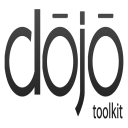
In the third section summarizing the basic course of Dojo Toolkit, we will see the creation of visual effects using this library.
Dojo Toolkit: effects and animations
Any self-respecting JavaScript framework includes solutions to support programming visual effects. It is no different in the Dojo Toolkit. The library since version 1.9 provides two modules: dojo/_base/fx and dojo/fx.
The dojo/_base/fx module gives the programmer the basic methods for effects, including animateProperty, anim, fadeIn or fadeOut, whilst the dojo/fx provides more advanced methods, such as chain, combine, wipeIn, wipeOut or slideTo.
So let’s move on to the practice.
Fading
This is a popular effect. Fading in / fading out ie appearance / disappearance of elements with animation. A simple example below.
HTML
<button id="fadeOut_btn">Fade out</button> <button id="fadeIn_btn">Fade in</button> <div id="fade_block" style="background-color: #f00; width: 40%"> <h2>Some content here …</h2> </div>
JavaScript
require( ["dojo/_base/fx", "dojo/on", "dojo/dom", "dojo/domReady!"], function(fx, on, dom) { var fadeOut_btn = dom.byId("fadeOut_btn"), fadeIn_btn = dom.byId("fadeIn_btn"), fade_block = dom.byId("fade_block"); on(fadeOut_btn, "click", function(evt) { fx.fadeOut({ node: fade_block }).play(); }); on(fadeIn_btn, "click", function(evt) { fx.fadeIn({ node: fade_block }).play(); }); });
Animation functions take one argument: the object with parameters. The most important is DOM node (ID of an element).
In addition to this, another feature that is defined with animations is their duration. This parameter is expressed in milliseconds (ms). In Dojo Toolkit it’s 350 ms by default.
At the end of the function call, we see .play(), which gives a signal to reproduce the animation.
Animation functions returns objects of dojo/_base/fx::Animation, and in addition also include: pause, stop, status, and gotoPercent methods.
Sliding
Fade manipulates the visibility of an element. And sliding (fx.slideTo) changes the position of element with animation effect. Below is an example of use.
HTML
<button id="slide_btn">Slide the block</button> <button id="slideBack_btn">Return</button> <div id="test_block" style="background-color: #f00; width: 40%"> <h2>Some content here …</h2> </div>
JavaScript
require( ["dojo/fx", "dojo/on", "dojo/dom", "dojo/domReady!"], function(fx, on, dom) { var slide_btn = dom.byId("slide_btn"), slideBack_btn = dom.byId("slideBack_btn"), test_block = dom.byId("test_block"); on(slide_btn, "click", function(evt) { fx.slideTo({ node: test_block, left: "222", top: "222" }) .play(); }); on(slideBack_btn, "click", function(evt) { fx.slideTo({ node: test_block, left: "0", top: "100" }) .play(); }); });
In this case, the parameters are not only the ID element (node), but also the value for the new x and y position (left and top), expressed in pixels (px).
Animation Events
As already mentioned, the methods return objects of dojo/_base/fx::Animation. These objects provides also events to listen for certain actions during or after the animation.
The most important are beforeBegin and onEnd (before and after animation).
Example:
// HTML elements the same as in the previous example … require( ["dojo/fx", "dojo/on", "dojo/dom-style", "dojo/dom", "dojo/domReady!"], function(fx, on, style, dom) { var slide_btn = dom.byId("slide_btn"), slideBack_btn = dom.byId("slideBack_btn"), test_block = dom.byId("test_block"); on(slide_btn, "click", function(evt) { var anim = fx.slideTo({ node: test_block, left: "200", top: "200", beforeBegin: function() { // alert("Target: ", test_block); style.set(test_block, { left: "0px", top: "100px" }); } }); // handle the termination event on(anim, "End", function() { style.set(test_block, { backgroundColor: "green" }); }, true); // we have to start the animation anim.play(); }); // code for handling slideBack_btn button });
We used beforeBegin to ensure execution of the handler code in the correct order (at the beginning).
Chaining
It means combining animation as sequences. It is a convenient way to define an animation sequence (fx.chain).
Dojo Toolkit with dojo/fx module gives us methods of defining animation performed sequentially or even in parallel.
Example — animation sequences:
// HTML elements the same as in the previous example … require( ["dojo/_base/fx", "dojo/fx", "dojo/on", "dojo/dom", "dojo/domReady!"], function(baseFx, fx, on, dom) { var slide_btn = dom.byId("slide_btn"), slideBack_btn = dom.byId("slideBack_btn"), test_block = dom.byId("test_block"); on(slide_btn, "click", function(evt) { fx.chain([ baseFx.fadeIn({ node: test_block }), fx.slideTo({ node: test_block, left: "200", top: "200" }), baseFx.fadeOut({ node: test_block }) ]).play(); }); on(slideBack_btn, "click", function(evt) { fx.chain([ baseFx.fadeIn({ node: test_block }), fx.slideTo({ node: test_block, left: "0", top: "100" }), baseFx.fadeOut({ node: test_block }) ]).play(); }); });
By fx.chain we easily created and started few visual effects directly in one call. We don’t start individual effects (animations) but everything as a whole.
Links to resources in the documentation:
– dojo/fx — http://dojotoolkit.org/reference-guide/1.9/dojo/fx.html
– dojo/_base/fx — http://dojotoolkit.org/reference-guide/1.9/dojo/_base/fx.html
Summary
It’s probably not the end of articles or tutorials about the Dojo Toolkit, but the end of the basic course about this framework.
Today we learned how easy it is to create effects and animations, and this is after all just the tip of the Dojo Toolkit library capabilities.
dojo/thank_you