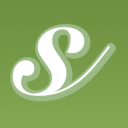
The script.aculo.us library
Delving into the JavaScript frameworks, we would like to present the essence of script.aculo.us library.
As we mentioned in JS Frameworks introduction, Prototype JS and script.aculo.us libraries are often seen together.
The library, which now referred to, is like an extension of Prototype JS of visual effects, animation, Drag and Drop, creating widgets, tools for working with DOM, or Ajax controls. Functions of this library are easy to use, and of course there are cross-browser.
Working with script.aculo.us library
At the beginning we add Prototype JS and script.aculo.us to the document. Let’s start with a few words about how to create dynamic elements.
Component that supports the creation of DOM elements is the Builder, fulfilling the function of pattern.
Example — using the Builder component:
var tbl = Builder.node("tbl", { border: 0, width: 200 }, [ Builder.node( "tr", [ Builder.node("td", {width: 100}, "column 1" ), Builder.node("td", {width: 100}, "column 2" ), Builder.node("td", {width: 100}, "column 3" ) ]) ]); $('mydiv').appendChild(tbl);
Example #2:
var element = Builder.node('div', { id: 'ghosttrain' }, [ Builder.node('div', { className: 'controls', style: 'font-size:11px;' }, [ Builder.node('h1', 'Ghost Train'), 'testtext', 2, 3, 4, Builder.node('ul', [ Builder.node('li', { className: 'active', onclick: 'test()' }, 'Record') ]), ]), ]);
Drag&drop support
Script.aculo.us allows us to quickly implement Drag and Drop, and add eye-catching animations.
The library provides for this purpose following classes:
– Draggable — defines an object that we will drag,
– Droppables — allows to design an appropriate response for dropping the item.
The Draggable class
That means elements, which can be dragged.
Example — Draggeble test:
var test = Builder.build("<span>Displacement</span>"); document.getElementsByTagName("body")[0].appendChild(test); new Draggable(test); new Draggable('id_of_element', [options]);
More details about this class you can find here.
Example #2 — Draggable — settings:
new Draggable('mydiv', { constraint: 'horizontal', handle: 'handle' }); var mydrag = new Draggable('dv1', { revert: true }); mydrag.destroy(); // assignment back // new Draggable('dd1', { revert: true }); new Draggable('dd1', { revert: true, snap: [40, 40] }); new Draggable('dd2', { scroll: window });
The Droppables class
So elements that can be dropped.
Example — using Droppables:
Droppables.add('id_of_element',[options]); Droppables.add('shopping_cart', { accept: 'products', onDrop: function(element) { $('shopping_cart_text'). update('Dropped the ' + element.alt + ' on me.'); } }); Droppables.remove(element); // removing
Example — all the elements of the list as Draggable:
$$('container li').each( function(li) { new Draggable(li); });
And now we can drag items of the list.
Example — implementation of drag&drop:
HTML structure
<ul id="container"> <li id="item_1"><span>@</span> Lorem</li> <li id="item_2"><span>@</span> Ipsum</li> <li id="item_3"><span>@</span> Dolor</li> </ul>
CSS
#container .handle { background-color: #090; color: #fff; font-weight: bold; padding: 3px; cursor: move; }
JavaScript code:
$('container').select('li').each( function(li) { new Draggable(li, { handle: 'handle' }); });
Another example is the use of droppables (items “dropped”).
Example — Droppables
HTML:
<ul id="drop_zone"></ul>
CSS:
#container, #drop_zone { width: 200px; height: 300px; list-style-type: none; margin-left: 0; margin-right: 20px; float: left; padding: 0; border: 2px dashed #999; }
JavaScript:
document.observe("dom:loaded", function() { $('container').select('li').each( function(li) { new Draggable(li); }); Droppables.add('drop_zone'); });
Now, before talking about components, a little mention about the effects and control of elements.
Example — controlling elements:
<div onclick="$(this).switchOff()">Click to hide</div> <!-- or with the effect --> <div onclick="$(this).blindUp({ duration: 1 })">Click</div>
Methods such as blindUp() perform the task in a way, accompanied by effects (animations). We will deal with this in part 2.
Autocompleter (Ajax) component
Implementation of solution like Ajax suggest — auto-complete text-box suggestions.
The call looks like this:
new Ajax.Autocompleter(id_of_text_field, id_of_div_to_populate,
url, options);
Example — use of Ajax.Autocompleter:
In the HTML structure we create new text field, with ID = “autocomplete”, and also an element (e.g. DIV) with ID = “autocomplete_choices”.
Then we add JS code:
new Ajax.Autocompleter("autocomplete", "autocomplete_choices", "/url/on/serv/bar.php", { paramName: "value", minChars: 2, indicator: 'indicator1' });
The bar.php file on the server side returns the results as a list — the simplest example below:
echo "<ul><li>Data1</li><li>Data2</li></ul>";
The alternative is another element of script.aculo.us library.
Autocompleter.Local — is a local data storage element, e.g. a table, without the need of the data returned from server-side.
Usage of this Autocompleter.Local looks as follows:
new Autocompleter.Local('id_text_field', 'id_div_to_populate', 'array_of_strings', options);
For example:
var array_of_strings = [ 'AB', 'CD', 'EF', // … itd ]; // we set input_id (ID of the field) // warning: set autocomplete="off" for this field new Autocompleter.Local('input_id', 'mydiv', bandsList, { });
Finally, I will discuss InPlace Editor — my favorite element of this library. Some time ago (“the Web 2.0 boom”) we used this almost all the time in projects.
InPlace Editor
Allows user to edit an item in the implementation of the site, and change the value without reloading the page. This is useful functionality in many Web applications based on Ajax.
The constructor:
new Ajax.InPlaceEditor(element, url, { options });
Example — using InPlaceEditor:
<p id="editme">Click me </p>
new Ajax.InPlaceEditor('editme', '/demoajax.php');
We obtain an editable paragraph ‘Click me!’, which goes into edit mode after clicking. Data can be sent to the PHP script, where further operations can be done.
There are a lot of details, which are fully described in the documentation InPlace Editor and InPlaceCollection Editor.
Summary
That’s all in the 1st part of the basic script.aculo.us course. I invite you to the next part, which will be focused on visual effects.