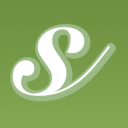
The 2nd part of the script.aculo.us library course. Today about its strongest point — visual effects.
Getting to know more possibilities of script.aculo.us
Visual effects
Effects have their own options, but everywhere we can use Core Effect properties.
The general form:
new Effect.EffectName(element, required-params, [options]);
Let’s see how it looks in practice.
Example — use effects:
<div id="morph_demo" style="background: #ccc; width: 100px; height: 100px;"></div> <ul> <li> <a href="#" onclick="$('morph_demo').morph('background: #00ff00; width: 300px;'); return false;"> Click … </a> </li> <li> <a href="#" onclick="$('morph_demo').morph('background: #cccccc; width: 100px;'); return false;"> Reset </a> </li> </ul>
Example #2 — determination of the effect parameters:
HTML
<div id="our_div" style="background: #eee; width: 100px; height: 100px; border: solid 2px red;"></div>
JavaScript
new Effect.Opacity('our_div', { duration: 2.0, transition: Effect.Transitions.linear, from: 1.0, to: 0.2 });
The above code defines the effect of changing the transparency for the our_div element.
Common callback functions
These are:
– beforeStart()
– beforeSetup()
– afterSetup()
– beforeUpdate()
– afterUpdate()
– afterFinish()
Effects: properties and methods
For the Effect object instances, there are a number of useful properties and methods, such as:
– effect.element — element to which the effect is applied
– effect.options — contains options defined for effect
– effect.currentFrame — the last rendered frame number
– effect.startOn, effect.finishOn — determine the time (ms) when the effect will be started / stopped
– effect.effects[] — an array containing the definitions of the individual effects (for Effect.Parallel)
– effect.cancel() — stopping the effect
– effect.inspect() — returns basic information about the instance (debug)
Example — simple animation:
new Effect.Move(this, { x: 200, transition: Effect.Transitions.spring });
Effect queues
This is an excellent possibility offered by the library, allowing us to queue and perform many effects as a sequence.
Example — queue effects:
new Effect.SlideDown('test1'); new Effect.SlideUp('test1', { queue: 'end' }); // 2 new Effect.SlideUp('test1', { queue: 'end' }); new Effect.SlideDown('test1', { queue: 'front' }); // 3 new Effect.SlideUp('menu', { queue: { position: 'end', scope: 'menuxscope' } }); new Effect.SlideUp('bannerbig', { queue: { position: 'end', scope: 'bannerscope' } }); new Effect.SlideDown('menu', { queue: { position: 'end', scope: 'menuxscope' } });
Effects in examples
Example — scope:
var queue = Effect.Queues.get('myscope'); var queue = Effect.Queues.get('myscope'); queue.each(function(effect) { effect.cancel(); }); Effect.Queues.get('myscope').interval = 100;
Example — Effect.Appear — show the element (with the effect):
$('id_of_element').appear(); // or $('id_of_element').appear({ duration: 3.0 }); // Effect.Appear('id_of_element', { duration: 3.0 });
Element appears, optionally with defined effect.
Options:
duration (default 1.0), from (default 0.0), to (1.0)
Next methods (Blind-) causes the appearance (or disappearance) of the element, with the animation (winding / unwinding in a certain direction).
An example of Effect.BlindDown
Effect.BlindDown('id_of_element', { duration: 3.0 });
Options:
scaleX, scaleY, scaleContent, scaleFromCenter, scaleMode, scaleFrom, scaleTo, duration.
Effect.BlindRight
Effect.BlindRight(‘id_of_element’);
Practical example — blind right:
Effect.BlindRight = function(element) { element = $(element); var elementDimensions = element.getDimensions(); return new Effect.Scale(element, 100, Object.extend({ scaleContent: false, scaleY: false, scaleFrom: 0, scaleMode: { originalHeight: elementDimensions.height, originalWidth: elementDimensions.width}, restoreAfterFinish: true, afterSetup: function(effect) { effect.element.makeClipping().setStyle({ width: '0px', height: effect.dims[0] + 'px' }).show(); }, afterFinishInternal: function(effect) { effect.element.undoClipping(); } }, arguments[1] || { })); };
Example — Effect.BlindUp:
Effect.BlindLeft = function(element) { element = $(element); element.makeClipping(); return new Effect.Scale(element, 0, Object.extend({ scaleContent: false, scaleY: false, scaleMode: 'box', scaleContent: false, restoreAfterFinish: true, afterSetup: function(effect) { effect.element.makeClipping().setStyle({ height: effect.dims[0] + 'px' }).show(); }, afterFinishInternal: function(effect) { effect.element.hide().undoClipping(); } }, arguments[1] || { }) ); };
Effect.DropOut
Effect.DropOut('id_of_element');
// Effect.DropOut — element opacity
$(‘id_of_element’).fade({ duration: 3.0, from: 0, to: 1 });
Opcje:
duration, form, to
Effect.Fold — “folding” of an element
Effect.Fold('id_of_element');
Effect.Grow — “growing” element
Effect.Grow(‘id_of_element’);
Options:
direction (default = ‘center’, inne: ‘top-left’, ‘top-right’, ‘bottom-left’, ‘bottom-right’), duration
Effect.Highlight — highlighting
new Effect.Highlight('id_of_element', [options]);
Options:
