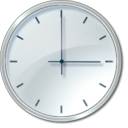
The time flies, and we will write about date and time in JavaScript. Today less theory, more practical examples.
Date and time in JavaScript. The Date object.
To handle dates in JavaScript there is an object called Date.
Basic example:
var data = new Date(); document.write(data.getDate());
The Date object — methods
Methods of the Date object:
data.getDay(); // day number 0-6 data.getFullYear(); // 1000-9999 data.getHours(); // current hours on the computer data.getMiliseconds(); // the number of milliseconds data.getMinutes(); // minutes data.getMonth(); // months (0-11) data.getSeconds(); // seconds // conversion into a readable string: data.toDateString(); data.toLocaleDateString(); data.toLocaleString(); date.toString();
Tip: use instanceof
With this operator we can check whether we are dealing with an object of some type. For example let’s check if we are operating on the Date object instance:
var my_date = new Date(); alert(my_date.getDate()); // is the 'my_date' an instance of Date? alert(my_date instanceof Date);
Setting the date
By creating a Date object, we can specify (to set) the date, and this in several ways:
var today = new Date(); var birth = new Date("March 27, 1986 05:24:00"); alert(birth); // or var birth = new Date(1986,03,27); var birth = new Date(1986,03,27,5,24,0);
As you can see, we can set only the date, or date and hour. We can also set the date through dedicated methods:
var dt = new Date(); dt.setFullYear(2012,02,12);
This code sets: year, month and day.
In contrast, here:
var dt = new Date(); dt.setDate(dt.getDate() + 10);
… we set a future date (10 days ahead).
Other examples
Example — measuring the execution time:
var start = Date.now(); // … code to measure… alert('test'); // … end: code to measure… var stop = Date.now(); var diff = stop — start; // time in milliseconds
Example — comparison of dates using the Date object:
var cmpDate = new Date(); cmpDate.setFullYear(2020,2,25); var today = new Date(); // comparing if (cmpDate > today) { alert("Future"); } else { alert("Past"); }
Simple and effective way.
Example — current time:
function getTime() { var now = new Date(); var result = now.getHours() + ":" + now.getMinutes(); return(result); } alert("It's " + getTime());
Example — building a readable date:
var tm = new Date(); var resTxt = ''; resTxt += "We have now " + tm.getHours() + ":" + tm.getMinutes() + ":" + tm.getSeconds() + ", "; resTxt += " of day: " + tm.getDate() + "." + (tm.getMonth() + 1) + "." + tm.getFullYear(); alert(resTxt);
In case of getMonth() method, we added 1 to the result. That’s because the method returns month numbers starting from 0. It’s a trait of JavaScript.
There is another issue — formatting. The instruction alert(resTxt); will display the result similar to following:
“We have now 21:47:3, of day: 16.8.2013″
Would be great to add missing ‘0’ to have the result like:
“We have now 21:47:03, of day: 16.08.2013″
Just let’s add conditions to the code that generates the final string, such as:
if (minute < 10) minute = "0" + minute;
Such code we will see in the next, a little more extensive example.
Example — our own date formatting function:
function showTime() { var monthsArr = ["Jan", "Feb", "Mar", "Apr", "May", "Jun", "Jul", "Aug", "Sep", "Oct", "Nov", "Dec"]; var daysArr = ["Sat", "Mon", "Tue", "Wed", "Thu", "Fri", "Sat"]; var dateObj = new Date(); var year = dateObj.getFullYear(); var month = dateObj.getMonth(); var numDay = dateObj.getDate(); var day = dateObj.getDay(); var hour = dateObj.getHours(); var minute = dateObj.getMinutes(); var second = dateObj.getSeconds(); if (minute < 10) minute = "0" + minute; if (second < 10) second = "0" + second; var out = daysArr[day] + ", " + numDay + " " + monthsArr[month] + " " + year + ", " + hour + ":" + minute + ":" + second; return out; } alert(showTime());
Presented code will display the result such as for example:
“Fri, 16 Aug 2013, 22:00:57”
It’s actually a complete code example, that displays the date and time in JavaScript. It also demonstrates how to operate on the values returned by the Date object’s methods.
