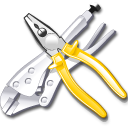
What is that? The Data URI scheme is simply a way to represent the information, but in such a way the data usually kept in files (e.g. images) are available in a form of text string!
Such a string has its own format, and allows developers to easily transfer the resource, e.g. between Web applications, or use it directly in JavaScript / CSS code.
Moreover, there’s no need to execute additional HTTP requests to fetch the files, as all the data can be fetched immediately, in one request.
The Data URI string has a following form:
data:[media type][;charset=xxx][;base64],data
Generating and using the Data URI
We talked about using Data URI in the one of older posts, concerning HTML5 Canvas. operations. Today we expand this topic.
The Data URI string can be used directly as an attribute of HTML image source and displayed in a browser:
<img src="data:image/png;base64,iVBORw0KGgoAAAANSUhEUgA…" />
OK, but actually how can I obtain this Data URI string?
We can for example use the toDataURL() method of the HTML5 Canvas object:
myCanvas.toDataURL();
However, would be great to have more convenient method, on the server-side. Fortunately doing this task in PHP is very simple.
Converting the file to the data — generating the Data URI in PHP
PHP provides everything we need — MIME type detection and base64 encoding method.
$filename = 'test.png'; $finfo = finfo_open(FILEINFO_MIME_TYPE); $mimetype = finfo_file($finfo, $filename); finfo_close($finfo); $contents = file_get_contents($filename); $data_uri = "data:" . $mimetype . ";base64," . base64_encode($contents); echo $data_uri;
Few lines and we have our file represented as Data URI!
Usage is very simple
Such a string we can paste to CSS class, or simply use as src attribute of the img element:
<img src="<?php echo $data_uri ?>" />
For test purpose I used this small, PNG image:
Full Data URI string generated for this image you can find here:
Copy, paste and test. I don’t send you my file, but only text string. You can paste it in the source of your website and display. Without having any file!
Sample — HTML:
<img src="HERE-OUR-DATA-URI-STRING" alt="Data URI" />
Sample — embedding in CSS:
body { background: url(data:image/png;base64,iVBORw0KGgoAAAANSUhEUgAAAMwAAADECAIAAABcE1nIAAAAAXNSR0IArs4c[…]) no-repeat left center; }
JavaScript and adding image dynamically:
var str = "data:image/png;base64,iVBORw0KG… [THE-REST-OF-OUR-STRING]"; var image = new Image(); image.src = str; image.onload = function() { document.body.appendChild(image); }
Cool?
Resources:
– MDN
https://developer.mozilla.org/en-US/docs/Web/HTTP/data_URIs
Summary
If we have seen such strings in the past, and wondered what is that, now we know it’s any magic — it’s Data URI.
It allows us to easily embed encoded images in our sources, or send across our web applications.
Enjoy! And don’t forget to check our second blog:
http://javascript-html5-tutorial.net