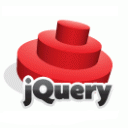
Today we present another tutorial about creating jQuery plugins, from practical point of view.
Creating jQuery plugins — further steps
The development of a plugin may be in a way as shown below. We define the elements — options and functions:
(function($) { // extend with a new function $.fn.myFunctionExt = function(param) { alert("The parameter is: " + param); }; // … // plugin code $.mySimplePlugin = function(options) { var myVar1 = 'A', …, actions = { doSomething: function() { // … }, }, // calling … }; })(jQuery);
Let’s do something practical, by adding more code.
Example — our own jQuery plugin:
(function($) { // we extend jQ by new simple function $.fn.myFunctionExt = function(param) { alert("The parameter is: " + param); }; // standard CSS modifier for elements $.fn.myCSSModifier = function() { return this.css({ margin: '5px', padding: '5px', border: 'solid 2px #f00', }); }; // plugin code $.mySimplePlugin = function(options) { // define properties and methods var str = 'abdef', number = 128, arr = ['One', 'Two', 'Three', '…'], actions = { test_alert: function() { this.myFunctionExt('X1'); // this.myFunctionExt(str); this.myFunctionExt(arr[1]); }, test_css: function() { // apply for all elements in body // this.myCSSModifier(); // apply only for specified element $('#d2').myCSSModifier(); }, default_action: function() { alert('Bye bye!'); } }, // core processing - options body = $('body'); if (options) { // multiple options if (typeof options == 'object') { for(i in options) { if (options[i] != false && actions[i]) { actions[i].call(body); } } } else { // string - one option if (actions[options]) { actions[options].call(body); } } } else { // no option specified - call default return actions['default_action'].call(body); } }; })(jQuery);
It is a fully working example. The result below.
Example of the use (assuming we include jQuery and the .js file with our plugin code in HTML document):
$(document).ready(function() { $.mySimplePlugin('test_alert'); // or // $.mySimplePlugin({test_css: true, test_alert: true}); });
The first option is a single method call:
$.mySimplePlugin('test_alert');
To trigger multiple actions at the same time, we write:
$(document).ready(function() { // $.mySimplePlugin('test_alert'); // -> $.mySimplePlugin({test_css: true, test_alert: true}); });
Let’s discuss the various elements of our plugin code.
Elements of jQuery plugin
The first two functions (myFunctionExt and myCSSModifier) extends jQuery by functionality added by us.
The available functions (actions), that can be performed on the elements of the document (page) we keep inside the “actions” object:
… // we extend jQ by new simple function $.fn.myFunctionExt = function(param) { alert("The parameter is: " + param); }; …
and
… actions = { test_alert: function() { this.myFunctionExt('X1'); // this.myFunctionExt(str); this.myFunctionExt(arr[1]); }, …
We also defined the properties, which can be referenced directly:
… $.mySimplePlugin = function(options) { var str = 'abdef', number = 128, arr = ['One', 'Two', 'Three', '…'], …
The “test_css” function, which modifies the style of elements, is noteworthy. We can apply styles to all elements of the document (default):
this.myCSSModifier();
or use the code only for a specific element:
$('#d2').myCSSModifier();
Of course, all we can customize as needed, e.g. passing data elements as parameters, etc.
The last part of the code checks if the string was passed (single instruction) or object (multiple instructions to execute).
… body = $('body'); if (options) { // multiple options if (typeof options == 'object') { for(i in options) { if (options[i] != false && actions[i]) { actions[i].call(body); } } } … }
And that’s it. As you can see, creating jQuery plugins doesn’t have to hurt.
The full example here:
http://directcode.eu/samples/jquery_plugins_dev/jq_plugin_dev_demo2.zip
Another example — a random string generator
Now we’ll write another plugin — a random string generator. We code elements step by step. Our plugin will start automatically.
Implementation:
(function($) { $.fn.randStrGenerator = function(options) { options = $.extend({ minLength: 7, randomStrLength: 12, theBox: this }, options); return this.each(function(index) { // run automatically evaluate(); function evaluate() { var randomPassword = generate(); $(options.theBox).val(randomPassword); } function generate() { return 'Something …'; } }); } })(jQuery)
To run the example, we need:
– input field:
<input type="text" id="random" />
– a call:
$(document).ready(function() { $('#random').randStrGenerator(); }); // or with our custom options // $('#random').randStrGenerator({ // minLength: 5, // randomStrLength: 10 // });
Full example on-line and do download here.
Creating yet another plugin — slide show
The third and final practical example for today is a slideshow plugin. The effect was achieved with minimum effort, and the code is trivial to use.
The plugin operates on HTML elements — let’s assume we have a list with images.
Our plugin will display them one by one, along the animation sequence.
Step 1 — define our list of images:
<div id="slideshow"> <img src="img/1.png" alt="" /> <img src="img/2.png" alt="" /> <img src="img/3.png" alt="" /> <img src="img/4.png" alt="" /> <img src="img/5.png" alt="" /> <img src="img/6.png" alt="" /> </div>
Step 2 — we need to define CSS styles for proper display of images:
#slideshow img { display: none; position: absolute; }
Step 3 — plugin implementation:
(function($) { $.mySlideShow = function(element, settings) { // setup var config = { 'fadeSpeed': 500, 'delay': 1000 }; if (settings) { $.extend(config, settings); } var obj = $(element); var img = obj.children('img'); var count = img.length; var i = 0; // display first image img.eq(0).show(); // run setInterval(function() { img.eq(i).fadeOut(config.fadeSpeed); i = (i + 1 == count) ? 0 : i + 1; img.eq(i).fadeIn(config.fadeSpeed); }, config.delay); return this; }; })(jQuery);
Step 4 — call the plugin for an element with images:
$(document).ready(function() { $.mySlideShow('#slideshow'); });
And ready! The interval executes the code at a time. Both the time and the speed of animation are customizable.
An example on-line:
Download:
http://directcode.eu/samples/jquery_plugins_dev/jq_plugin_dev_demo4_slideshow.zip
And that concludes today’s tutorial.
Summary
Creating jQuery plugins is not so complicated. In a fairly simple way we can change our code in jQuery plugin, and allow so many users to enjoy it easy.
Thank you.