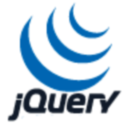
Here we go with the second part of jQuery basics, in which we describe the visual effects, DOM operations and events handling using this library.
Getting deeper in jQuery
Let’s start with the basics of visual effects.
There are main methods for elements visibility:
show() — causes dynamic showing of hidden element. As arguments can take a speed and the callback function:
show( speed, callback )
hide() — hiding an element:
hide( speed, callback )
toggle() — toggle the visibility of the element: show — if hidden, hide — when visible:
toggle( switch )
Example — use the toggle() method on the button element:
var flip = 0; $("button").click(function () { $("p").toggle( flip++ % 2 == 0 ); });
Folding and unfolding the element
These methods are able to change the visibility of an item, and they do it with animation (winding / unwinding):
slideDown( speed, callback )
slideUp( speed, callback )
Fading — transitions
In this case there is an animation of appearance / disappearance:
fadeIn( speed, callback )
fadeOut( speed, callback )
Change the style of element
We can dynamically set the element’s style, using methods: css(), addClass() and removeClass().
Examples:
$(this).css('color', 'yellow'); // set the color property $(this).addClass('inny'); // add the CSS class $(this).removeClass('inny'); // remove class
Example #2 — iteration:
$(document).ready(function(){ // every li element will be blue $('li').css('color', 'blue'); });
Cascade
We know this already from other frameworks. Many methods can be linked together in a chain.
Example:
$('li').css('color', 'yellow').append('- read more') .css('background', 'black'); // of course we can call this as 3 separate instructions
Events and jQuery
Another very strong point of jQuery is the event handler.
Example — shwo and alter after clicking on a paragraph:
$(document).ready(function() { $('p').click(function() { alert('Hello'); }); });
Handling of double click we obtain by:
$('p').dblclick(function(){…});
Mouse
jQuery also has handling of mouse. Methods such as .mouseout(), .mouseOver(), and much more, are described in documentation.
Removing event handler we execute by unbind():
$('#switcher-narrow, #switcher-large').click(function() { $('#switcher').unbind('click'); });
Keyboard
Keyboard support with jQuery is completely painless.
Example:
$(document).ready(function() { $(document).keyup(function(event) { switch (String.fromCharCode(event.keyCode)) { case 'D': $('#switcher-default').click(); break; case 'N': $('#switcher-narrow').click(); break; case 'L': $('#switcher-large').click(); break; } }); });
Animations — examples
Basically, animation support in jQuery looks like this:
Описание Derila anatomski jastuci od memorijske pjene на сайте.
.animate({property1: 'value1', property2: 'value2'}, speed, easing, function() { alert('The animation is finished.'); } );
For example — growing item:
$('#grow').animate({ height: 500, width: 500 }, "slow", function() { alert('Element has grown!'); });
Animation speed is defined in the second parameter, in this case it’s “slow”.
Example — positioning the elements with animation effect:
$(document).ready(function() { $('div.label').click(function() { var paraWidth = $('div.speech p').outerWidth(); var $switcher = $(this).parent(); var switcherWidth = $switcher.outerWidth(); $switcher .animate({left: paraWidth - switcherWidth},'slow') .animate({height: '+=20px'}, 'slow') .animate({borderWidth: '5px'}, 'slow'); }); });
DOM and jQuery
How can we expect, jQuery provides us powerful tools to work the DOM.
Example — when the DOM is ready, the second paragraph of the document (index 1) will be hidden:
$(document).ready(function() { $('p:eq(1)').hide(); });
Example — dynamic content:
$(document).ready(function() { $('p:eq(1)').hide(); $('a.more').click(function() { $('p:eq(1)').show(); $(this).hide(); return false; }); });
Example — accessing DOM elements:
var myTag = $('#my-element')[0].tagName;
Example — DOM and manipulation of form:
$('form#login') // hide all labels having the 'optional' CSS class .find('label.optional').hide().end() // add red frame for input elements of type 'password' .find('input:password').css('border', '1px solid red').end() // handle submit action .submit(function() { return confirm('Are you sure you want to submit?'); });
Inserting a new element to the DOM — insertXXX() methods
Example — insert a link at the end of the article:
$(document).ready(function() { $('<a href="#top">back to top</a>') .insertAfter('div.article p'); $('<a id="top"></a>'); });
The insertAfter() method — inserts after the element. To insert before we will use the insertBefore() method.
There is another approach — adding to the element: prependXXX.
Example:
$('<a id="top" name="top"></a>').prependTo('body');
Wrapping elements, so embedding an element within a specific HTML code
Example:
.wrap('<li id="foot-note-' + (index+1) + '"></li>');
Copying items we can perform by the method clone(), e.g.:
// this code will create a copy of the first paragraph in // the DIV with "chapter" CSS class $('div.chapter p:eq(0)').clone(); // Example #2 $('div.chapter p:eq(0)').clone().insertBefore('div.chapter');
Methods to manipulate the DOM — summary
1. Create a new element in the structure:
the $() method
2. Inserting elements inside the element:
.append(), .appendTo(), .prepend(), .prependTo()
3. Inserting new elements next to the element:
.after(), .insertAfter(), .before(), .insertBefore()
4. Insert new HTML elements around the indicated elements — wrapping:
.wrap(), .wrapAll(), .wrapInner()
5. Changing the elements or text contained therein:
.html(), .text(), .replaceAll(), .replaceWith()
6. Remove items from the interior of the specified element:
.empty()
7. Deleting a particular element and its descendants from the document:
.remove()
Summary
We described how to do the deal with event handling, effects and animations. And with short summary of DOM methods, we finish the 2nd part of basic jQuery course.
Thank you.