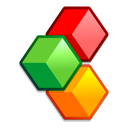
Welcome to the first part of the articles about object-oriented programming (OOP) in JavaScript. It is actually necessary to explore of the language secrets.
Object-oriented programming in JavaScript
JavaScript is an object-oriented language, although there is a lack of certain elements typical for object-oriented languages, at least in the current implementations of JavaScript.
But let’s get to the specifics.
Almost everything in JavaScript can be treated as an object (arrays, dates, strings, etc).
We can write either:
var text = "Hello World";
and:
var text = new String("Hello World");
In short, objects are data (specific type), which have properties and methods.
Properties are values associated with the objects, whereas methods are related actions (functions) that can be performed on those objects.
Examples of properties:
text.length; // get myCar.name = 'VW'; // set
Examples of methods:
text.indexOf("World"); myCar.drive();
Creating objects
Now we will create an object of a new type. We will do this by defining a function, where we implement the properties and methods for the objects of our new type.
Classes? I deliberately didn’t want to use the term. In typical object-oriented languages, class is something like a recipe for creating objects. Usually a class is defined using the class keyword.
As mentioned in basics of JavaScript, there is a reserved keyword class — for future compatibility.
Then would be possible for following “class”:
function Employee() { this.name = ""; this.dept = "IT"; }
could be written in Java style:
public class Employee { public String name; public String dept; public Employee() { this.name = ""; this.dept = "IT"; } }
But for now, let’s back to the current JavaScript language. Function shown below can be considered as a class (or rather the class equivalent). It is a way to simplify the course of thinking.
Example — definition of a function (“class”) and creating an object:
function niceCar() { // properties this.name = ''; this.speed = 0; // a method this.getSpeed = function() { return this.speed; }; // another way to write this.accelerate = Accelerate; function Accelerate() { this.speed++; } } var myCar = new niceCar(); myCar.name = 'VW'; alert(myCar.name); // VW alert(myCar.getSpeed()); // 0
Thus, it looks like the creation of a standard function: function myClass() {}.
The difference lies in the fact, that it will be called for the creation of a new object: the new operator.
Access to the members of the current class copy (an object with methods and properties) give us the this operator:
this.count = 128;
There are other ways of creating objects.
Example — new Object():
var uc = new Object(); uc.txt = 'Text'; uc.print = function() { alert(this.txt); } // calling uc.print();
Example — use the object literal (more information soon):
Warning = { Text: '', Run: function() { alert(this.Text); } } Warning.Text = 'Lorem ipsum!'; Warning.Run();
Tip
// a, b, c refers to the same (empty) object a = b = c = {};
Inheritance
In JavaScript, the equivalent of inheritance can be achieved using the prototype word:
// pointCounter may refer to a member of the class // pointParent(parent) pointCounter.prototype = new pointParent();
With the prototype we introduce members of the class, who will be valid for all objects, and also — inherited.
Thus, searching for a specific member, JavaScript searches the “class” as first, and then the prototype if needed.
Prototypes may extend built-in classes:
// enter a prototype 'leapYear' to the Date class Date.prototype.isLeapYear = isLeapYear;
The object literals
Object literal is enclosed in curly braces ({}) list of zero or more pairs (the name of object property and assigned value).
Example:
var bonus = "Air conditioning"; function carTypes(name) { if (name == "VW") return name; else return "Unavailable: " + name + "."; } auto = { myAuto: "Bora", getAuto: carTypes("VW"), extra: bonus } alert(auto.myAuto); // Bora alert(auto.getAuto); // VW alert(auto.extra); // Air conditioning
In addition, we can use a string or numeric literal as property names, or even make an object nested inside another object:
auto = { moreCars: {a: "Toyota", b: "Jeep"}, 8: "Subaru" } alert(auto.moreCars.b); // Jeep alert(auto[8]); // Subaru
Example — definition and use of the object and accessing items:
var flight = { airline: "LOT", number: 669, departure: { TRACE: "WARSAW", time: "2010-07-22 15:55", city: "Warszawa" }, arrival: { TRACE: "LA", time: "2010-07-23 11:42", city: "Los Angeles" } }; alert(flight["airline"]); // LOT alert(flight.departure.TRACE); // "WARSAW"
Another example shows how to add a method to the object.
Example — adding a new method to the object:
var myObject = { value: 0, increment: function (inc) { this.value += typeof inc === 'number' ? inc : 1; } };
And at the end we will see how to create the equivalent of a “constructor”. His instructions will be executed when creating a new object (the new operator).
Example — “constructor”:
var Foo = function (string) { this.status = string; }; // add public method get_status for all instances Foo.prototype.get_status = function() { return this.status; }; var myFoo = new Foo("Tested"); // an instance myFoo.get_status();
Bonus: debug of objects and the toSource() method
The toSource() is a method which represents the source code of the object. This method converts the object into a source form (text).
The constructor method is available for each object, and points to the object constructor.
Example — debugging object:
function myType(price, color) { this.price = price; this.color = color; } var obj = new myType(99, "green"); alert(obj.constructor); // #1 alert(obj.toSource()); // #2
Results:
// alert #1:
function myType(price, color) {
this.price = price;
this.color = color;
}
// alert #2:
({price:99, color:”green”})
Summary
That’s all in the first part of object-oriented programming in JavaScript.
Thank you for you attention.