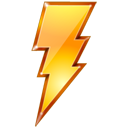
The next article about JS and jQ tips, but in fresh, concise form.
Quick JS and jQuery mini tips
1. Clickable background image on the website
It’s about the case, when we want to make a clickable background of the website, for example, as advert pointing the user to some url after click on website background.
Wrapping website content, or adding onclick handler for the background, or other solutions which would come to our head as first could be not enough.
Here we have simple and working solution. As first we set image for the background:
var img = "http://our-page.com/image.png"; var link = "http://target-website.com"; document.body.style.backgroundImage = "url('" + img + "')";
Next thing is click handler — working, and not allowing to open new page / tab many times after one click:
$('body').click(function(e) { e.stopPropagation(); if (e.target === this) { // window.open(link); window.location = link; } });
That’s it!
2. JS, jQuery and loading external website (to the iframe)
In our project there could be a requirement to load the content of external website. We can do it in few ways, including Ajax (let’s don’t forget about CORS).
Some people try to use HTML elements like Object or Iframe.
In our example we will use iframe. As solutions that may work for few test URLs, will fail for one of the further URLs, in case when we have to load external website, e.g. as a preview, we can use iframe and jQuery. Such a solution should work actually always.
YES, from the other hand it’s related with security issues, which we have to take into consideration. However, when we have to do something like this, we can try following way:
<iframe id="content" src="about:blank" style="overflow-y: visible;" sandbox="allow-forms allow-popups allow-scripts"></iframe>
With this we will load whole content of external website, and it will be visible. Please pay attention on the sandbox parameter of the iframe (HTML5).
Our settings are, among other, to display forms of external website, and allow to execute scripts, but still helping us to avoid solutions like “iframe buster”, by preventing redirections.
More about sandbox:
http://www.w3schools.com/tags/att_iframe_sandbox.asp
The rest if also easy — in jQuery we set the “src” attribute for our iframe, by setting external website URL as a value:
var the_url = "http://our-website.com"; // … $(document).ready(function() { $("#content").attr("src", the_url); $("#content").css("width", "100%"); $("#content").css("height", "1080px"); });
And that’s all in the matter.
3. Checking Internet connection in JavaScript
So how to check it in JS? And actually for what? Front-end of our web application could be able to check if there network connection is present, and do some action otherwise.
Our web apps need Internet connection actually for to do most of operations. Especially in case of critical operations, the break of Internet connection can be an issue.
We can detect and handle such a case to avoid errors and problems. For example we can switch collection the data by our app to web storage instead of online communication, and do sync after connection is restored.
Interesting approach about checking Internet connection in JS has been described in details here:
http://www.kirupa.com/html5/check_if_internet_connection_exists_in_javascript.htm
JS and jQuery mini tips — summary
It is good to collect and publish some solutions for the others. Especially when we had to solve it in real tasks. There will be more similar post soon, with next tips and tricks.
Everyday we have some issues to solve, and sometimes they are interesting and worth of publishing, so maybe someone else will be able to solve its issues someday. Faster.
Thank you!