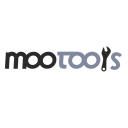
Here is the second, summarizing part of the course, the theme of which is the JavaScript MooTools library.
The MooTools library
Let’s start with the benefits of object-oriented programming (OOP) support, offered by MooTools.
“Class” and creating classes
Constructor:
var MyClass = new Class(properties);
where: properties (object) — is a collection of class properties.
These properties can also be incorporated by Extends and Implements methods.
Example — the Class construction:
var Cat = new Class({ initialize: function(name) { this.name = name; } }); var myCat = new Cat('Kitty'); alert(myCat.name); // 'Kitty'
This is equivalent to creating classes. Whereas using Extends, we get the equivalent of inheritance.
Example — using Extends:
var Animal = new Class({ initialize: function(age) { this.age = age; } }); var Cat = new Class({ Extends: Animal, initialize: function(name, age) { this.parent(age); // will call initalize of Animal this.name = name; } }); var myCat = new Cat('Kitty', 20); alert(myCat.name); // 'Kitty' alert(myCat.age); // 20
If someone has programmed in object-oriented languages, where interfaces were available, and wants to try it in JavaScript, may have a look at “Implementes” from MooTools library.
Example — working with Implements:
var Animal = new Class({ initialize: function(age) { this.age = age; } }); var Cat = new Class({ Implements: Animal, setName: function(name) { this.name = name; } }); var myAnimal = new Cat(20); myAnimal.setName('Kitty'); alert(myAnimal.name); // 'Kitty'
MooTools and inheritance
OOP support from MooTools helps to simulate inheritance, so ultimately useful in programming games, widgets, etc.
Let’s analyze the example:
var Human = new Class({ initialize: function(name, age) { this.name = name; this.age = age; }, isAlive: true, energy: 1, eat: function() { this.energy++; } }); var Ninja = new Class({ Extends: Human, initialize: function(side, name, age) { this.side = side; this.parent(name, age); }, energy: 100, attack: function(target) { this.energy = this.energy - 5; target.isAlive = false; } }); var bob = new Human('Bob', 25); var blackNinja = new Ninja('evil', 'Nin Tendo', 'unknown'); // blackNinja.isAlive = true // blackNinja.name = 'Nin Tendo' blackNinja.attack(bob);
As you can see, in MooTools we can define ‘classes’ easy, and expand them by creating ‘child-classes’.
Elements
Time to say a few words about handling elements in MooTools.
First of all, we have well-known function $():
var myElement = $(el);
The $$() function
It retrieves items by the HTML tag name.
var myElements = $$(aTag[, anElement[, Elements[, …]);
For example:
$$('a', 'b'); // all links and text bold text // an array containing only the element with the id 'myElement' $$('#myElement'); // an array with links with the 'myClass' CSS class, inside of // an element with id = 'myElement' $$('#myElement a.myClass');
Example #2:
var myAnchor = new Element('a', { 'href': 'http://mootools.net', 'class': 'myClass', 'html': 'Click me!', 'styles': { 'display': 'block', 'border': '1px solid black' }, 'events': { 'click': function() { alert('clicked'); }, 'mouseover': function() { alert('mouseovered'); } } });
Getting element properties
This property or multiple properties of element we will retrieve by writing:
var imgProps = $('myImage').getProperties('id', 'src', 'title', 'alt');
Assuming that in the document we have a picture with myImage ID.
Set / change element properties — for this purpose there is the method:
setProperty(‘element’, ‘prop’);
Example — using setProperty():
$('myImage').setProperty('src', 'mootools.png'); $('myImage').setProperties({ src: 'whatever.gif', alt: 'whatever dude' });
Deleting — for this purpose the is removeProperty() method, e.g.:
myElement.removeProperty('name');
Elements and events
The following code may already look familiar. An event handler we add via addEvent() method, e.g.:
$('myElement').addEvent('click', function() { alert('clicked!'); });
And we remove it by typing:
myElement.removeEvent(type, fn);
We can define support for multiple events, in following way:
myElement.addEvents(events); $('myElement').addEvents({ 'mouseover': function() { alert('mouseover'); }, 'click': function() { alert('click'); } });
Element.style — dynamic styles for element
The main method is setStyle(property, value); — let’s consider the example below.
