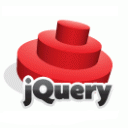
Here we have the 3rd part of jQuery course.
Further exploring of jQuery possibilities
Today we briefly describe programming Ajax elements, using jQuery.
We show also some interesting examples and tips jQuery, and finally mention about jQuery UI.
The jQuery gives us a high level of abstraction, with a rich support for Ajax. So the programmer is is able to make final effect with a small amount of code.
A basic example — load file content:
$(document).ready(function() { $('#content').load('lorem.html'); });
To work with Ajax in jQuery, we use main methods: $.post(), $.get(), $.ajax().
Example — sending data to the server using the POST method and a simple callback:
$.post('save.cgi', { text: 'my string', number: 23 }, function() { alert('Your data has been saved.'); });
Example — getting data using $.get():
$('#letter-e a').click(function() { $.get('e.php', {'term': $(this).text()}, function(data) { $('#dictionary').html(data); }); return false; });
Serialization and send the form data
Consider the simple form:
<div id="letter-f"> <h3>Form sample</h3> <form action="plik.php"> <input type="text" name="term" value="" id="term" /> <input type="submit" name="search" value="search" id="search" /> </form> </div>
By using the serialize() method, we get quick access to all the values of form fields. Dispatch is just as easy thanks to the method submit().
Example:
function doSubmit() { var str = $("form").serialize(); // alert(text(str)); $("form").submit(); }
Indicator (loader) in jQuery
In a simple way, we can implement a popular thing, which is showing that the Ajax processing is in progress.
Example — dynamic load indicator:
$('<div id="loading">Loading…</div>') .insertBefore('#dictionary') .ajaxStart(function() { $(this).show(); }).ajaxStop(function() { $(this).hide(); });
The details about programming Ajax with jQuery we’ll find in the documentation.
There is also a rich collection of Ajax plugins.
Now we get to the examples, solutions and tricks in jQuery, which can facilitate the daily work.
jQuery — tips and tricks
Example — set CSS style to all ‘label’ elements with attribute ‘for’:
$('label[@for]').css({'font-weight': 'bold'});
Example — working with form elements of type checkbox:
$('input:checkbox').bind('click', function() { if ($(this).is(':checked')) { $(this).parent().css({'font-weight': 'bold'}); } else { $(this).parent().css({'font-weight': 'normal'}); } });
Example — implementing simple tool-tips:
JavaScript
var $tooltip = $('<div id="tooltip"></div>').appendTo('body'); var positionTooltip = function(event) { var tPosX = event.pageX; var tPosY = event.pageY + 20; $tooltip.css({top: tPosY, left: tPosX}); }; var showTooltip = function(event) { var authorName = $(this).text(); $tooltip .text('Highlight articles of ' + authorName).show(); positionTooltip(event); };
CSS
#tooltip { position: absolute; z-index: 2; background: #efd; border: 1px solid #ccc; padding: 3px; }
Usage in code:
var hideTooltip = function() { $tooltip.hide(); }; foobar.hover(showTooltip, hideTooltip). mousemove(positionTooltip);
Example — implementation of the suggest component with Autocompleter.
First, the PHP code that returns data:
// server-side code - autocomplete.php if (strlen($_REQUEST['search-text']) < 1) { print '[]'; exit; } $terms = array( 'access', 'action', // … 'xaml', 'xoops', ); $possibilities = array(); foreach ($terms as $term) { if (strpos($term, strtolower($_REQUEST['search-text'])) === 0) { $possibilities[] = "'". str_replace( "'", "\'", $term)."'"; } } print ('['. implode(', ', $possibilities) .']'); // note: we can simply use the json_encode() function ?>
JavaScript code:
$(document).ready(function() { var $autocomplete = $('<ul></ul>') .hide() .insertAfter('#search-text'); $('#search-text').keyup(function() { $.ajax({ 'url': '../search/autocomplete.php', 'data': {'search-text': $('#search-text').val()}, 'dataType': 'json', 'type': 'GET', 'success': function(data) { if (data.length) { $autocomplete.empty(); $.each(data, function(index, term) { $('<li></li>').text(term). appendTo($autocomplete); }); $autocomplete.show(); } } }); }); });
At the end of the basic jQuery course, we present a little closer to perfect extension for the creation and use of rich user interface elements. It’s about jQuery UI solution.
This extension offers a large number of facilities, such as:
– interactions: Drag & Drop, Resizable (scaling elements), Sortable (sorting)
– widgets: Accordion, Autocomplete, Button, Datepicker, Dialog, Progress bar, Slider, Tabs
– utilities and lots of visual effects
Examples — how the widgets looks:
Then there are interchangeable — through CSS — skins for UI components.
Summary
We presented the basics of jQuery, to easy use this framework in normal work. It is good to have this information at hand. Sometimes we need to refresh the knowledge about something.
Many of future articles and tutorials that we create here, will be done using jQuery.
Thank you.