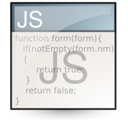
Welcome to the new article. We touch various topics, and now we feel obliged to write about JSON (JavaScript Object Notation).
It is a lightweight computer data interchange format. JSON is a text format, and you can treat it as a subset of JavaScript.
JSON format in JavaScript
The MIME type for JSON format is application/json. Due to the usefulness and convenience of use is often found in web (and even mobile) applications. JSON is often chosen instead of XML as a data carrier in applications using AJAX.
It is true that JSON is associated mainly with JavaScript, and allows to save entire objects of the language in its notation, however, this format is independent of any particular language.
Many other programming languages support the JSON format of the data by additional packages and libraries. These include ActionScript, C i C++, C#, Java, Perl, PHP, Python, Ruby, and others.
JSON is a great way to serialize the data.
On the official website of the JSON format, we can find a lot of information and tools, including libraries for many other programming languages.
A basic example — use JSON format in JavaScript:
var obj_txt = '{"firstname": "John", "lastname": "Doe", "addr":{'; obj_txt += '"street": "Ajax Street 77", "city": "Ajax City"}}'; var obj = eval("(" + obj_txt +")"); var str = "Data read from the JSON object:\n"; str += "Firstname: " + obj.firstname + "\n"; str += "Lastname: " + obj.lastname + "\n"; str += "Address - street: " + obj.addr.street + "\n"; str += "Address - city: " + obj.addr.city + "\n"; alert(str);
In a simple way we processed the data from the JSON object.
Example — use JSON with AJAX:
Paste the following content into the file books.json:
var book= { "title" : "JavaScript and HTML5 Tutorial", "author" : "John Doe", };
Usually we just send out such data in the HTTP request, but let’s assume that we have stored the JSON data in a file on the server.
Example — read the data in JSON format from a file (AJAX call):
// … AJAX.open("GET", "books.json"); var json = XMLHttp.responseText; var book = eval("(" + json + ")"); var title = book.title; // get data
JSONP
Let’s look at this piece of code (AJAX call using jQuery):
$.ajax({ url: tokenUrl, dataType: "jsonp", success: function(results) { … } });
Sometimes we can meet dataType: “jsonp” instead of json.
It’s about the “JSON with Padding“. This is the mechanism used for communication (e.g. loading data) from another domain (cross-domain request).
The JSON-js library and serialization
When we want to work with JSON, we can confidently choose the library written by Douglas Crockford.
JSON-js library inclusion:
<script type="text/javascript" src="//raw.github.com/douglascrockford/JSON-js/master/json2.js"></script>
We get a convenient tool for working with JSON in JavaScript.
Examples of the use of JSON.stringify:
// … data: JSON.stringify(response.data) // transform data from server //… JSON.stringify(true); // 'true' JSON.stringify("foo"); // '"foo"' JSON.stringify({ x: 77 }); // '{"x":77}'); JSON.stringify([2, "true", true]); // '[2,"true",true]');
By the way, analyzing the codes provided by Douglas on Github, we can learn something new about JavaScript.
With a language such as PHP, we can easily implement two-way communication, where the data carrier is of course JSON.
Example — communication with PHP:
// call example var myArr = [1, 77, 111]; var url = 'server_side.php?arr=' + JSON.stringify(myArr); // …
Service on the server side might look like this:
// server_side.php $myArr = array(); if (isset($_REQUEST['arr'])) { $myArr = json_decode($_REQUEST['arr']); }
The json_decode() function decodes the value passed as a string (JSON) into the data in correct format (in this case: an array).
From the other side, if for example we want to send some data to the JavaScript, we can simply encode them using json_encode().
Summary
JSON is without a doubt a very important format for exchanging data in the network.
It is an alternative to XML, and in many cases it becomes as preferred. I myself also choose JSON when possible, simply because for me working with the JSON format is better than, for instance with XML.
‘{“thank”:”you”}’
Nice article related:
http://www.w3resource.com/JSON/introduction.php
Something extra — JSON on-line editor!
http://jsoneditoronline.org/