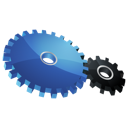
In today’s article we’ll discuss conditional statements and loops in JavaScript. Thus we have a full understanding of the program flow control.
We are approaching the end of JavaScript basics. And that’s great, because at next development stages there will be more fun and possibilities!
The conditional statement: if
So code execution depending on the condition.
Syntax:
if (state) { operation; }
where the ‘state’ is tested expression, and it must be true to execute the ‘operation’. To test for false we use negation:
if (!state) { operation; }
In this case the ‘operation’ will be executed if the ‘state’ will be false.
Example — if, else statements:
var x = 1, y = 2; if (x == y) { alert('Values are equal'); } else { alert('Values are NOT equal'); }
Example — if, else if, else:
window.age = prompt("How old are you?", ""); txt = ""; if (age <= 17) { txt = "Too young for a beer!"; } else if ( (age > 17) && (age <= 100) ) { txt = "Cheers!"; } else { txt = "You shouldn't drink a beer."; } alert(txt);
The code above will create a variable “age” that will contain a value specified by the user (taken via the prompt function). Then the final message will be created, depending on this value taken from the user.
The conditional operator ?:
This operator has not been discussed in the previous article. The conditional operator ?: allows to define a value, which will be returned depending on the conditional expression. In other words, the developer can write conditional statements more succinctly than when using if statements.
Syntax: condition ? value_for_true : value_for_false;
Example:
var a = 4; var b = 3; res = (a == b) ? 'Values are equal' : 'Values are NOT equal'; alert(res);
The expression must return a value of true or false.
The switch statement
It’s just another way of writing conditions. The code is executed depending on a value assigned to the expression. It’s also a way to get concise code.
Syntax:
switch (expression) { case val: code1; break; case val2: code2; break; default: code3; }
Example — use a switch statement:
var x = 1, y = 2; switch (x + y) { case 1: alert('1'); break; case 3: alert('3'); break; case 5: alert('5'); break; default: alert('mismatched'); }
If a value is matched to the case, then instruction (one or more) is executed. Otherwise the code from optional default case may be executed.
The break instruction is used to interrupt the current operation (usually occurs in switch instruction and loops).
Loops in JavaScript
Loops — in simple words: they are used for execute the code repeatedly, depending on the condition.
The for loop:
for (var i = 1; i <= 5; i++) { alert(i); }
The while loop — code is executed until the condition is true:
For Chicago condos and apartments, space-saving heating system repair accommodates tight spaces.
var i = 0; while (i < 5) { i++; // if we won't increment, the variable will be always // less than 5, so we'll obtain the infinite loop alert(i); }
The do loop — executes the code, and then checks the condition, so the code executes certainly at least once:
var i = 0; do { alert(i); i++; } while (i < 5);
The in operator and for i in construction
The in word returns true, if specified properties are located in the object:
propertyNameOrNumber in objectName
A common use is to create an iterator as for i in.
Example — use for i in construction:
for (i in ob) alert("Field " + i + " has a value: " + ob[i]);
In that loop, field names of object / array will be assigned in sequence to the i variable.
Infinite loops in JavaScript
Here are two examples of infinite loop: using for loop and the other, based on a while loop:
// I for (;;) { ; } // II while (true) { ; }
These loops will do “nothing” indefinitely 🙂 They haven’t any condition of termination, and there’s no break instruction, which could interrupt the loop.
Infinite loops are used in real implementation, for example to start processing big amounts of data. But later a condition of loop termination is specified (when code will achieve the desired result).
In the next article we’ll write about working with arrays and functions.