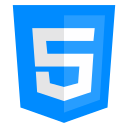
Introduction to HTML5 we start by one of the most important elements, namely HTML5 Canvas.
We’ll talk briefly about programming in JavaScript in the context of the Canvas element (and other elements in further articles).
HTML5 Canvas
HTML5 gives us many new opportunities, including the new Canvas element, which is part of the HTML5 specification.
This element is something like the environment to create dynamic graphics, rendering shapes and bitmap images, or graphics operations.
Graphics is created using JavaScript codes, and thanks to HTML5 Canvas became possible to create animations and games running in browsers, without additional plug-ins.
Let’s move on to the code. The following example shows a basic HTML5 document.
Example:
<!doctype html> <html> <head> <meta charset="utf-8"> <title>HTML5 Basics</title> </head> <body> <p>Hello HTML5</p> </body> </html>
Basics of the HTML5 Canvas
To create a Canvas element, we use the appropriate tag. And we should set the dimensions (default 300×150 px).
Example:
<canvas id="example" width="400" height="200"> This text will be displayed if the browser does not supports HTML5 Canvas. </canvas>
If we give some content between the opening and closing tags, this text will be displayed in browsers without HTML5 Canvas element support.
It’s practically everything when it comes to creating the same Canvas element in HTML5. The rest — proper drawing — is done in JavaScript.
HTML5 Canvas API
The first thing we should do is to refer to the Canvas element and its context (API):
var canvas = document.getElementById('test'); var context = canvas.getContext('2d');
And now we can draw in two-dimensional space of HTML5 Canvas.
It is also worth mentioning that coordinates in HTML5 Canvas start at the top left corner — the 0.0 point is here.
Running the example
We must have a browser that supports this technology, such as Google Chrome or newer versions of Firefox.
In truth, there is also a library html5.js helps to deal with the compatibility of HTML5 in IE:
<!--[if lt IE 9]> <script src="//html5shim.googlecode.com/svn/trunk/html5.js"></script> <![endif]-->
Canvas includes the area, where we can draw with a suitable API, available from JavaScript level.
In JavaScript, we refer to the object and retrieve for him the context in which we will be able to perform appropriate operations:
var test = document.getElementById('test'); var ctx = test.getContext('2d'); // blue color ctx.fillStyle = "rgb(0,0,255)"; // draw a square with set color ctx.fillRect(10, 10, 90, 90);
This is how it looks, and here is a complete example:
<!DOCTYPE html> <html lang="en"> <head> <meta charset="utf-8"> <script type="application/javascript"> function doDraw() { var canvas = document.getElementById("canvas"); var ctx = canvas.getContext("2d"); // rgb ctx.fillStyle = "rgb(0,0,200)"; ctx.fillRect (10, 10, 90, 90); // rgb + parameter alpha ctx.fillStyle = "rgba(200, 0, 0, 0.6)"; ctx.fillRect (20, 20, 90, 90); } </script> </head> <body onload="doDraw()"> <canvas id="canvas" width="250" height="250"></canvas> </body> </html>
Example available here.
Please note the typical for HTML5 sections DOCTYPE and JavaScript script type (application/javascript).
For the curious more interesting and instructive examples of HTML5 Canvas fundamentals Opera Developer Community.
Summary
Broach the subject of HTML5 Canvas is just a warm-up prior to future articles and projects, and for potentially a real revolution, which is WebGL.
In the next article we will try to present the course of HTML5 in a nutshell, discussing key issues of working with this technology. We will also use the “modernizr” library, to improve our work and solve the problems with compatibility.