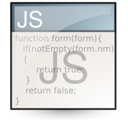
Welcome to the next article about JavaScript basics. Today we’ll focus on very important topics, such as data types and operators in JavaScript.
Operators in JavaScript
They serve the programmer to perform basic operations on variables, like assignment, arithmetic, comparison, and more.
Arithmetic operators
This looks the same as in other programming languages:
+ (addition)
– (subtraction)
/ (division)
* (multiplication)
% (modulo division — the division remainder)
++ (incrementation — increase of 1)
– – (decrementation — decrease of 1)
So that is basic math.
Example — arithmetic operations in JavaScript:
var x = 2, y = 3, result = 0; result = x + y; alert('2+3 = ' + result); result = x / y; alert('2/3 = ' + result); result = x % y; alert('2%3 to: ' + result); y++; // y--; alert(y);
2/3 is 0.66… and here we can see the essence of data types. If the result would be assigned to int (integer) variable, we would obtain 0. However, typing is done by JavaScript (dynamic typing).
Logical operators
They are used to express the logic conditions:
! — negation of logical value
&& — conjunction (“and”)
|| — logical alternative (“or”)
For example:
if (!a) { // negation - NOT operator } if (a > 0 && b > 0) { // conjunction - AND operator, both conditions must be true } if (a >= 1 || b >= 1) { // alternative - OR, at least one of conditions must be true }
Comparison operators
As the name suggests, they are used to compare the values:
== (equal)
=== (identical; not only values, but also data types are compared; we can find this operator also in PHP)
!= (different)
!== (not identical)
< (less than)
<= (less than or equal)
> (greater than)
>= (greater than or equal)
Usage already shown in the previous example.
Bitwise operators
They are used to operate on bits.
Bitwise operators are: ~, &, |, ^, <<,>>,
and >>> i.e. unsigned right shift.
Note: bitwise operators we will describe in details in one of further articles.
Assignment operators
So, how to assign values to variables:
= (assigning values)
+= (increasing the value of a variable and assigning it to the variable on the left)
-= (decreasing the value of a variable and assigning it to the variable on the left)
*= (multiplying the value of a variable and assigning it to a variable on the left)
/= %= — dividing (and modulo dividing) value of a variable and assigning it to a variable on the left.
Other operators in JavaScript
The language provides more operators:
, (comma — separating arguments)
. (dot — access to a property or method of an object: object_name.element)
new (creating a new object)
delete (deleting an object, particular element of array, etc)
?: (conditional operator — we will write more about it in the next article)
typeof the_name (returns the text name of the operand, specifying its type)
As we are around typeof topic, let’s talk about data types.
Data types in JavaScript
The language gives us a possibility of working on following, basic data types:
– numbers (Number)
– strings (String)
– boolean values — true and false (Boolean)
– objects (Object)
– arrays (Array)
– and special: null and undefined
Declarations of variables
Variables can be declared immediately prior to use anywhere in the script. And this can be done in several ways, as shown in the example below:
var text; // declare a variable named 'text', now its value is null // declaration with setting the value var text = "John"; var my_number = 123; // by creating a new object of a given type var str = new String("New text string");
Text can be placed in single or double quotes.
When a value of the variable can’t be determined (for example in case of division by 0), the returned result will be infinity.
The type of variable after its initial declaration is automatically determined.
The operator typeof(my_variable) returns a type of variable given in parentheses:
document.write(typeof(true)); // boolean
In this example, we check if given name is the name of a function or not:
if (typeof foobar !== 'function') { alert("foobar is not a function"); }
We can also easily check the type of object component:
// returns the name of component type alert(typeof object_name.element_name);
Additional functions for working with types
The isNaN() function checks whether the argument doesn’t contain the NaN value (Not a Number).
Therefore:
isNaN(25) — returns false (25 is a number)
var a1 = “x”;
isNaN(a1) — returns true (argument is not a number )
In addition, we have (very useful in some situations) functions, which allow us to extract numeric values (Integer or Float) from expressions, for example:
parseInt(5e1) — returns 5
parseInt(-21a) — returns -21
parseFloat(-2.3) — returns -2.3
parseFloat(-12.1+5) — returns -12
Literals and the \u — express it in Unicode!
We can use literals. And JavaScript provides a special notation for expressing characters.
Examples:
alert("A" === "\u0041"); alert('\u0041'); alert('\u0042'); // B
The eval() function
This function evaluates JavaScript code represented as a string. The eval() function evaluates the expression passed in argument, and tries to execute JavaScript statement(s).
Hardware complex for automated deposit machine in banking
Example — use the function eval():
// returns String object containing value "2+4" eval(new String("2+4")); eval("2+4"); // returns 6 my_var = "10+2*4"; // my_var2 will be a number variable, with a value of 18 my_var2 = eval(my_var);
Moreover, there is an operator void, which defines the expression to be evaluated without returning a value. In other words it performs the specified expression, and returns undefined.
Example — creating a link to some action in code:
<a href="javascript:void(some_function())"></a>
Global methods and properties
Short summary about global properties and methods in JavaScript.
Properties:
– Infinity
– NaN (Not A Number)
– undefined
Methods:
– encodeURI
– decodeURI
– encodeURIComponent
– decodeURIComponent
– eval
– isFinite (returns false when a value is: +infinity, -infinity or NaN)
– isNaN
and also: parseInt and parseFloat, mentioned above.
Summary
And we finished the 3rd part of JavaScript basics. In the next part we take a look closer on conditional statements, loops, so in general: program flow control.
Thank you for your attention.