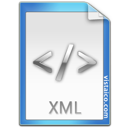
In this article we take a look on how to work with XML in JavaScript. About the processing of XML in JavaScript we already mentioned in an introduction to AJAX.
Today we go further, and of course will try to find solutions that help us in tasks related to XML.
XML in JavaScript
Though in JavaScript probably more popular is work with the JSON format, however, sometimes we have to deal with XML data.
For example, I prefer JSON, but in case of integrating the application with an external API, XML is imposed by the client.
Let’s move on to the examples, and start with test XML structure, defined as a string in JavaScript:
var inputXML = '<?xml version="1.0" ?><root>Tester</root>';
The inputXML variable is a simple string. The function presented below converts such string to the object (XML), so we will be able to operate in JavaScript.
function stringToXML(text) { try { var xml = null; if (window.DOMParser) { var parser = new DOMParser(); xml = parser.parseFromString(text, "text/xml"); var foundErr = xml.getElementsByTagName("parsererror"); if ( !foundErr || !foundErr.length || !foundErr[0].childNodes.length) { return xml; } return null; } else { xml = new ActiveXObject("Microsoft.XMLDOM"); xml.async = false; xml.loadXML(text); return xml; } } catch (e) { ; } }
Using this function, we create an object based on a string containing XML, and then we process this object:
var xmldoc = stringToXML(inputXML); var root_node = xmldoc.getElementsByTagName('root').item(0); alert(root_node.firstChild.data);
If we try:
alert(xmldoc);
we receive a result: [object XMLDocument]. And very well — this is what we wanted in this case.
The other side
What if we have such object, and we want to process it to the string, e.g. to view the structure? Simply we do reverse operation, which presents the following code:
function xmlToString(objXML) { if (window.ActiveXObject) { return objXML.xml; } else { return (new XMLSerializer()).serializeToString(objXML); } } // example of use: alert(xmlToString(xmldoc)); // ->: <?xml version="1.0" encoding="UTF-8"?><root>Tester</root>
Working with string
Is there a simple way just to cut the value form string?
Someone may say that only wants to retrieve a value from a text string containing with simple XML, without additional operations. Well, we can write a function which operates directly on the XML string.
For example, we use input data as below:
var inputXML = '<?xml version="1.0" ?><root><title>Testing</title>'; inputXML += '<content>Lorem ipsum…</content></root>';
Process the XML string in JavaScript:
function getXmlValue(input, xmlMarkup) { var beg = ""; var end = ""; var dt = 0; var xmlVal = ""; var start = "<" + xmlMarkup + ">"; var stop = "</" + xmlMarkup + ">"; beg = input.indexOf(start); end = input.indexOf(stop); dt = start.length; if ( (beg !== false) && (end !== false) ) { xmlVal = input.substr(beg + dt, end - beg - dt); } return xmlVal; } alert(getXmlValue(inputXML, 'content'));
In second parameter we define the name of tag, from which we want to extract data. The function is basically trivial, but really was useful in older projects.
Converting XML to an array of JavaScript
We converted the string to an object using our stringToXML() function. We can use it in the next example, where we go one step further: process object into an easy to use array of JavaScript.
The following function will do this task:
function xmlToJsArray(rawXmlData) { var output = new Array(); var i, j, rec, obj; for (i = 0; i < rawXmlData.childNodes.length; i++) { if (rawXmlData.childNodes[i].nodeType == 1) { rec = rawXmlData.childNodes[i]; obj = output[output.length] = new Object(); for (j = 0; j < rec.childNodes.length; j++) { if (rec.childNodes[j].nodeType == 1) { obj[rec.childNodes[j].tagName] = rec.childNodes[j].firstChild.nodeValue; } } } } return output; } // example var arr = xmlToJsArray(stringToXML(inputXML)); // in our example the result will be: // '0' … // 'title' => "Testing" // 'content' => "Lorem ipsum…" // call: alert(arr[0]['title']);
And thus we have the data from XML in JavaScript array, which is more convenient to process.
Using jQuery for processing XML in JavaScript
As might be expected, jQuery framework provides us support for working with XML in JavaScript.
The base will be jQuery.parseXML: http://api.jquery.com/jQuery.parseXML/.
Example:
var xml = "<rss version='2.0'><channel><title>The Title"; xml += "</title></channel></rss>"; jQuery(document).ready(function($) { xmlDoc = $.parseXML(xml); $xml = $(xmlDoc); $title = $xml.find("title"); // get value alert($title.text()); // set value $title.text("XML Title"); });
With jQuery, we do all we need in a few lines of code.
There is a lot of plugins dedicated for XML, such as jParse: http://jparse.kylerush.net/.
Summary
This concludes our brief overview of methods for handling XML in JavaScript. Surely there is more — here we relied on the experience of own projects.
thank_you.xml