsByClassName(‘foo’) — elements having specified CSS class
– write() — displays the text
– open() — opens a data stream in a browser window
– close() — finishes writing to a document, opened with document.open()
Example — simple operations on DOM:
<button id="btn" onclick="turnOff()" class="foo">Test</button>
function turnOff() { document.getElementById("btn").disabled = true; }
The disable parameter will be set to true for the button with ‘btn’ ID.
Accessing to properties:
<p id="par">Text…</p>
var par = document.getElementById("par"); // get value of style: display var view = par.style.display; // another way var view = par.style['display']; alert(view);
Attributes
Elements have attributes, and we can operate on them via JavaScript.
We read attributes using the getAttribute(name) method; to set a value for attribute we use setAttribute(name, value).
To check if the attribute exists, just use the hasAttribute(name) method.
Example — attributes:
<a href="http://google.com/" rel="google" id="link1">Google</a>
anchor = document.getElementById('link1'); var anchorRel = anchor.getAttribute('rel'); // returns value of rel attribute alert(anchorRel);
Example — checking attributes:
if (anchor.hasAttribute('class')) { var anchorClassName = anchor.getAttribute('class'); // if attribute 'class' exists, will return its value alert(anchorClassName); } // overwrite the value of rel anchor.setAttribute("rel", "new value"); // will create new attribute (lang) with value (en) anchor.setAttribute("lang", "en");
The getAttribute() method returns null if attribute doesn’t exist. However, to remove the attributes use the removeAttribute(name) method instead of setAttribute(name, null).
Example — set the style attribute:
// hide the paragraph element par.setAttribute("style", "display: none");
Example — remove attribute:
// remove the appearance of a paragraph, set previously in CSS par.removeAttribute("style");
The next example demonstrates even more interesting possibility — extending content. We will meet with this sometimes when coding (e.g. using AJAX).
Example — adding dynamic content using DOM in JavaScript:
// create a new element: TextNode var new_elem = document.createElement("p"); var elem_text = document.createTextNode("New one"); new_par.appendChild(elem_text); document.body.appendChild(new_elem);
The appendChild method adds an element to the parent element. Instead of appendChild, we can also insert an element before another element using code like below:
var old_elem = document.getElementById("par"); // insert before element insertBefore(new_elem, old_elem);
Deleting an item:
// remove the first paragraph in the collection var op = document.body.getElementsByTagName("p")[0]; document.body.removeChild(op);
Replace:
var new_elem = document.createElement("p"); var elem_text = document.createTextNode("Dynamic text …"); new_elem.appendChild(elem_text); var old_elem = document.body.getElementsByTagName("p")[0]; old_elem.parentNode.replaceChild(new_elem, old_elem);
Nodes
In short: ‘node’ in the context of JavaScript DOM is a single element of the document. Such element may have a parent, and himself may be the parent of other elements.
Following picture presents this idea:
Main properties of the JavaScript DOM nodes
– firstChild — the first node in the element
– lastChild — the last node in the element
– previousSibling — neighboring node before this element (“siblings before”)
– nextSibling — neighboring node for this element (“siblings after”)
– parentNode — parent of element
– childNodes — collection of all nodes contained in this element (“all children” list)
And furthermore:
– attributes — list of attributes for the element
– nodeName — element’s name (current node)
– nodeValue — value (only for text items)
– nodeType — shows the type of the given element node
– ownerDocument — the document containing the element
Example:
if (element.nextSibling && element.nextSibling.nodeType == 1) alert('Neighboring node is an element')
Example — remove item:
// the element will "remove himself" element.parentNode.removeChild(element);
Getting parent element:
// sample result: tr for the td element var a = document.getElementById('foo').parentNode.nodeName);
Events
I will say now a few words about the events, and about functions for detecting and handling events (event listeners).
On the elements you may have a number of events, such as hovering or mouse clicking. We can program the code to detect and handle these events when they become.
Example — adding event listener to a button:
button = document.getElementById("btn"); // in real browsers (FF, Chrome, Safari, …) button.addEventListener("click", eventHandler, false); // in IE button.attachEvent("onclick", eventHandler);
Removing event listener:
// IE detachEvent(); // normal browsers removeEventListener();
Summary
By touching events in JavaScript, we end the discussion of the basics of DOM and BOM. With these issues we will meet in further articles and development tasks.