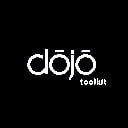
In the part 2 of the Dojo Toolkit basic course, we will do the review on various aspects of working with this JS framework.
The Dojo Toolkit library in practice
The possibilities of the library already discussed in the first part, although the words are unnecessary when we revise examples.
Configuration
At the beginning — the Dojo configuration mechanism.
The dojoConfig object is the main mechanism Dojo configuration in our application / web page. In older versions this object was called djConfig — it’s outdated name, which we can meet in old codes based on the Dojo Toolkit.
Example of use — load the library with setting configuration parameters:
JavaScript
dojoConfig= { has: { "dojo-firebug": true // has (dojo-firebug sub-property) }, // others parseOnLoad: false, foo: "bar", async: true };
HTML
<script src="//ajax.googleapis.com/ajax/libs/dojo/1.9.1/dojo/dojo.js"> </script>
This configuration must be defined before loading dojo.js, otherwise our settings will be ignored.
It should be noted the difference between dojo/_base/config and dojoConfig (it’s for determination of input parameters). In this way we “communicate” with the code involved in the loading of modules.
The declaration of parameters can also be accomplished in the following way (via data-dojo-config):
<script src="//ajax.googleapis.com/ajax/libs/dojo/1.9.1/dojo/dojo.js" data-dojo-config="has:{'dojo-firebug': true}, parseOnLoad: false, foo: 'bar', async: 1"> </script>
Dojo Toolkit configuration options are quite extensive, as we will see in the right place documentation.
Events and more about DOM
In addition to handling the DOM, a large part of the JavaScript code used to generate events and responding to them. In Dojo Toolkit we have dojo/on — uniform API event handler in an accessible way.
Let’s consider the next example.
HTML
<button id="myButton">Click</button> <div id="myDiv">Move the cursor</div>
JavaScript — handling
require(["dojo/on", "dojo/dom", "dojo/dom-style", "dojo/mouse", "dojo/domReady!"], function(on, dom, domStyle, mouse) { var myButton = dom.byId("myButton"); var myDiv = dom.byId("myDiv"); // for a button on(myButton, "click", function(evt) { domStyle.set(myDiv, "backgroundColor", "blue"); }); // for div element on(myDiv, mouse.enter, function(evt) { domStyle.set(myDiv, "backgroundColor", "red"); }); on(myDiv, mouse.leave, function(evt) { domStyle.set(myDiv, "backgroundColor", ""); }); });
Arguments: ID of HTML element, a type of an event, and a function with implementation of the right action to execute.
In contrast, a way to appeal (removal) event handler is handle.remove.
var handle = on(myButton, "click", function(evt){ // usuń event handler handle.remove(); alert("Test"); });
JSON and Dojo Toolkit
A framework such as Dojo must have the appropriate module for working with JSON format.
Parser and JSON serialization
The dojo/json module has two methods:
– parse() — parsing JSON object
– stringify() — serialization to JSON
Arguments for the parse() method: JSON string to processing, and second optional parameter (true or false) to set whether secure parsing should be used.
Example:
require(["dojo/json"], function(JSON) { JSON.parse('{"hello":"world"}', true); });
The stringify() method from Dojo Toolkit accepts the same arguments, as JSON.stringify().
Example:
require(["dojo/json"], function(JSON) { var jsonString = JSON.stringify({ hello: "world" }); });
In an article about JSON in JavaScript, we have mentioned JSONP (JSON with Padding, cross-domain requests). The Dojo Toolkit documentation has also a small article about JSONP topic.
XML
A now few words about XML. Dojo Toolkit provides a unified support for XML: dojox.xml.
Example:
dojo.require("dojox.xml.DomParser"); function init() { // Parse text and generate an JS DOM var xml = "<tnode><node>Some Text</node>"; xml += "<node>Some Other Text</node></tnode>"; var jsdom = dojox.xml.DomParser.parse(xml); console.debug(jsdom); } dojo.ready(init);
The Dojo’s documentation part about XML available here.
AJAX in Dojo Toolkit
We already discussed AJAX basics, in “pure” JavaScript, and also using JS frameworks. Dojo Toolkit also has great support for Ajax.
Requests — the simplest example below:
require(["dojo/request"], function(request) { request("our-file.txt").then( function(text) { console.log("File content: " + text); }, function(error) { console.log("Error: " + error); } ); });
The file content is loaded by XHR (XMLHttpRequest).
A more practical example is simple logging-in based on AJAX. Assuming we have HTML form (my-form) with appropriate fields (e.g.: login and pass), which will be sent and handled in process.php file.
require(["dojo/dom", "dojo/on", "dojo/request", "dojo/dom-form"], function(dom, on, request, domForm) { var form = dom.byId('my-form'); // add an event handler for onSubmit on(form, "submit", function(evt) { // prevent the default behavior of a form submit action evt.stopPropagation(); evt.preventDefault(); // send data to the server (PHP file) via POST method request.post("demo.php", { // here we send data from fields data: domForm.toObject("my-form"), timeout: 2000 }).then(function(response){ alert(response); }); }); } );
In several lines of code we have getting and sending data from the form fields, an event handler and callback.
AMD Modules
Finally a word about the AMD modules (and no, it’s not about the processor).
Dojo Toolkit supports modules written in the Asynchronous Module Definition (AMD) format. This format is available in the library since version 1.7 and contains many improvements over the “normal” / existing modules in the Dojo, including full asynchronous and portability packages, and improved management of dependencies.
The documentation includes basic and advanced tutorial allowing us to explore AMD:
Summary
And we finished the 2nd part of basic Dojo Toolkit course. In the 3rd (summary) part we will work with visual effects and animations.