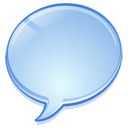
Today we recommend the jQuery tutorial, that shows how to create own ToolTips. In four easy steps.
jQuery tutorial — creating own tooltips
Source available: here.
Step 1 — the project
Create a new project (e.g. in Aptana IDE) or simply create a structure as below:
Tutorial jQuery — tooltip
Step 2 — the view
We create the basic look, assuming that the tooltip element will be “span”:
style.css:
span#own-tooltip { height: 20px; position: absolute; top: 100px; left: 100px; border: 2px solid #00f; background-color: #fff; color: #00f; padding: 4px; display: none; }
Appearance can be adapted freely by changing the CSS presented above.
Step 3 — HTML structure
We create HTML structure. In my case, I wanted tooltips for links, so the text for the tooltip is gathered from “title” attribute of an element.
For example:
<div id="main-place"> <a href="http://www.javascript-html5-tutorial.com/" title="JavaScript and HTML5 blog">Link 1</a> <a href="http://www.google.pl/" title="Link to Google">Link 2</a> <a href="http://www.odesk.com/" title="Odesk">Link 3</a> </div>
Step 4 — JavaScript / jQuery code
We code everything in JS using jQuery.
The things to do are:
– adding tooltip element to DOM
– traverse all elements for which we want to show tooltip
– set the position and the text for tooltip, display tooltip
– optionally we can add some visual effects
Such operations we do in following way:
// jqt.js $(document).ready(function() { // add an element to document body $("body").append("<span id='own-tooltip'></span>"); // show the tooltip for "a" html element with title attribute $("a[title]").each(function() { $(this).hover(function(e) { // show the tooltip below element $().mousemove(function(e) { var dY = e.pageY + 4; var dX = e.pageX + 4; $("#own-tooltip").css({'top': dY, 'left': dX}); }); $("#own-tooltip").stop(true, true); // get text from title attribute and set as tooltip's // text $("#own-tooltip") .html($(this).attr('title')) // show the tooltip with fadeIn effect .fadeIn(100); $(this).removeAttr('title'); }, function() { $("#own-tooltip").stop(true, true); // hide $("#own-tooltip").fadeOut(100); // cleanups $(this).attr('title', $("#own-tooltip").html()); }); }); });
That’s all. Final effect you can see here.
Source code to download : available here.
Summary
As you can see, we just can write few lines of code to create something cool and useful. The jQuery framework helped a lot of course.
Thank you!
[…] https://javascript-html5-tutorial.com/jquery-tutorial-own-tooltips.html […]