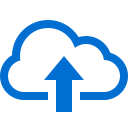
Update 09-2014 : note — this article has been originally created several years ago, so maybe a little different from today’s standards of good and recommended solutions.
Today we recommend the first part of best practices and solutions in JavaScript. It is purely practical. We present tips and simple tricks.
Best solutions in JavaScript
An example for a good start — adding a dynamic element to the document (use of DOM):
<div id="some_div">In this element we will add the next…</div>
// dom_create_input.js function createInput() { var input = document.createElement("input"); input.name = "user_location"; // field name input.id = "user_location"; // id input.value = ""; // default value input.style.width = "150px"; // CSS options // readonly, or other options … document.getElementById("some_div").appendChild(input); }
And that’s all.
Another trick is to send the form by clicking the link.
Example — send the form through plain link:
<a href="javascript:document.forms[0].submit()">OK</a>
How quickly set a CSS class for the element in pure JavaScript?
Here is an example:
var cn = "foobar"; document.getElementById("elem_id").className = cn;
Thus, we already set CSS class “foobar” for the element.
OK, and how to obtain the text content from an HTML element using DOM operations?
Example — getting text values from elements such as div, h1, etc:
<h1 id="topic"> Test </h1>
var dTopic = document.getElementById('topic').firstChild.nodeValue; alert(dTopic);
Let’s move on. How to write the simplest equivalent for the isset() function in JS?
Example:
function isset(variable) { if (variable == undefined) { return false; } return true; }
And here we have something better. It’s an implementation of isArray function. It’s a strict check whether the given argument is an array.
Example — checking whether the argument is an array in JavaScript:
// is_array JavaScript var isArray = function(value) { return value && typeof value === 'object' && typeof value.length === 'number' && typeof value.splice === 'function' && !(value.propertyIsEnumerable('length')); }; // examples of use: isArray('x'); // false isArray(1); // false isArray(new Array()); // true
And now, one of those simple tricks that I really like. It’s a concise and fast way to circumvent errors, such as when we don’t know if an item exists.
Such code tries to get the value of an item (in this case from an array), and returns either the value or the default value, which has been defined after OR logical operator (||).
Example:
var tab = new Array(); // … var myvar = tab["some_name"] || "(none)"; alert(myvar);
It just works, giving an elegant result, even if an element of the array (or object) doesn’t exist, and we’re trying to get a value from this element.
A little math, and regular expressions
By the way, I want to mention here about sharing of solutions. So if you know more best solutions and practices, tips and tricks, would be nice if you could post them as a comment below this article.
OK, let’s back to the code.
Example — factorial in JavaScript :
// factorial - JS var factorial = function factorial(i, a) { a = a || 1; if (i < 2) { return a; } return factorial(i - 1, a * i); }; alert(factorial(4)); // 24
Yes, we love maths.
OK, now we remind an example shown already when discussing regular expressions:
Example — parsing URLs:
var parse_url = /^(?:([A-Za-z]+):)?(\/{0,3})([0-9.\-A-Za-z]+)(?::(\d+))?(?:\/([^?#]*))?(?:\?([^#]*))?(?:#(.*))?$/; var url = "http://www.server.com:80/stuff?q#fragment"; var result = parse_url.exec(url); var names = ['url', 'protocol', 'slash', 'server', 'port', 'path', 'query', 'anchor']; var blanks = ' '; var i; for (i = 0; i < names.length; i += 1) { document.writeln(names[i] + ':' + blanks.substring( names[i].length), result[i]); }
Below we have an interesting and practical example about using regular expressions to format fractions as real numbers.
Example — format fractions as real numbers in JavaScript:
function fractions(txt) { txt = txt.replace(/^ */, ""); txt = txt.replace(/ *$/, ""); txt = txt.replace(/(([0-9]+) *\/ *([0-9]+)).*/, "($2/$3)"); txt = txt.replace(/ /, "+"); return(eval(txt)); } // examples of use alert(fractions("1/4")); // 0.25 alert(fractions(" 1 / 4 ")); // 0.25 alert(fractions(" 10 /80 ")); // 0.125 alert(fractions("2 3/8")); // 2.375 alert(fractions("1 2/5 cala")); // 1.4
The next example is a simulation of “varargs”, ie. variable list of function arguments, certainly well-known by programmers of languages such as C.
Example — variable list of arguments in JavaScript:
// va_args JS var sum = function() { var i, sum = 0; for (i = 0; i < arguments.length; i += 1) { sum += arguments[i]; } return sum; }; alert(sum(4, 8, 15, 16, 23, 42)); // 108
The numbers (variables of type Number) in JavaScript have native toString() method, which converts it to a string.
Example — toString for numbers (convert number to string in JavaScript):
var number = 5; alert(typeof number); // number number = number.toString(); // "5" alert(typeof number); // string // overriding the method (modified action) Number.prototype.toString = function() { return String(this + 1); }; var nv = number.toString(); alert(nv); // "6"
The code contains also an example of overriding the built-in method. In this case the number is increased by 1, and then converted to the String type.
And the last example for today — natural sorting in JavaScript.
Example — natural sort:
var m = ['aa', 'bb', 'a', 8, 4, 16, 15, 23, 42]; m.sort(function(a, b) { if (a === b) { return 0; } if (typeof a === typeof b) { return a < b ? -1 : 1; } return typeof a < typeof b ? -1 : 1; }); // m is now: [4, 8, 15, 16, 23, 42, 'a', 'aa', 'bb'] alert(m.toString());
Summary
And here we finish the first part of the series: Best solutions in JavaScript. Some of them were used to fix the problems encountered in real projects.
If any solution will be useful for at least one reader, I think the today’s job is done.
Thank you for your attention.
Nice stuff, thnx!
Also, near best solution is good to keep best practices, like:
http://code.tutsplus.com/tutorials/24-javascript-best-practices-for-beginners–net-5399