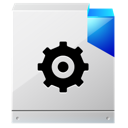
OK, it’s time for the next JS and jQuery mini tips. Today something about Fancybox plugin, check-boxes and parsing URLs in raw text.
JavaScript and jQuery tips
To the point.
1. Conditional form submit using JavaScript / jQuery
It’s about sending the form only if the user checked a check-box (e.g. accept the Terms of Service). Of course it’s simple validation in JS, without using language such as PHP.
There is our check-box:
<div class="error">Please accept the TOS!</div> <input type="checkbox" name="terms" id="terms" value="1" /> <label style="display: inline;" for="terms">I agree … </label>
and the form:
<form id="submit-form" action="index.php" method="post"> <input type="hidden" name="hidden" value="1" /> <input type="text" id="name" /> … other inputs … <input type="submit" id="submit-button" value="Send" /> </form>
The validation is very simple to implement using jQuery. We check if our check-box is checked. If not, we prevent sending the form (default action, e.preventDefault()) and display the error message.
If checked, we hide the error message, and additionally we set the submit button as disabeld, to avoid sending the form by user more than once.
JS code:
$('#submit-form').bind('submit', function (e) { var terms = $('#terms').is(':checked'); if (terms !== true) { e.preventDefault(); $(".error").show(); } else { $(".error").hide(); $('#submit-button').attr("disabled", true); } });
2. How to prevent closing Fancybox (outside, etc)
Doubtless from time to time we do a project, where we have to show some dialog. This dialog contains for example a form, or some information, or just asks the user to click one of the buttons, to determine further action to execute.
Sometimes it is a required that the user cannot close such a dialog. Here is an example of how to do it using Fancybox jQuery plugin:
$.fancybox({ // hide close Btn closeBtn : false, // prevents closing when clicking INSIDE fancybox closeClick : false, helpers : { // prevents closing when clicking OUTSIDE fancybox overlay : { closeClick: false } }, keys : { close: null }, 'padding': 0, 'width': 760, 'height': 600, // show the content of element content: $('#our-div-with-content').show() });
This code prevents closing Fancybox through:
– disable “Close” button
– no closing the dialog after clicking outside the overlay
– no reaction for Escape key
With this, user won’t have a possibility to close displayed Fancybox.
3. JavaScript and clickable links in the text
Simply it’s about processing the text in JavaScipt, to change all the URLs into clickable links. In this case we can use regular expressions.
By the way, let’s take a look at simple example in PHP:
//'<a class="'.$class.'" href="//$3" target="'.$target.'">$1$3</a>', function make_links($string) { return preg_replace( '%(https?|ftp|mailto)://([-A-Z0-9./_*?&;=#]+)%i', '<p><a target="blank" rel="nofollow" href="$0"><u>$0</u></a></p><br>', $string); }
It’s not so scary. Let’s back to JavaScript and a little bit more clean example, which is able to handle URLs, ftp and mailto links:
function processLinksInText(text) { // http://, https://, ftp:// var urlPattern = /\b(?:https?|ftp):\/\/[a-z0-9-+&@#\/%?=~_|!:,.;]*[a-z0-9-+&@#\/%=~_|]/gim; // www., http:// or https:// var pseudoUrlPattern = /(^|[^\/])(www\.[\S]+(\b|$))/gim; // e-mail var emailAddressPattern = /(([a-zA-Z0-9_\-\.]+)@[a-zA-Z_]+?(?:\.[a-zA-Z]{2,6}))+/gim; return text .replace(urlPattern, '<a target="_blank" href="$&">$&</a>') .replace(pseudoUrlPattern, '$1<a target="_blank" href="http://$2">$2</a>') .replace(emailAddressPattern, '<a target="_blank" href="mailto:$1">$1</a>'); }
We just have to define the correct regular expressions, and then call the .replace() method for each expression.
To read also:
http://stackoverflow.com/questions/37684/how-to-replace-plain-urls-with-links
Summary
That’s it for today. Other small tips concerning solutions of the problems, that the developer can meet when is working on the projects.
Thank you.
[…] https://javascript-html5-tutorial.com/javascript-and-jquery-mini-tips-2.html […]