Example — setting CSS styles through setStyle():
// width = 300px $('myElement').setStyle('width', '300px'); // width = 300px also $('myElement').setStyle('width', 300); var style = myElement.getStyle(property); $('myElement').getStyle('width'); // "300px" $('myElement').getStyle('width').toInt(); // 300
Typically for MooTools, we can set something (in this case styles) in bulk, via setStyles():
$('myElement').setStyles({ border: '1px solid #000', width: 300, height: 400 });
We can also get them in a simple way:
$('myElement').getStyles('width', 'height', 'padding'); // np.: {width: "10px", height: "10px", // padding: "10px 0px 10px 0px"}
Utilities.selectors
MooTools, like e.g. jQuery implements advanced selectors for matching the elements in a desired manner:
– = : expression “is equal to”
– *= : contains
– ^= : starts with substring
– $= : ends with
– != : not equal
– ~= : included in the list (separator: a space)
– |= : as above (separator: -)
Examples of selectors:
// returns all the links inside the myElement $('myElement').getElements('a'); // all tags with the name "dialog" $('myElement').getElements('input[name=dialog]'); // all inputs, whose name ends with the string 'log' $('myElement').getElements('input[name$=log]'); // all links starting with "mailto:" $('myElement').getElements('a[href^=mailto:]');
MooTools and JSON
The JSON format is also supported by MooTools.
Encoding — the encode() method of JSON object: var myJSON = JSON.encode(obj);
Example:
var fruitsJSON = JSON.encode({apple: 'red', lemon: 'yellow'}); // returns: '{"apple":"red","lemon":"yellow"}'
Decoding — decode() method: var object = JSON.decode(string[, secure]);
Example:
var myObject = JSON.decode('{"apple":"red","lemon":"yellow"}'); // returns: {apple: 'red', lemon: 'yellow'}
Cookie files in MooTools library
We have already written a fairly extensive article about the use of Cookies in JavaScript. MooTools has its own objects and methods to work with cookies.
Methods write() and read():
var myCookie = Cookie.write(key, value[, options]);
Example:
// save var myCookie = Cookie.write('username', 'Harold'); // create, read, remove (expire cookie) var myCookie = Cookie.write('username', 'Rene', { domain: 'mootools.net' }); if (Cookie.read('username') == 'Rene') { Cookie.dispose(myCookie); }
Fx — visual effects module
Fx is a part of framework, supports the creation of visual effects. Somewhat similarly, as for example in script.aculo.us.
Constructor:
var myFx = new Fx([options]);
Examples of effects:
var myFx = new Fx.Tween(element); // set red background myFx.set('background-color', '#f00'); // transition of colors var myFx = new Fx.Tween(element); myFx.start('background-color', '#000', '#f00'); myFx.start('background-color', '#00f');
Example — highlight:
// change background element on the light-blue $('myElement').highlight('#ddf');
Full description of this topic you can find here.
AJAX in MooTools
The Request object — is the basis of handling Ajax in case of this library.
Constructor:
var myRequest = new Request([options]);
The request GET we can do by:
var myHTMLRequest = new Request.HTML().get('myPage.html');
whereas POST:
var myHTMLRequest = new Request.HTML({url:'myPage.html'}). post("user_id=25&save=true");
Example — use the Request object for AJAX:
// basic var myReq = new Request({ method: 'get', url: 'handler.php' }); myReq.send('name=john&lastname=dorian'); // JSON format var myReq = new Request({url: 'getData.php', method: 'get', headers: {'X-Request': 'JSON'}}); myReq.setHeader('Last-Modified','Sat, 1 Jan 2005 05:00:00 GMT'); // XML format var myReq = new Request(url, {method: 'get', onSuccess: function(responseText, responseXML) { alert(this.getHeader('Date')); // np.: "Thu, 26 Feb 2009 20:26:06 GMT" }});
Example — multiple AJAX requests (XHR):
var xhr1 = new Request('/one.js', { evalResponse: true }); var xhr2 = new Request('abstraction.js', { evalResponse: true }); var xhr3 = new Request('template.js', { evalResponse: true }); var group = new Group(xhr1, xhr2, xhr3); group.addEvent('onComplete', function() { alert('All Scripts loaded'); }); xhr1.request(); xhr2.request(); xhr3.request();
In this example, an alert will appear after these three requests.
A detailed description of the next large topic, which is to support Ajax, can be found here.
Summary
MooTools is an extensive library with considerable potential. We discussed it in this short course quite briefly. Find out more at MooTools library homepage:
Check also MooTools tutorials:
http://sixrevisions.com/javascript/mootools_tutorials_and_example/
MooTools library is an interesting alternative to other JavaScript libraries, able to fill in many gaps of JavaScript.