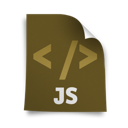
So far, we have described a number of different topics, and now it’s time for an article about forms in JavaScript.
Forms, especially in combination with CSS and JavaScript, are providing a strong foundation for development of UI elements to interact with the user.
Forms in JavaScript
Handling forms in JavaScript from scratch.
The form markup and document.form(s):
<form name="test"> <input name="el1" /> </form>
Access to the form object:
document.forms["test"];
Access to the field (element):
document.form[0].elements["el1"]; // another way document.test.el1;
Being inside of a form we can pass it to a function by this.form.
The checkbox input
<form name="myform"> <input type="checkbox" name="cb" id="cb" /> </form>
Example — handling checkbox field type:
alert(document.forms.myform.cb.checked); // or document.forms.myform.elements["cb"].checked ? "yes" : "no";
Radio buttons
var f = document.forms.myform; var btn = f.elements["radio"][0];
We get an access to btn.value and btn.checked.
Example:
<form name="myform1" action="index.php" method="get"> <div> <input type="radio" name="radio1" value="x1" /> <input type="radio" name="radio1" value="x2" /> </div> </form>
The radio1 property of this form points to a list of all the fields in the radio1 group, so the reference will look like this:
document.forms.myform1.radio1.length;
For each item in this group can be referenced using square brackets, e.g.:
document.forms.myform1.radio1[0]; // or through the item() method: var f1 = document.forms.myform1; f1.radio1.item(0);
So if you need to find out if the first field (index = 0) in the radio1 group in myform1 form is marked, you should write:
document.forms.myform1.radio1[0].checked // or document.forms.myform1.radio1.item(0).checked
Value — get and set value.
In general the case is simple: the value of fields can be obtained using .value property, for example:
document.forms[0].elements[1].value.
In a similar way, we can set (dynamically change) the value for the form field.
Example — setting the value of a form field in JavaScript:
document.forms['myform1'].username.value = 'John';
Selection list
<form name="myform1" action="index.php" method="get"> <div> <select name="list1" multiple> <option>first</option> <option>second</option> </select> </div> </form>
Following code gives index of selected item, or -1 if nothing selected:
var f = document.forms.myform; var i = f.elements["list1"].selectedIndex;
We get access to properties: value , text (the text), and options (an array).
Example — another way:
var x_s = document.forms.NameOfForm.myListName.selectedIndex; alert(x_s);
References to selection lists can be written in various formats:
document.forms.registerForm.elements["birthday[day]"].selectedIndex;
In modern browsers there is a shortcut to selected item:
var f = document.forms.myform; f.elements["myList"].value;
However, for maximum compatibility it is best to write:
f.elemetns["myList"].options[f.elements["myList"].selectedIndex].value;
The textarea
To the textarea type field called text1, we refer using the code:
document.forms.myform1.text1.value;
Disabled i readOnly
Objects representing the elements of the form (e.g. “text” input), can be turned off or set to “read only”, so that their modification would be impossible.
Setting disabled = true turns off this field, but also changes its appearance. This can be avoided by using the readOnly attribute.
Example:
<input type="text" name="firstname" id="firstname" /> <a href="javascript:turnOffTheField()">Click to turn off</a>
function turnOffTheField() { document.myform1.firstname.disabled = true; // document.myform1.firstname.readOnly = true; }
More about form objects
The total number of forms (in the document) is obtained by reading the property length:
var number_of_forms = document.forms.length;
An access to particular forms:
document.forms["form_name"] // or document.forms.form_name // or document.forms[form_index]
Properties of the Form object
– elements – an array containing elements of the form,
– length – a number of form elements,
– name – a value of the attribute name (form name),
– acceptCharset – attribute accept-charset, the list of supported code pages,
– action – value of the action parameter, specify where you want to send data from a form,
– enctype – the method of encoding data for the POST method,
– encoding
– method – mode of sending data to the server,
– target – a value for target parameter, e.g. determine the frame to which you want to send data.
Methods
– reset() – resets the values of form fields,
– submit() – sends the form.
Events
onreset – when the Reset button is pressed,
onsubmit – when the Submit button is pressed (send form).
Summary
That’s all when it comes to the basic processing of the form fields of various type in JavaScript, and the extraction of values.
In the near future we will describe support for forms using JS frameworks and excellent HTML5 forms.