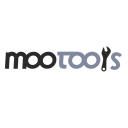
MooTools is another library, worthy of attention. It can also expand our view on the JavaScript language itself.
“My Object-Oriented Tools” — MooTools
It is modular (in construction) JavaScript library. The foundation is called Core.
Other libraries are optional. MooTools consists of a plurality of modules. Such design allows users to retrieve only the parts of the library, which they intend to use.
The implement method
Its goal is to extend the functionality of our native objects.
Example — implement method:
var Person = new Class({ initialize: function(firstname) { this.firstname = firstname; } }); Person.implement({ sayHi: function() { alert('Hi ' + this.firstname); } });
We can extend also in bulk:
Native.implement([obj1, obj2, obj3], { method1: function() { // (…) }, method2: function() { // (…) } });
More about the Core
It offers many possibilities:
$chk() — checking objects:
function myFunction(arg) { if ($chk(arg)) alert('The object exists or is 0.'); else alert('The object is either null/undefined/false'); }
$clear() — cleans timeout / interval:
// execute myFunction in 5 sec var myTimer = myFunction.delay(5000); myTimer = $clear(myTimer); // cancels myFunction
$defined(obj) — checks whether the value is defined, for example:
$defined(document.body); // true
$arguments() — creates a function that returns the argument depending on the index:
var secondArgument = $arguments(1); alert(secondArgument('a','b','c')); // "b"
$empty() — empty function (mockup):
var myFunc = $empty;
$lambda() — creates an empty function that returns the passed value:
var returnTrue = $lambda(true); // prevents a link Element from being clickable myLink.addEvent('click', $lambda(false));
It is outdated method. Currently recommended to use Function.from.
$extend(original, extension) — extending objects, where:
– original — is an original object,
– extension — object whose properties are copied to the original.
$each() — iterator (on each item):
$each(iterable, fn[, bind]);
Example — iterating with $each:
// array $each(['Sun','Mon','Tue'], function(day, index) { alert('name:' + day + ', index: ' + index); }); // "name: Sun, index: 0", "name: Mon, index: 1", etc
$random() — random number:
var random = $random(min, max);
$splat() — converts the argument to an array, for example:
$splat('hello'); // ['hello']. $splat(['a', 'b', 'c']); // ['a', 'b', 'c'].
Currently instead of $splat, the use of Array.from is recommended.
$time() — returns the current time stamp:
var time = $time(); // we recommend, however, use just: // var time = Date.now();
$try — attempt to perform a number of functions:
$try(fn[, fn, fn, fn, …]);
Browser
This is an object that provides methods for detecting browsers and platforms (the content from the original documentation).
Features
– Browser.Features.xpath — (boolean) True if the browser supports DOM queries using XPath
– Browser.Features.xhr — (boolean) True if the browser supports native XMLHTTP object.
Engine
– Browser.Engine.trident — (boolean) True if the current browser uses the trident engine (e.g. IE).
– Browser.Engine.gecko — (boolean) True if the current browser uses the gecko engine (e.g. FF, Moz).
– Browser.Engine.webkit — (boolean) True if the current browser uses the webkit engine (e.g. Safari, Google Chrome, Konqueror).
– Browser.Engine.presto — (boolean) True if the current browser uses the presto engine (e.g. Opera 9).
– Browser.Engine.name — (string) The name of the engine.
– Browser.Engine.version — (number) The version of the engine. (e.g. 950)
– Browser.Plugins.Flash.version — (number) The major version of the flash plugin.
– Browser.Plugins.Flash.build — (number) The build version of the flash plugin installed.
Platform
– Browser.Platform.mac — (boolean) True if the platform is Mac.
– Browser.Platform.win — (boolean) True if the platform is Windows.
– Browser.Platform.linux — (boolean) True if the platform is Linux.
– Browser.Platform.ipod — (boolean) True if the platform is an iPod touch / iPhone.
– Browser.Platform.other — (boolean) True if the platform is neither Mac, Windows, Linux nor iPod.
– Browser.Platform.name — (string) The name of the platform.
Let’s move on to practical applications of what the MooTools framework offers.
Operations on arrays — examples
Specific examples with the commentary:
// filter for a given condition var biggerThanTwenty = [10, 3, 25, 100]. filter(function(item, index) { return item > 20; }); // biggerThanTwenty = [25, 100] // merging var animals = ['Cow', 'Pig', 'Dog', 'Cat']; var sounds = ['Moo', 'Oink', 'Woof', 'Miao']; sounds.associate(animals); // {'Cow': 'Moo', 'Pig': 'Oink', 'Dog': 'Woof', 'Cat': 'Miao'} // flattening - convert to the 1-D array var myArray = [1,2,3,[4,5, [6,7]], [[[8]]]]; var newArray = myArray.flatten(); // newArray = [1,2,3,4,5,6,7,8] // conversions - something for web developers ['11','22','33'].hexToRgb(); // "rgb(17,34,51)" ['11','22','33'].hexToRgb(true); // [17, 34, 51] [17,34,51].rgbToHex(); // "#112233" [17,34,51].rgbToHex(true); // ['11','22','33'] [17,34,51,0].rgbToHex(); // "transparent"
The $A function — constructing arrays:
var anArray = [0, 1, 2, 3, 4]; var copiedArray = $A(anArray); // [0, 1, 2, 3, 4].