startcolor — starting color; the default is #ffff99
endcolor — ending color; #ffffff
restorecolor — the background color of the item after the effect call
Example of using the effect of highlighting:
<p id="highlight_demo" style="padding:10px; border:1px solid #ccc; background:#ffffff;"> Demo. Click!<br /> <a href="#" onclick="new Effect.Highlight(this.parentNode, { startcolor: '#ffff99', endcolor: '#ffffff' }); return false;"> Highlight me! </a> </p>
Effect.Morph — change the CSS properties of an element (aculo v. 1.7+)
Example:
new Effect.Morph('error_message', { style: 'background: #f00; color: #fff;', // CSS duration: 0.8 // Core Effect properties });
Effect.Move — moving element:
new Effect.Move('object', { x: 0, y: 0, mode: 'absolute' });
Options:
x, y, mode (absolute, relative)
Effect.Puff — “explosion” effect
Effect.Puff('id_of_element', { duration: 3 });
Options:
duration, from, to
For example:
<div id="puffd" style="width: 80px; height: 80px; background: #c2defb; border: 1px solid #333;"></div> <ul> <li> <a href="#" onclick="new Effect.Puff('puffd'); return false;">Click</a> </li> <li> <a href="#" onclick="$('puffd').setStyle({ display: 'block', opacity: 1, width: '80px', height: '80px' }); return false;">Reset</a> </li> </ul>
Effect.Pulsate — pulsation:
Effect.Pulsate('id_of_element', { pulses: 5, duration: 1.5 });
Options:
duration, from, pulses
For example:
<div id="pulsate_demo" style="width: 150px; height: 40px; background: #ccc; text-align: center;"> <a href="#" onclick="Effect.Pulsate('pulsate_demo'); return false;" style="line-height: 40px;">Click!</a> </div>
Effect.Scale — scaling:
new Effect.Scale(element, percent, [options]);
Example:
<a href='#' onclick="new Effect.Scale(this.parentNode, 200); return false;">Click</a>
Effect.ScrollTo — a nice looking effect of scrolling the page to a specified location:
Effect.ScrollTo('id_of_element');
Options:
duration, offset (px)
Example:
<p><a name="article_top" id="article_top"></a></p> … <a href="#" onclick="Effect.ScrollTo('article_top'); return false;">Click </a>
Effect.Shake — shaking:
Effect.Shake('id_of_element');
Options:
duration, distance
Example:
<div id="shake_demo" style="width:150px; height:40px; background: #ccc; text-align: center;> <a href="#" onclick="new Effect.Shake('shake_demo'); return false;" style="line-height: 40px;">Click </a> </div>
Effect.Shrink — shrinkage of the element:
Effect.Shrink('id_of_element');
Options:
direction, duration
Effect.SlideDown — expand:
Effect.SlideDown('id_of_element', { duration: 3.0 });
Effect.SlideUp — collapse:
Effect.Squish — disappearance of the left and top:
Effect.Squish('id_of_element');
Effect.SwitchOff — the “off” effect:
Effect.SwitchOff('id_of_element');
The Slider component
Programmer-friendly GUI component implementation: the slider.
A constructor:
new Control.Slider('handles','track', [options]);
Example:
div.slider { width: 256px; margin: 10px 0; background-color: #ccc; height: 10px; position: relative; } div.slider div.handle { width: 10px; height: 15px; background-color: #f00; cursor: move; position: absolute; } div#zoom_element { width: 50px; height: 50px; background: #2d86bd; position:relative; }
HTML <div> Use the slider to resize the box <div id="zoom_slider"> <div></div> </div> <p>And this to change its color</p> <div id="rgb_slider"> <div style="background-color: #f00;"></div> <div style="background-color: #0f0;"></div> <div style="background-color: #00f;"></div> </div> <div id="zoom_element"></div> </div>
JavaScript
(function() { var zoom_slider = $('zoom_slider'), rgb_slider = $('rgb_slider'), box = $('zoom_element'); new Control.Slider(zoom_slider.down('.handle'), zoom_slider, { range: $R(40, 160), sliderValue: 50, onSlide: function(value) { box.setStyle({ width: value + 'px', height: value + 'px' }); }, onChange: function(value) { box.setStyle({ width: value + 'px', height: value + 'px' }); } }); new Control.Slider(rgb_slider.select('.handle'), rgb_slider, { range: $R(0, 255), sliderValue: [45, 134, 189], onSlide: function(values) { box.setStyle({ backgroundColor: "rgb("+ values.map(Math.round).join(',') +")" }); }, onChange: function(values) { box.setStyle({ backgroundColor: "rgb("+ values. map(Math.round).join(',') +")" }); } }); })();
Final example — a simple widget:
var Widget = Class.create({ initialize: function(element, options) { this.element = $(element); // fire an event and pass this instance as a property // of the event object this.element.fire("widget:created", { widget: this }); } }); // using the custom event $('some_element').observe('widget:created', function() { console.log("widget created"); }); // or observe document-wide: document.observe("widget:created", function() { console.log("widget created"); }); var someWidget = new Widget('some_element');
Summary
As we have seen, this great library allows us to create excellent results with quite small amount of code. Only our imagination can limit us, and sometimes knowledge of details, which however, can be quickly found in the official documentation.
Have fun with script.aculo.us!