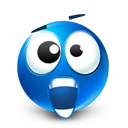
This article is a little different. Namely, we revise the strange elements of JavaScript. Sometimes is called JavaScript WTF 🙂 Some are just funny, others cause debugging a bit longer. So let’s remember them, when our code starts to do weird things.
JavaScript WTF
Certainly much depends on the browser (implementation of JavaScript). In newer versions of the browser, some peculiarities can be removed / handled in a particular way.
NaN is a Number?
Not a Number (NaN) is a Number — welcome in JavaScript.
alert(typeof NaN); // 'Number' alert(NaN === NaN); // false
To determine whether something is a number or not, simply use the isNaN() function.
Null is an object
alert(typeof null); // 'object'
“So what? In JavaScript everything is an object…”. Ok, but:
alert(null instanceof Object); // false
🙂
Functions can call themselves
Functions usually contain code to be executed at a specified time (calling the function). However, there is no blocking for code as below:
(function() { alert('hello'); })(); // 'hello'
So the function will be called and executed immediately.
0.1 + 0.2 equals 0.3 ?
Generally yes, but in JavaScript it’s 0.30000000000000004.
It’s about precision of floating point calculations. JavaScript implements it with a small oddity, which, however, can cause problems for developer working (especially comparing) with such numbers.
Tantra сексшоп интернет магазин.
Just as in the following code, which unfortunately returns false:
var num1 = 0.1, num2 = 0.2, cmp = 0.3; alert(num1 + num2 == cmp); // false
To avoid this, we can use for example a such workaround:
alert(num1 + num2 > cmp - 0.001 && num1 + num2 < cmp + 0.001); // true
OK, who explored issues of handling floating point implementation knows that it is really a complicated subject.
Equal is not always equal
For example:
1 == 1; // true 'foo' == 'foo'; // true [1,2,3] == [1,2,3]; // false
Comparison of arrays returns false. This is because, as in many other languages, operates in the references to the array, not the exact value.
But if we compare:
new Array(3) == ",,"; // true
it returns true. That’s because the chain representation of such an array is “,,”:
new Array(3).toString(); //",,"
JavaScript converts the expressions on both sides to a string when using the comparison operator ==.
Therefore, do not forget the === operator (compare value and type):
new Array(3) === ",,"; // false
“Arithmetic” of objects and arrays
Sadists like to bully JavaScript language, through using operators typical for numbers to operate on objects and arrays.
alert(1 * 'foo'); // NaN alert(1 * 2); // 2 alert('foo' * 1); // NaN alert('foo' + 1); // 'foo1'
Simple — by adding 1 to the string we get a combination of two strings (here: foo1). There is no multiplication for strings, thus rightly we get NaN.
Table plus object gives the object:
[] + {}; // [object Object]
but why such an operation gives the number 1 as a result?
alert( + ! + []); // 1
quirk? JavaScript WTF?
Let’s add functions and think how JavaScript conjured the string “42” as a result of the operation:
[]+(-~function(){}-~function(){}-~function(){}-~function(){}) + (-~function(){}-~function(){})
🙂
Positive and negative zero?
This may come from the IEEE 754, where is something like zero with the sign.
alert( (+0) + " " + (-0) ); // result 0 0
Clear — conversion to the string. So, let’s see how JavaScript compares with each other “positive” and “negative” zero:
alert((+0 === -0)); // true alert((-0 < +0)); // false
However, if we divide by 0, you get plus infinity:
alert((1 / 0)); // Infinity
but divided by the “minus” zero, gives minus infinity:
alert((1 / -0)); // -Infinity
Summary
For sure JavaScript has many peculiarities. But this don’t prevent this language reign in frontend development, and even enter new areas of application. Using this language intensively, learn us to live with these quirks.
As a consolation, articles such as this:
https://wiki.theory.org/YourLanguageSucks#JavaScript_sucks_because
show that not only JavaScript, but other languages have own quirks.