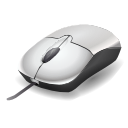
Welcome to the article, in which we will try to comprehensively but succinctly talk about working with the mouse and keyboard in JavaScript. We will also see how popular frameworks can help us.
Handling mouse and keyboard in JavaScript
Considerations we should start with events, because processing data from basic input devices will be based on event handling.
Events for the mouse in JavaScript:
– onclick — single-click (press and release the mouse button),
– ondblclick — double-click,
– onmouseover — the mouse cursor is over the element (in the area of an element),
– onmousemove — the mouse cursor has been moved,
– onmouseout — the mouse cursor is outside the element (left his area),
– onmousedown — pressing the mouse button,
– onmouseup — release the mouse button.
In addition, there are available (by object event) parameters for mouse events:
– clientX and clientY — relative coordinates of the mouse cursor,
– screenX and screenY — screen / absolute coordinates of the mouse,
– button — the number of the pressed mouse button,
– altKey — determines whether the Alt key is pressed,
– ctrlKey — determines whether the Control key is pressed,
– shiftKey — determines whether the Shift key is pressed.
The keyboard events are:
– onkeypress
– onkeydown
– onkeyup
Handling mouse in JavaScript
Having to deal with the events, the first thing comes to mind is to implement handlers for them. This applies both to mouse and keyboard.
As in the following example:
document.onmousedown = mouseDown; function mouseDown(e) { alert('Pressed …'); } // document.onmousemove = xyz; // myElement.onmouseup = foobar; // etc …
We just assigned a handler for onmousedown event of an element (here for the document element). This is how it’s done.
Getting coordinates of the mouse cursor is done by getting the relevant parameters of an Event object:
document.onclick = function(e) { e = e || window.event; var pX = 0; var pY = 0; if (e.pageX || e.pageY) { pX = e.pageX; pY = e.pageY; } else if (e.clientX || e.clientY) { pX = e.clientX + document.body.scrollLeft; pY = e.clientY + document.body.scrollTop; } // console.log([pX, pY]); alert(pX); alert(pY); };
This is a simple case, where handler is assigned to the click event. The above code returns the coordinates of where you clicked the mouse.
Of course, we can also do the assignment in the HTML. For example, define a function that will be called when we move the mouse on the item:
<div style="border: solid 1px red; width: 400px; height: 250px" onmouseover="myFunc()" />
When this occurs, the myFunc() function will be called.
Determining the mouse button that was pressed, is allowed by the parameter “button” of event object.
By default, numbers are as follows: 0 for left mouse button, 1 for middle (center button) and 2 for the right button.
The following is a simple example of use, gives the number of the clicked mouse button:
document.onclick = function(e) { e = e || window.event; alert(e.button); // checking the right mouse button if (e.button == 2) alert('The right'); };
Mouse in JavaScript vs frameworks
For sure we can find great solutions to support our work with mouse in JavaScript, and more importantly giving the results of cross-browser.
Personally, I am in this case a big fan of jQuery, which offers great possibilities and is easy to use.
I recommend list of events supported by jQuery. On every page you will find very good examples of the use.
If someone prefers Dojo Toolkit‘s approach, also will not be disappointed, thanks to the dojo/mouse module.
Mouse middle button
Javascript and its libraries also supports this button and the scroll of course.
The simplest case is the onscroll event handler of the document. And by the way, this applies to scroll at all (e.g. use the scroll bar), and not only the use of middle mouse button.
Example:
document.onscroll = function(e) { alert('Scrolling!'); };
In case of handling scrolling, I recommend the opportunities offered by MooTools library — MouseWheel. On that page you will find a very neat example of using MooTools to handle the scroll key.
Let’s don’t forget about jQuery. In projects we have sometimes used the jquery.mousewheel plugin. Works well if you want, for example, implement your own scroll-bar to scroll the content in own UI element.
Keyboard in JavaScript
Keyboard handling is done also based on events:
element.onkeydown = func1;
element.onkeypress = func2;
element.onkeyup = func3;
A small example. Let’s define an event listener for the keydown event:
window.addEventListener('keydown', function(event) { var key = event.keyCode; alert(key); alert(String.fromCharCode(key)); }, false);
The “key” variable contains the ASCII character code, and after processing (String.fromCharCode(key)) we obtain the correct character.
It is easiest to operate on character codes — by comparison. We can use the table of codes, such as available here, or in this article, which says also about keyboard shortcuts.
As a more interesting example: the code below is a skeleton of arrows handling (left, right, top, down) arrows of the keyboard. Typically, may be used to move the screen element, even in the context of a game programming.
window.addEventListener('keydown', function(event) { switch (event.keyCode) { case 37: // Left alert('Turn left'); break; case 38: // Up alert('Top'); break; case 39: // Right alert('Turn right'); break; case 40: // Down alert('Bottom'); break; } }, false);
Simple and working code in pure JavaScript.
So let’s check what is offered by JS frameworks. Handling keyboards in jQuery is painless:
$(document).keydown(function(event) { alert('You pressed ' + event.keyCode); event.preventDefault(); });
In this way we have served the onkeydown event. Calling the event.preventDefault() method prevents the default action, which takes place after the event.
The list of events supported by jQuery and examples available here.
Dojo Toolkit also offers us his interesting approach to handle keyboard.
Summary
In conclusion, here are some links to sites with similar content and interesting libraries.
Links:
– FAQ about mouse and keyboard events
http://www.javascripter.net/faq/keyboardmouseevents.htm
– detailed article of the keyboard from Mozilla
https://developer.mozilla.org/en-US/docs/Web/API/KeyboardEvent
– a tutorial about creating own, virtual keyboard using jQuery and CSS
http://net.tutsplus.com/tutorials/javascript-ajax/creating-a-keyboard-with-css-and-jquery/
Other libraries:
– keymaster — a simple library, that allows to define keyboard shortcuts for web application
https://github.com/madrobby/keymaster
– Keypress library
http://dmauro.github.io/Keypress/
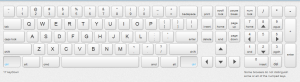
Keypress library
It’s some kind of virtual keyboard, and allows us to capture the user’s keyboard input. From the programmer’s point of view, is certainly worth a look for its source code.
Thank you. Press any key…
Something cool? -> http://www.webresourcesource.com/jquery-ui-virtual-keyboard/
[…] https://javascript-html5-tutorial.com/mouse-and-keyboard-in-javascript.html […]