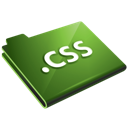
When we work with CSS, we often create or find interesting solutions for various tasks or problems encountered.
CSS Tips
1. Cross-browser CSS word break for too long text
For example, when we are creating front-end for the on-line chat, and there we have div-s for particular messages. The user writes very long text or URL address — any string of consecutive characters. Such a text won’t wrap and come out in a straight line outside our area (div).
We can process strings in our programming language, but we can make a painless deal with CSS. Our div needs to get CSS styles, which will take care:
.word-break { word-break: break-all; word-wrap: break-word; -ms-word-break: break-all; -webkit-hyphens: auto; -moz-hyphens: auto; hyphens: auto; }
This will ensure correct display of a long text in our element.
2. Two elements next to each other
In HTML and pure CSS we can simply write:
<div> <div style="float: left">Left<div> <div style="float: right">Right<div> </div>
3. Horizontal centering
To center an element horizontally, let’s add margin: 0 auto style:
#my-div { width: 800px; margin: 0 auto }
This item will be centered relative to its parent element.
To center elements inside of some element, let’s use text-align: center style:
<div style="text-align: center"> <p>AAAAA</p> <p>BBBBB</p> </div>
4. Rounded corners of an element
Let’s use simply the border-radius CSS property:
.element { border-radius: 5px }
It will set 5px radius for all 4 corners.
However, it’s a good idea to use cross-browser styles, by adding properties typical for Mozilla and Webkit based browsers:
.element { border-radius: 5px; -webkit-border-radius: 5px; -moz-border-radius: 5px; }
Of course there are properties to rounding only some corners, for example top-left:
.element { border-top-left-radius: 5px; -webkit-border-top-left-radius: 5px; -moz-border-radius-top-left; }
5. Conditional comments
Unfortunately *some* browsers still needs special care, like adding CSS or JS files, but necessary only in case of *this* browser.
Conditional comments comes with the help:
<!--[if IE]> <link rel="stylesheet" type="text/css" href="special-care.css" /> < ![endif]-->
The code above means adding the special-care.css file only in case of IE browser.
We can determine versions:
<!--[if IE 6]> - targets IE6 only --> <!--[if gt IE 6]> - targets IE7 and above --> <!--[if lt IE 6]> - targets IE5.5 and below --> <!--[if gte IE 6]> - targets IE6 and above --> <!--[if lte IE 6]> - targets IE6 and below -->
6. 100% Height
A simple solution for the case, where we want to make our element (e.g. vertical div for left menu in web app) held 100% of the page.
For this, we simply define height 100% both for html and body elements, and for our div:
html, body { height: 100% } … #our-div-left { min-height: 100% }
7. Remove borders from image-links
Usually, by default such elements are displaying annoying border, which can be simply removed:
a image { border: 0 } /* OR a image { border: none } */
8. Visibility vs Display
What should I use? The difference is very simple:
{ visibility: hidden } — in this an element will be hidden, but an empty space will remain in its place
while in the case of:
{ display: none } — both element and the place which this element would take, will be hidden.
9. Cross-Browser Transparency
Not all browsers support the opacity property, so the following code may be still useful:
.element { filter:alpha(opacity=50); /* IE */ -moz-opacity:0.5; /* Mozilla */ /* Older versions of Safari and khtml-based browsers */ -khtml-opacity: 0.5; opacity: 0.5; /* Standard */ }
10. Styles for active form element
To improve usability we can set styles added dynamically for the form element (input), in which the user is currently working. The :focus pseudo-selector will help us:
input:focus { border: 2px solid #f00; }
With this, the current input where user is working, will be marked by styles we’ve set.
CSS tips — summary
That’s it for today. I hope that this 10 tips we collected here will be useful sometimes, to help in faster development of a good front-end.