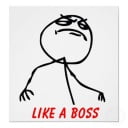
Bitwise operators in JavaScript
Today we will try to present bitwise operators in JavaScript language, in an accessible form.
The overall concept of the bitwise operators’ work in JavaScript is as follows:
– arguments are converted to 32-bit integer and expressed by a sequence of bits,
– each bit in the first argument is matched to the corresponding bit in the second operand,
– operator is used for each pair of bits, and the result at this level is bitwise.
Among the bitwise operations we distinguish:
– bitwise product (and, operator &),
– bitwise sum (or, operator |),
– negation (not, operator ~),
– symmetric difference (xor, operator ^).
We can see similarities to the logical operators.
The difference is that bitwise operators works not on logical values, or even on the values directly, but at lower level — on the individual bits of a number.
Bitwise operators treat their arguments as a set of 32 bits (ones and zeros), and not as decimal or hexadecimal.
For example, a binary representation of the decimal number of 10 is 1010.
Bitwise operators perform their operations on such binary representations, but they return standard JavaScript numerical values.
JavaScript bitwise operators — a cheatsheet
AND | a & b | Only 1 and 1 gives a score of 1, the rest 0 |
OR | a | b | 1 anywhere gives a score of 1, 0 and 0 gives the result 0 |
XOR | a ^ b | Returns 1 in a position where only one of the arguments is equal 1, 1 and 1, 0 and 0 gives 0 |
NOT | ~ a | Reverses (negate) bit operand |
<< | a << b | Bitwise left shift |
>> | a>> b | Bitwise right shift |
>>> | a>>> b | Zero-fill right shift |
The truth tables
More illustrative for some people may be truth tables for particular operations:
Bitwise AND (&):
a | b | a AND b |
---|---|---|
0 | 0 | 0 |
0 | 1 | 0 |
1 | 0 | 0 |
1 | 1 | 1 |
Bitwise OR (|):
a | b | a OR b |
---|---|---|
0 | 0 | 0 |
0 | 1 | 1 |
1 | 0 | 1 |
1 | 1 | 1 |
Bitwise XOR (^):
a | b | a XOR b |
---|---|---|
0 | 0 | 0 |
0 | 1 | 1 |
1 | 0 | 1 |
1 | 1 | 0 |
Bitwise NOT (^):
a | NOT a |
---|---|
0 | 1 |
1 | 0 |
Bitwise shifts
The shift operators shift their first operand left (<<) or right (>>) by the number of positions specified by the second operand.
The effect of the shift operations can be summarized briefly:
a>> N is a / 2 ^ N
a << N is a * 2 ^ N
Example — bitwise shifts:
// multiply and divide by 2: faster (bitwise) version var a = 64 >> 4; // so: 64 / 16 = 4 var b = 64 << 4; // so: 64 * 16 = 1024
And the operator a>>> b shifts a in binary representation b bits to the right, discarding bits shifted off, and shifting in zeros from the left.
Examples — other bitwise operators in JavaScript:
// bit not - reverse bits var a = 10; // 00000000000000000000000000001010 a = ~a; // 11111111111111111111111111110101 // bit and var x1 = 10, x2 = 8; var x3 = x1 & x2; // alert(x3); // bit or var x1 = 10, x2 = 8; var x3 = x1 | x2; // bit xor var x1 = 10, x2 = 8; var x3 = x1 ^ x2;
Of course there are assignment operators for bitwise operations in JavaScript:
n &= 4; // n = n & 4 n |= 4; // n = n | 4 n ^= 4; // n = n ^ 4 n <<= 4; // n = n << 4 n >>= 4; // n = n >> 4 n >>>= 4; // n = n >>> 4
Summary
We discussed essential information about the bitwise operations in JavaScript. It now remains to use them in practice. Operators we will use primarily where we want to speed up calculations.
Also, by analyzing the source code of existing scripts and applications (what I always recommend as great method of learning), we can find out their use. Especially in case of games programming, and graphical operations in general.
For the curious recommend a more extensive article at MDN.
JavaScript Yeah!