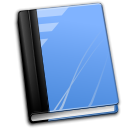
I18n so internationalization, and also L10n, are important issues, whenever we create applications targeted for users from many countries.
I18n in JavaScript
Certainly, many solutions such as frameworks, SDKs for mobile apps and programming languages, have support for multiple languages in developed application.
Today we try to find such solutions in JavaScript. We look at some of the ways to support multiple languages in the JavaScript code.
Simple solutions for I18n in JavaScript (pure language)
Let’s start with the simplest of solutions, based on fundamental JavaScript structures. Such solutions may be sufficient for simple web applications.
Example — simple support for I18n in JavaScript:
// set language var _lang = "de"; // define translations var hello_message_homepage = { en: "English hello", fr: "French hello", de: "German hello", pl: "Polish hello"}; var introduction_text_homepage = { en: "English text…", fr: "French text…", de: "German text…", pl: "Polish text…"}; // etc … // get translation for current language alert(hello_message_homepage[_lang]); alert(introduction_text_homepage[_lang]);
In this example we simply display an alert with obtained translation. In practice it may be, for example, substitution of a text label of selected HTML element.
We used language symbols (en, de, etc) as keys of the array with translations for particular messages. Simple and effective.
Another way
In this case we introduce some order, and we will keep translations in separate files.
var _lang = "de"; document.write("<scr"+"ipt src='lang/"+lang+"/messages.js'></scr"+"ipt>");
Having defined the files in lang/ directory, our script will include the appropriate file for the current language.
For example:
// file lang/en/messages.js var hello_message_homepage = "English hello"; var introduction_text_homepage = "English text…";
// file lang/de/messages.js var hello_message_homepage = "German hello"; var introduction_text_homepage = "German text…";
… and other languages, which we want to support.
When the script attach the file into HTML document, we refer to our variables, for example:
alert(hello_message_homepage), obtaining correct translation.
Of course, these are the basic solutions, without error handling, etc.. They are the only clue that can follow in order to develop the most appropriate solution for our application.
Let’s take a step further towards libraries.
The R.js library
R.js is a small library under the MIT license, which supports I18n in JavaScript. It has no dependencies and does not interfere with other libraries.
The usage is quite simple:
<script type="text/javascript" src="R.js"></script> <script type="text/javascript"> R.registerLocale('en-GB', { // English (Source) // Translated String 'A Translated String': 'A Translated String', }); R.registerLocale('pl', { // English (Source) // Translated String 'A Translated String': 'Przetłumaczony łańcuch', }); </script> <script type="text/javascript"> var h1 = document.createElement('h1'); h1.innerHTML = R('A Translated String'); </script>
It is quite an interesting alternative to support I18n in JavaScript.
Library available on GitHub:
https://github.com/keithamus/R.js
I18n and JavaScript frameworks
Firstly we can recommend to take a look at script-tutorials.com, on a simple I18n solution based on jQuery:
http://www.script-tutorials.com/how-to-translate-your-site-in-runtime-using-jquery/
Another interesting article briefly describes I18n in JavaScript using jQuery (Ajax) and XML:
http://www.webgeekly.com/tutorials/jquery/how-to-make-your-site-multilingual-using-xml-and-jquery/
And as we are already around jQuery, it is worth mentioning a library with extensive possibilities — jQuery Globalize:
https://github.com/jquery/globalize
To a wide range of solutions already we can still add Dojo Toolkit.
The dojo/i18n module:
http://dojotoolkit.org/reference-guide/1.9/dojo/i18n.html
Tutorial — I18n using Dojo Toolkit
http://dojotoolkit.org/documentation/tutorials/1.9/i18n/
Summary
We presented methods known to us about I18n in JavaScript. In practice, we can restrict ourselves to the simplest ways.
If we create something more advanced for a wider range of users, then we look to more refined and uniform methods. Among such examples we can mention about WYSIWYG editors, which are distributed with translations for many languages.
Such projects usually has a standard way to handle I18n, and to add new translations simply.
There is another, a very simple method. Namely, if our web application is created using also backend (e.g. in PHP), then translations handling may be remove from JavaScript level, if there are no contraindications.
In such case JavaScript variables will be generated in PHP code, where some I18n solution is already available. I worked with projects with this approach, with very good effects.
As the opportunities are different, everyone for certain can find something suitable for the project.
alert(thank_you_message_homepage[_lang]);
And as usually, there is something nice from Mozilla:
https://developer.mozilla.org/en-US/docs/Mozilla/Projects/L20n