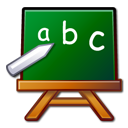
The jQuery is a very popular framework. By using it, we very often also use plug-ins, which is really a lot. And we could say, that they are one of the strongest features of this library.
Tutorial: creating jQuery plugins
Plugin is an elegant and practical solution to implement the code based on jQuery, that will be used repeatedly.
This is a very valuable skill, so let’s start. We implement a jQuery extension, that will operate on the objects of the document.
An example of such method can be fadeOut(), which operates on specific elements:
$('#my_element').fadeOut();
Implementation of a simple jQuery plugin
The general form, which can be treated as a template might look like this:
(function($) { $.fn.extend({ myplugin: function(options) { this.defaultOptions = {}; var settings = $.extend({}, this.defaultOptions, options); return this.each(function() { var $this = $(this); }); } }); })(jQuery);
But let’s go to our practical example.
Let’s create jq_plugin_dev_1.js file, in which we place basic code:
(function($) { var myApp = {}; $.extend(myApp, { name: 'jQuery plugin demo', version: '0.1', init: function() { alert(this.name + " v. " + this.version); } }); // init / run $(function() { myApp.init(); }); })(jQuery);
Thus, we have created a new type (myApp) that represents our simple “application”, and then we expanded it with new data (properties) and behavior (methods). The whole was “packed” in a jQuery plugin.
Simply attach this .js file to HTML page, and the code will run automatically. The result in our case will be: “jQuery plugin demo v. 0.1”.
Plugin should have operating parameters specified, which the user (programmer) can overwrite.
For example, replace this code:
… $(function() { myApp.init(); }); …
to the following:
… $(function() { myApp.name = 'Changed name'; myApp.version = '0.3'; myApp.init(); }); …
and the result will be: “Changed name v. 0.3”, which means that the default values are overwritten.
An example to download:
http://directcode.eu/samples/jquery_plugins_dev/jquery_plugins_dev.zip
These parameters are defined initially inside the $.extend method, which can extend parameters of our plugin:
(function($) { $.fn.mycarousel = function(options) { options = $.extend({ display_elems : 5, animation_speed : 100, pause_time : 1500, onScroll : function() { alert('Scrolling …'); } }, options); return this.each(function() { var $t = $(this); // operations … }); } })(jQuery);
In our example, we defined default parameters as “options”. The name can be any — it’s just an object, that we extend.
The user can call our plugin with own parameters:
$('myPhotoList').mycarousel({ display_elem : 10 });
The next part of code is:
return this.each(function() { // … });
It’s responsible for the passage of all the elements, that have been passed to the plugin. For each of these elements, the appropriate code of our plugin will be applied.
Example of call — assuming we have the list (ul/li) of photos:
$(document).ready(function() { $('.our_li_items').mycarousel(); });
Such a call will apply our plugin for list items with ‘our_li_items’ CSS class, and the code inside of this.each(function() … will be executed for each of them.
At the end, I would like to present a simple and practical example: code for removing HTML tags from a string, implemented as jQuery plugin.
An example comes from the website:
http://www.prodevtips.com/2009/12/05/html-stripping-jquery-plugin/
jQuery.fn.stripHTML = function() { return this.each(function() { var me = jQuery(this); var html = me.html(); me.html(html.replace(/<[^>]+>/g, "").replace(/<\/[^>]+>/g, "")); }); }
An example of call:
$(".article_title").decHTML().stripHTML();
The decHTML() function decodes the possible code, as described here.
And for the curious, an additional article about the basic issues of creating jQuery plugins:
http://learn.jquery.com/plugins/basic-plugin-creation/
Summary
jQuery plugins are one of the major factors, which determine the power of this framework.
Creating jQuery plugins is relatively simple — we create the code doing some task, and we “pack” this into the plugin, which we can reuse easily.
In the next article we will analyse more examples, and write our own, specific jQuery plugin.
(function($) { $.fn.thanks = function(options) { alert('Thank you!'); } })(jQuery);
Hey this is actually a really good tutorial. As somebody who just started working on my first plugin, I really learned a lot. It’s written in such a way that makes it much easier to understand compared to the jQuery documentation on this same topic. Thanks for this. I’m definitely bookmarking it this page!
Hello!
That’s great, thank you. Yes, it’s really basic. The second, more advanced tutorial about creating jQuery plugins is coming.
Best regards
Basic is what people need to get started! I really appreciate the effort put forth! I look forward to the next one. 🙂
OK! So here we go
https://javascript-html5-tutorial.com/creating-jquery-plugins-our-own-extensions-in-practice.html