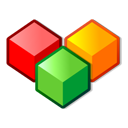
Welcome to the first, but certainly not the last topic of this type, which touches interesting features of JavaScript, tricks and less common structures, like the with instruction.
Interesting issues and good practices in JavaScript
For a good start: Tip #1 — the ‘with’ instruction
The with instruction, being a part of JavaScript language, allow us to determine the object, to which we will refer inside a “body” defined for this instruction. This can greatly simplify the code.
This is illustrated by the following example:
var a, b, c; var r = 5; with (Math) { // here by default are called methods and properties // of the Math object a = PI * r * r; b = r * cos(PI); c = r * sin(PI / 2); } alert(a + " - " + b + " - " + c);
Thus, in this example we don’t need to specify name of the Math object, each time a property or method of the object is called. For example sin() instead of Math.sin().
Tip #2 — escape and unescape functions
These functions encodes and decodes string values:
– escape — returns the hexadecimal encoding of an argument for special characters; in other words encodes the string to a portable form, that can be freely transmitted through the network,
– unescape — returns the string as ASCII; decodes the values encoded by the escape function.
These functions are often used on server side. JavaScript encodes and decodes names / value pairs in the URL.
var str = 'JS? Go to https://javascript-html5-tutorial.com/'; alert(escape(str)); // JS%3F+Go+to+http%3A%2F%2Fjavascript-html5-tutorial.com%2F
Tip #3 — Fast testing
With the help of logical operators, we can perform a quick test expressions:
– false && some_expression is an acronym for executing the false expression;
– true || some_expression is an acronym for executing the true expression;
For example:
var x = 1; if (false && x > 0) alert('Debug: 0'); if (true || x > 0) alert('Debug: 1'); // -> Debug: 1
Tip #4 — select the text box
So it’s a simple implementation of the ‘Select all’ function. In this case for the textarea field.
<form> <textarea name="text" cols="30" rows="2">Here is the text </textarea> <input onclick="java_script:this.form.text.focus(); this.form.text.select();" type="button" value="Select"> </form>
Tip #5 — argument mapping
An example of the mapping function. In this example we are passing as arguments a function and an array, which will be processed by this function.
function map(f, a) { var result = new Array; for (var i = 0; i != a.length; i++) result[i] = f(a[i]); return result; } // returns [0, 1, 8, 125, 1000] map(function(x) { return x * x * x }, [0, 1, 2, 5, 10];
Tip #6 — the ‘debugger’ instruction
We can see this simple instruction in action, having active debug tool in our browser, e.g. Firefox + Firebug. In practice, it may be really useful.
var x = 20, y = 40; var dt = x + y; debugger; // moves into the debugger
Tip #7 — is HTML element visible / hidden ?
This example will be well known for people familiar with DOM.
We’ll get style: display value of our element.
<button id="btn">My button</button>
function isElemHidden(el) { if (document.getElementById(el).style.display == "none") { return true; } return false; } if (isElemHidden('btn')) { alert('Yes'); } else { alert('No'); }
This is obviously a very simple solution in pure JavaScript, without the use of frameworks.
Tip #8 — the use of alternative functions
We can say it’s function alternative through adding a property. The details will be obvious when we’ll get deeper in JavaScript OOP. For the moment let’s consider simple example:
function sayHi() { alert("Hi"); } sayHi.alternative = function() { alert("Hello, hello!"); }; // call the function sayHi(); // displays "Hi" // call alternative sayHi.alternative(); // displays "Hello, hello!"
We hope that these few tips can encourage readers to explore the secrets of JavaScript. A language has a lot of interesting secrets, even if some were unintentional 🙂
People
And at the end, let me pay tribute to people who have had a great impact on JavaScript and the modern frontend development:
– Brendan Eich — American programmer, creator of JavaScript, today — CTO in Mozilla Corporation,
– Douglas Crockford — the next guru, software developer having a big impact on JavaScript, he defined and popularized JSON, we owe him other tools like JSMin or JSLint,
– John Resig — founder and leader of the jQuery project.
The next article will be about bitwise operators.
Thank you for your attention.