The time and timers
The setTimeout() function
Here we briefly tell about counting down, and also how to execute the code after some time (setTimeout function):
// execute in 4 secs setTimeout(function() { alert("Foobar!") } , 4000);
It is sometimes very useful, e.g. when we want to call some code from a library, which at the moment is not yet fully loaded. Or calling a function which must wait for work results of another code parts.
Then we need to call our code with some delay, to keep everything working properly.
The setInterval() function
In addition, the program can execute code repeatedly every now and then, using the setInterval function:
var countdown = 5; var timer = setInterval( function() { countdown--; if (!countdown) { clearInterval(timer); } alert("Countdown: " + countdown); }, 2000); // how often: 2000 [ms] = 2 [s]
The function given in setInterval performs 5 times every 2 seconds (2000 ms), and interrupts the countdown afterwards.
Further examples
Example — calculation of the time difference — how much time passed between dates:
var dt_in = new Date(2007, 02, 11, 23, 59, 59); var now = new Date(); var time = now.getTime() - dt_in.getTime(); var days = Math.floor(time / (1000 * 60 * 60 * 24)); if (days > 0) { alert("Elapsed time: " + days + " days"); } else { alert("The specified date is from the future"); }
Example — calculating age in JavaScript:
Firstly — simple UI as HTML form:
<body> <form> Month: <input type="text" id="b-month" /> Day: <input type="text" id="b-day" /> Year: <input type="text" id="b-year" /> <button onclick="getAgeFromBirthday()">Calculate age</button> </form> </body>
Handling code:
function getAgeFromBirthday() { var month = document.getElementById('b-month').value; var day = document.getElementById('b-day').value; var year = document.getElementById('b-year').value; var b_date = new Date(year, month, day); if (b_date.getDate() != day || b_date.getMonth() != month || b_date.getFullYear() != year) { alert('Please enter a valid date of birth'); return false; } today = new Date(); today.setHours(0); today.setMinutes(0); today.setSeconds(0); if (b_date > today) { alert('The date must be earlier than today'); return false; } alert(Math.floor((today - b_date) / 31556952000)); }
In this example, we are getting and processing the date of birth from the user. The result is user’s age calculated from the specified data.
Date and time with JavaScript libraries
Using frameworks, we can expect that they provide us various facilities and shortcuts. This also applies to the date and time.
For example, jQuery bring us the now() method:
jQuery.now(); // no arguments, returns the current time
The $.now() method is a shortcut of the (new Date).getTime() expression.
MooTools framework gives great possibilities of operations on date and time in JavaScript, using the minimum code (methods like: date.get(), date.set(), date.clone() or date.increment()).
When we need to implement for example a real calendar, we can of course write this solution “by hand, in pure JavaScript”, or using very helpful framework.
We can use our valuable time better than on reinventing the wheel again, and use of proven, ready-made and very good solutions.
Especially I can recommend libraries such as:
– MooTools DatePicker solution
Summary
In this article we learned not only about handling date and time in JavaScript, and many ready-made solutions in the examples, but also about typical elements of object-oriented programming (OOP). And we will take a closer look at OOP in further articles.
For the curious I would recommend a more detailed description of the Date object on MDN.
And now it’s time… to finish this article.
Thank you for your time and attention.
“The $.now() method is a shortcut of the (new Date).getTime() expression.”
No, it is an alias for Date.now.
Thank you for hint.
I just got this sentence from doc http://api.jquery.com/jquery.now/
Best
Then they’re wrong too : https://github.com/jquery/jquery/blob/master/src/core.js#L455
So it was in older versions perhaps.
Thanx!
[…] https://javascript-html5-tutorial.com/date-and-time-in-javascript.html […]