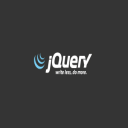
Welcome to the next article of the jQuery mini tips series. Today we will play around selected aspects of working with the form elements.
1. Prevent to select more than one item in select multiple input
Once I created a project, where I had to show UI in a little bit old-school way (similar to older desktop apps). Some of inputs had to be displayed as select multiple lists, but selecting more than one option was forbidden.
Fortunately no need to code any handlers in JS. With jQuery it’s a trivial thing:
// allow only one item to select $('#my-options').removeAttr('multiple');
In that way we disable the possibility of selecting more than one option, but our element still remains displayed as select multiple.
2. How to get ID and values of selected option in such input?
It’s simple to write, and can be done in many different ways. I prefer this one (using jQuery):
$("#my-items").on("click", function() { var val = $("#my-items option:selected").val(); var text = $("#my-items option:selected").text(); // … });
It works, even in IE.
Now let’s take a look how simply we can implement a functionality of transferring options across two select-multiple inputs (selecting more than one item enabled), in two directions.
3. How to transfer the options between select multiple inputs?
Let’s assume we have two such inputs next to each other. On the left side we have e.g. categories to choose, on the right — categories to block (blacklist). Selecting / blocking items is done quickly through moving items to the appropriate input element.
Moved options from the element on the left side are disappearing from this element, and going to the element on the right side. And vice-versa.
So having two inputs (with IDs “include” and “exclude”) and two buttons between (e.g. arrows with IDs “left-btn” and “right-btn”), which initiate the transfer of selected options, we can write:
// move from left to right $('#left-btn').click(function() { !$('#include option:selected').remove().appendTo('#exclude'); reArrangeRight(); return; }); // move from right to left $('#right-btn').click(function() { !$('#exclude option:selected').remove().appendTo('#include'); reArrangeLeft(); return; });
Please also take a look at reArrange functions. They have been added, as it’s worthwhile to sort the options, and display them alphabetically, with no clutter.
Here is the implementation of these functions — we use the sort() method:
function reArrangeLeft() { $("#include").html($("#include option").sort(function(a, b) { return a.text === b.text ? 0 : a.text < b.text ? -1 : 1; })); } function reArrangeRight() { $("#exclude").html($("#exclude option").sort(function(a, b) { return a.text === b.text ? 0 : a.text < b.text ? -1 : 1; })); }
After the call, these functions will sort the items in the select-multiple input.
4. Get all checked check-boxes
https://www.instagram.com/distinctivetravelscom
This solution shows how to retrieve all the selected checkboxes in the specified item, e.g. div:
var active = $("#my-div input[class=xyz]:checked").map(function() { return this.id; }).get().join(",");
In this case all the checkboxes we want to process, must be with the “xyz” CSS class added. The code returns the ID values of each checked element, as comma-separated string (join() method). Such a string we can for example send via Ajax and process.
Take a look also on previous articles with jQuery mini tips:
https://javascript-html5-tutorial.com/javascript-and-jquery-mini-tips.html
https://javascript-html5-tutorial.com/javascript-and-jquery-mini-tips-2.html
Have fun with jQ!
Check if multiple select has option selected =>
var ts = $(“select[name=’tasks[]’] option:selected”).length;
if (ts < 1) {
alert("Please select at least 1 Task!");
return false;
}