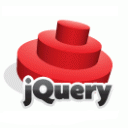
And now it’s time for jQuery!
jQuery course — basics of framework
Welcome to the 1st part of basic jQuery course. We talked already about various JavaScript frameworks (such as Prototype JS or MooTools).
Now we want to describe the key topics of a giant — jQuery.
Speaking giant, we mean the possibilities offered by this library. And for us, jQuery is preferred JavaScript / Ajax library.
The reason is simple — jQuery is popular and high-quality library, with extremely huge opportunities. If something is not offered by jQuery, probably there is at least one (usually much more) jQuery plugin(s).
Of course we can also create own plugins and publish them. In addition, jQuery has an excellent extension (jQuery UI). It is used to create and manage rich user interfaces for web applications.
Using jQuery in the project
Download the library from homepage.
It can also be added using CDN. In this example will always be included in the latest version:
<script src="//code.jquery.com/jquery-latest.js"></script>
We start with an important issue, which is noConflict.
Sometimes (fortunately perhaps less frequently than in the past) that the project uses several JavaScript libraries. And in many of them, as in jQuery, we can find e.g. the $() function. In such a case there is a threat of the conflict.
To avoid such a situation, jQuery introduces a method called noConflict (), which allows us to assign the full power of the $() to a variable, and wrap it separately.
Example:
var jq = jQuery.noConflict();
We can also insert code based on jQuery among another, e.g. based on Prototype JS, in a following way:
jQuery(document).ready(function($) { // here you can use $ of jQuery … });
The extreme parameter — jQuery.noConflict(extreme) — the method has boolean parameter (extreme). In short, this parameter forces the assignment of $() functions to its original owners.
Example:
var dom = {}; dom.query = jQuery.noConflict(true);
JQuery selectors in a nutshell
JQuery gives us a set of powerful and useful selectors for elements.
Consider a practical example.
Retrieving an element of the type (the name as a parameter):
// $('p').click(function(){ … }); // add click handler to all paragraphs $('p').click(function() { alert('Click'); });
Retrieving an element by ID (# as a prefix):
// get element with ID = 'content' $('#content').click(function() { alert('Click click'); });
Getting elements by CSS class (a dot as a prefix):
$('.niceClass').click(function() { alert('Get by CSS class'); });
Retrieving by the value of an attribute. We can retrieve the elements by the value of any attribute.
E.g. for :
<a href="ipsum.html">ipsum</a>
we write:
$('a[href="ipsum.html"]').click(function() { alert('You clicked ipsum.html'); });
Child combinator:
// add the class for li elements of the 'selected-uls' parent $(document).ready(function() { $('#selected-uls > li'). addClass('horizontal-foobar'); });
Negation — not:
$('#selected-uls li:not(.horiz)').addClass('sub-level');
The specified condition will be negated.
Practical example — adding a CSS class to the link, depending on the file extension, which is pointing to:
$('a[href$=.pdf]').addClass('pdflink'); $('a[href$=.doc]').addClass('mswordlink'); // …
Selectors used to style tables
The framework equips the developer in many selectors to simplify many operations, such as styling elements of the tables.
Example:
// odd - styling every other row $(document).ready(function() { $('tr:odd').addClass('alt-1'); }); // even $('tr:nth-child(even)').addClass('alt-2');
Let’s meet further operations on the tables.
Contains — determine whether the element contains the text.
Example — add a class depending on the content:
// all rows with 'Henry' word $('td:contains(Henry)').addClass('highlight');
Getting and setting element values
For both tasks the is the val() method. If no argument — jQuery executes the get() method, and will set the value specified as a parameter otherwise.
Examples:
// get element value var myValue = $('#myDivId').val(); // set values for element $('#myDivId').val("hello world");
The is() method
This is the additional range of possibilities. The is() method allows us to specify, among others, if an element has a CSS class, attribute, etc.
Examples:
// has the 'pretty' class? if ($('#myDiv').is('.pretty')) { alert('Yes'); } // is the element hidden? if ($('#myDiv').is(':hidden')) { alert('Yes - hidden'); } // :hidden vs :visible var isVisible = $('#myDiv').is(':visible'); var isHidden = $('#myDiv').is(':hidden');
Disable / enable
With jQuery, we can easily set the item on enabled or disabled. For this purpose, there are methods: attr(‘attribute’, ‘value’) and removeAttr(‘attribute’);
Examples:
// disable $("#x").attr("disabled", "disabled"); // enable $("#x").removeAttr("disabled");
With the same methods, we can handle form elements.
Example — check / uncheck the checkbox element:
// assuming that we have a checkbox element with id = 'c' $("#c").attr("checked", "checked"); // set checked $("#c").removeAttr("checked"); // remove checked state
Example #2 — handling selection lists in jQuery:
// get id of selected item $("select#myselect").val(); // get the text (text() method) of selected item $("#myselect option:selected").text();
Step further — forms selectors
So quick access to specific elements of a given type, eg :text refers to <input type=”text”>
And in addition to that we have:
:text
:checkbox
:radio
:image
:submit
:reset
:password
:file
Buttons can also refer to the type of ‘button’:
:button
Active elements of the form (enabled):
:enabled
Inactive (disabled):
:disabled
Checked (radio buttons or checkbox):
:checked
Selected (for selection lists):
:selected
Extensive documentation of selectors and more examples we find here.
Accessor methods and basic operations on objects in jQuery
– size() — the number of elements:
$(document.body).click(function () { $(document.body).append($("<div>")); var n = $("div").size(); $("span").text("There are " + n + " divs." + "Click to add more.");}).click();
– length() — similarly to size():
$(document.body).click(function () { $(document.body).append($("<div>")); var n = $("div").length; $("span").text("There are " + n + " divs." + "Click to add more."); }).trigger('click'); // trigger the click to start
– context() — context of the DOM element passed to jQuery():
$("ul") .append("<li>" + $("ul").context + "</li>") .append("<li>" + $("ul", document.body).context.nodeName + "</li>");
– eq() — fast and effective method for comparing, for example:
// whether the 1st paragraph has set the color to red $("p").eq(1).css("color", "red");
– get() — getting elements:
function disp(divs) { var a = []; for (var i = 0; i < divs.length; i++) { a.push(divs[i].innerHTML); } $("span").text(a.join(" ")); } disp($("div").get().reverse());
– get(index) — getting an element with a specified index:
$("*", document.body).click(function (e) { e.stopPropagation(); var domEl = $(this).get(0); $("span:first").text("Clicked on - " + domEl.tagName); });
– queue() — easy implementation of the queue
Example of queuing:
$("#show").click(function () { var n = $("div").queue("fx"); $("span").text("Queue length is: " + n.length); });
Extending jQuery — the extend method
At this point, we only want to mention an excellent way of expanding jQuery.
In a simple way, we can introduce new methods. We can use this in order to write a typical jQuery plugin.
Example:
// jQuery.fn.extend( object ) jQuery.fn.extend({ check: function() { return this.each(function() { this.checked = true; }); }, uncheck: function() { return this.each(function() { this.checked = false; }); } });
Example — extending the jQuery:
// jQuery.extend( object ) jQuery.extend({ min: function(a, b) { return a < b ? a : b; }, max: function(a, b) { return a > b ? a : b; } });
We will write more about extending jQuery later, in further articles.
Summary
The first part of the course jQuery comes to an end. We presented a handful of examples, and issues. But this is just the tip of the iceberg.
We invite to further exploring of jQuery in the 2nd and 3rd part of the basic course.
$(“thank_you”).show(); // !