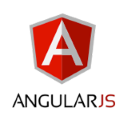
In the 3rd part of basic Angular course, we will continue topics from the previous part, as well we will touch next related topics.
Further learning AngularJS
Let’s start with filters. It’s a yet another Angular feature, which takes off a part of tedious work from the programmer. Let’s see them in action, when formatting various kind of data.
AngularJS filters
Even in the previous part we could see how simple in use (but useful) the filters are. For example:
… <p>{{ message.sent | date:'MMM d, y h:mm:ss a' }}</p> …
AngularJS provides various filters, which facilitate processing and formatting data:
– currency – format a number to a currency format
– date – format a date to a specified format
– filter – select a subset of items from an array
– json – format an object to a JSON string
– limitTo – limits an array/string, into a specified number of elements/characters
– lowercase – format a string to lower case
– number – format a number to a string
– orderBy – orders an array by an expression
– uppercase – format a string to upper case
Adding filters to expressions is simple — we use the “|” char, and then we type the name of filter, for example: uppercase:
<div ng-app="myApp" ng-controller="personCtrl"> <p>The name is {{ lastName | uppercase }}</p> </div>
This filter changes letter to uppercase.
Adding filters to directives is also simple, and very useful:
<div ng-app="myApp" ng-controller="namesCtrl"> <ul> <li ng-repeat="x in names | orderBy:'country'"> {{ x.name + ', ' + x.country }} </li> </ul> </div>
In this example, we have added sorting filter to the ng-repeat directive. Yeah — sorting will be done by Angular!
Money, money, money and the “currency” filter
This filter will format a given number as currency:
<div ng-app="myApp" ng-controller="costCtrl"> <h3>Price: {{ price | currency }}</h3> </div>
And so on. We can format the date, JSON string, etc.
By the way about JSON, it’s worth to mention, that Angular has its won methods for handling the data in this format:
angular.fromJSON() – deserializes a JSON string
angular.toJSON() – serializes a JSON string
There is also the “Global API” available. It’s a set of global JavaScript functions, to execute typical tasks, such as:
– comparing objects
– iterations
– converting objects
More information you can find here:
http://www.w3schools.com/angular/angular_api.asp
And about the filters, more information and examples you can find here.
It’s not everything, because we can also define own filters. I recommend an interesting article with examples:
https://toddmotto.com/everything-about-custom-filters-in-angular-js
While the single “|” char is used to define filters, double “||” char defines a normal OR operator:
<p>{{ data.length > 0 && 'My data' || 'No data' }}</p>
Let’s take a look at the forms.
Forms and AngularJS
The framework supports the work with forms (data-binding and validation). Let’s consider a simple example.
HTML:
<div ng-app="myApp" ng-controller="formCtrl"> <form novalidate> First:<br> <input type="text" ng-model="user.firstName"><br> Last:<br> <input type="text" ng-model="user.lastName"> <br><br> <button ng-click="reset()">Reset</button> </form> <p>form = {{user}}</p> <p>master = {{master}}</p> </div>
JS:
var app = angular.module('myApp', []); app.controller('formCtrl', function($scope) { $scope.master = {firstName: "Donnie", lastName: "Brasco"}; $scope.reset = function() { $scope.user = angular.copy($scope.master); }; $scope.reset(); });
We use here well known data binding between model and the form elements.
More about forms in AngularJS:
http://www.w3schools.com/angular/angular_forms.asp
http://www.w3schools.com/angular/angular_select.asp
See also:
But let’s take a look at validation, which Angular supports in a brilliant way.
Forms validation with AngularJS
The form fields can be validated using Angular. Basically we are talking here about client-side validation. For full, secure validation perhaps you will need to connect it with server-side validation parts.
Example — required:
We use the HTML5 required attribute to define required field:
<form name="xForm"> <input name="xInput" ng-model="xInput" required> </form>
We can very easily inspect the state of this field in Angular:
<p>State:</p> <h1>{{xForm.xInput.$valid}}</h1>
The same works with other validators, for example email:
<form name="xForm"> <input name="xInput" ng-model="xInput" type="email"> </form> <p>State:</p> <h1>{{xForm.xInput.$valid}}</h1>
Error message
In this example a span element with error message will be displayed, if the expression from ng-show ($error) returns true:
<form ng-app="x" name="xForm"> Email: <input type="email" name="myAddress" ng-model="text"> <span ng-show="myForm.myAddress.$error.email"> Invalid e-mail address </span> </form>
More about validating forms with Angular:
http://www.w3schools.com/angular/angular_validation.asp
Angular includes
An another interesting possibilincity offered by AngularJS, includes. Thanks to them we can include HTML content from an external file.
For this we use the ng-include directive:
<div ng-app="x"> <div ng-include="'xfiles.htm'"></div> </div>
AngularsJS is helpful all fields.
Custom directives
AngularJS is great because of many reasons. One of them is ability to define own directives.
Definition in HTML element:
<div ng-app="xApp" x-my-directive></div>
Directive definition — JS code:
var app = angular.module("xApp", []); app.directive("xMyDirective", function() { return { template : "I'm a brand new directive!" }; });
More about directives: here.
Resources
– video courses — Angular
Learning AngularJS — summary
It’s was the 3rd part of basic AngularJS course. Certainly there is a lot of interesting topics to discover, like ajax, services, routing, etc. We will try to describe them as tutorials, where we will be creating some more advanced
examples of web projects.
ng-ThankYou!
Thank you for the article.
Great blog that I enjoyed reading.