String
Just like Prototype JS, MooTools also supports strings.
Examples:
// cleaning up -> "i like cookies" " i like cookies \n\n".clean(); // camel-case -> "ILikeCookies" "I-like-cookies".camelCase(); // separation -> "I-like-cookies" "ILikeCookies".hyphenate(); // test of regexp "I like cookies".test("COOKIE", "i"); // RegExp escape -> 'animals\.sheep\[1\]' 'animals.sheep[1]'.escapeRegExp();
Let’s move on.
substitute() — this method interpolate elements; similar to the Template in Prototype JS.
Example — interpolation:
var myString = "{subject} is {property_1} and {property_2}."; var myObject = {subject: 'Jack Bauer', property_1: 'our lord', property_2: 'savior'}; // result: Jack Bauer is our lord and savior myString.substitute(myObject);
Hash (key-value)
MooTools, like Prototype JS, offers convenient handling of Hashes.
Constructor:
var myHash = new Hash([object]);
Example — work with Hash objects in MooTools:
var myHash = new Hash({ aProperty: true, aMethod: function() { return true; } }); alert(myHash.has('aMethod')); // true
It provides a number of methods, such as iteration using each():
hash.each(function(value, key) { alert(value); });
Metoda has() — it’s just checking, if the Hash has a given key, for example:
var hash = new Hash({'a': 'one', 'b': 'two', 'c': 'three'}); hash.has('a'); // returns true hash.has('d'); // returns false
And the same for values: hasValue:
var inHash = myHash.hasValue(value);
Extend
Typically for MooTools, we can extend the Hash:
var hash = new Hash({ 'name': 'John', 'lastName': 'Doe' }); var properties = { 'age': '20', 'sex': 'male', 'lastName': 'Fred' }; hash.extend(properties);
Thus we extended the Hash object by new properties.
Let’s take a look at the other available methods:
– myHash.erase(key); // erase the key
– myHash.get(key); // get the value of the key
– myHash.set(key, value); // set value for the key
– myHash.getKeys(); // get all keys
– myHash.getValues(); // get all values
– myHash.getLength(); // get size
– empty() — makes hash empty, e.g.:
var hash = new Hash({ 'name': 'John', 'lastName': 'Doe' }); hash.empty(); // hash is now an empty object: {}
– map() — mapping to Hash:
var timesTwo = new Hash({a: 1, b: 2, c: 3}). map(function(value, key) { return value * 2; }); // timesTwo now holds an object containing : {a: 2, b: 4, c: 6};
– clean() — clean the hash object completely:
var hash = new Hash({ 'name': 'John', 'lastName': 'Doe' }); hash = hash.getClean(); // hash ceased to be an object of Hash type hash.each() // error!
The next method allows us to create the HTTP request based on the hash. It’s myHash.toQueryString().
Example:
var myHash = new Hash({apple: "red", lemon: "yellow"}); myHash.toQueryString(); // "apple=red&lemon=yellow"
Event
So it’s about event handling in MooTools edition. In this context, the library also offers excellent support for the keyboard.
Constructor:
new Event([event[, win]]);
– event — (event) HTMLEvent object
– win — (window, optional) — the context of event
Example — event + keyboard — handling keys:
$('myLink').addEvent('keydown', function(event) { alert(event.key); // button pressed (lowercase) alert(event.shift); // returns true if pressed the Shift key if (event.key == 's' && event.control) alert('Document saved.'); // handling the Ctr+S combination // etc… });
Available properties:
– shift — (boolean) True if pressed the shift key
– control — (boolean) True if pressed the ctrl
– alt — (boolean) True if pressed the alt
– meta — (boolean) True jeśli “meta key”
– wheel — (number) the 3rd button (scroller)
– code — (number) key code
– page.x — (number) x-position of the mouse in relation to the whole window
– page.y — (number) y-position of the mouse in relation to the whole window
– client.x — (number) x-position of the mouse with respect to the viewport
– client.y — (number) y-position
– key — (string) pressed buttons as a lowercase string; it may be also: ‘enter’, ‘up’, ‘down’, ‘left’, ‘right’, ‘space’, ‘backspace’, ‘delete’, and ‘esc’
– target — (element) the target of event
– relatedTarget — (element) the target of event
Example:
myEvent.stop(); $('myAnchor').addEvent('click', function(event){ event.stop(); // 'this' is Element that fires the Event this.set('text', "Where do you think you're going?"); (function() { this.set('text', "Instead visit the Blog."). set('href', 'http://blog.mootools.net'); }).delay(500, this); }); myEvent.stopPropagation(); myEvent.preventDefault();
Event.keys:
Event.Keys.shift = 16; $('myInput').addEvent('keydown', function(event) { if (event.key == "shift") alert("You pressed shift."); });
Mouse
With the AddEvent method, we define an event handler function for the mouse.
function myFunction() { alert('Mouse…'); } $('myElement').addEvent('mouseenter', myFunction); $('myElement').addEvent('mouseleave', myFunction); $('myElement').addEvent('mousewheel', myFunction);
Summary
With these examples, about using mouse and keyboard in MooTools, we finish the 1st part of the course.