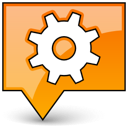
Part 2. of the OOP in JavaScript. Today we will show some more advanced examples.
OOP in JavaScript — techniques
For a good start, let’s take a closer look at arrays.
Arrays as objects
A simple array, e.g.:
var t = new Array("a","b","c");
can be written in a concise form:
var t = ["a","b","c"];
And certainly we can operate on arrays in the same way as we operate on other objects, by using methods and properties.
Examples of typical operations for arrays in JavaScript:
tab.push("x"); // adding an element alert(tab.pop()); // getting an element // deleting the element at a given index var index = 0; delete tab[index];
Associative arrays
Well-known in PHP, associative arrays can be created in JavaScript in create a fairly pleasant way:
var numbers_object = { '0': 'zero', '1': 'one', '2': 'two', '3': 'three', '4': 'four', '5': 'five', '6': 'six', '7': 'seven', '8': 'eight', '9': 'nine' }; alert(numbers_object[7]);
Objects management
I don’t mean that the Garbage Collector will be replaced by us, rather about the opportunities that we have, if we want to control our objects.
A basic example — deleting an object:
var foo = new Object(); delete foo;
Using the built-in operator delete, we can delete a component (property) of an object, array element, or even the whole object.
Fabrication of the objects (OOP factory)
A simple example of the objects factory (JavaScript OOP):
function createCar(sColor, iDoors) { var oTempCar = new Object(); oTempCar.color = sColor; oTempCar.doors = iDoors; oTempCar.showColor = function() { alert(this.color) }; return oTempCar; } var oCar1 = createCar("red", 4); oCar1.showColor(); // red
Copying objects
To copy an object, we must copy all the fields:
Object.prototype.clone = function() { var n = {}; for (p in this) { n[p] = this[p]; } return n; }
Once again: OOP in JavaScript through prototyping
JavaScript language creates objects based on prototypes. Each new object is a copy of the previously created template — a prototype, which is placed in the function.
Two examples below.
Example #1:
// a constructor for the NewClass "class" var NewClass = function() {} NewClass.prototype.hello = "hello world!" ; var k = new NewClass() alert(k.hello); // displays: "hello world!"
Example #2:
function Car() { } Car.prototype.color = "red"; Car.prototype.doors = 4; Car.prototype.drivers = new Array("John", "Sandra"); Car.prototype.showColor = function () { alert(this.color); }; var oCar1 = new Car(); oCar1.drivers.push("Tom"); alert(oCar1.drivers); // "John, Sandra, Tom"
Assignment of methods is done as follows:
object.method = function_name;
Removing properties it’s just:
delete object.color;
Further practical examples
A picture is worth a thousand words (String objects). A good example can give you more than a good description, so I hope that the examples below meet the task.
Example of stack implementation
Abstract data type such as a stack may be implemented as an array. JavaScript gives the corresponding functions (push, pop):
var stack = new Array(); stack.push("red"); stack.push("green"); alert(stack.toString()); // "red, green" var vItem = stack.pop(); alert(vItem); // "green" alert(stack.toString()); // "red"
Whoever has implemented own stack in e.g. C programming language, will appreciate the ease of this task using OOP in JavaScript.
And what if the methods already available, are not good enough, or we just want to write our own? No problem, because it is possible to …
… overwrite methods:
// our own pop() method: Array.method('pop', function ( ) { return this.splice(this.length - 1, 1)[0]; }); // overriding the push() method: Array.method('push', function () { this.splice.apply( this, [this.length, 0].concat( Array.prototype.slice.apply(arguments))); return this.length; });
Another example is actually trivial, but useful.
Example — object-oriented event handler:
<h1 id="myH1">Header…</h1>
function eventHandler() { alert("Event!"); } var myH1Object = document.getElementById("myH1"); myH1Object.onclick = eventHandler;
And in the next piece of code, we have a sample of typical OOP in JavaScript:
Example — implementation of the dictionary:
function universalTranslator() { this.copyright = "John Doe 2010"; } function universalCounter() { this.Count = count; var numbers = { "en": "one, two, three", "fr": "un, deux, trois", "de": "eins, zwei, drei" }; function count(language) { if (numbers[language]) { window.alert(numbers[language] + " [" + this.copyright + "]"); } else { alert("Unknown language"); } } } universalCounter.prototype = new universalTranslator(); var ul = new universalCounter(); ul.Count("de");
Summary
And that’s all in part two about OOP in JavaScript. We have described the key information you need to work with OOP in JavaScript, and also presented various examples of codes.
A whole bunch of new, interesting topics, coming soon.
For example: Math in JavaScript. This, and also OOP will be really useful, when we will start working around cool things, such as games programming.
Thank you for your attention.